Write a Java Program to Check Prime Number using For Loop, While Loop, and Functions. Prime Numbers are any natural number not divisible by any other number except 1 and itself.
And they are: 2, 3, 5,7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179, 181, 191, 193, 197, 199, etc.
Java Program to Check Prime Number using For Loop
This program allows the user to enter any integer value. Next, this Java example program checks whether the given number is a Prime or Not using For Loop.
TIP: 2 is the only even.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { int i, number, count = 0; sc = new Scanner(System.in); System.out.print(" Please Enter any Integer : "); number = sc.nextInt(); for (i = 2; i <= number/2; i++) { if(number % i == 0) { count++; break; } } if(count == 0 && number != 1 ) { System.out.println( number + " is a Prime"); } else { System.out.println(number + " is Not"); } } }
Using the for loop output.
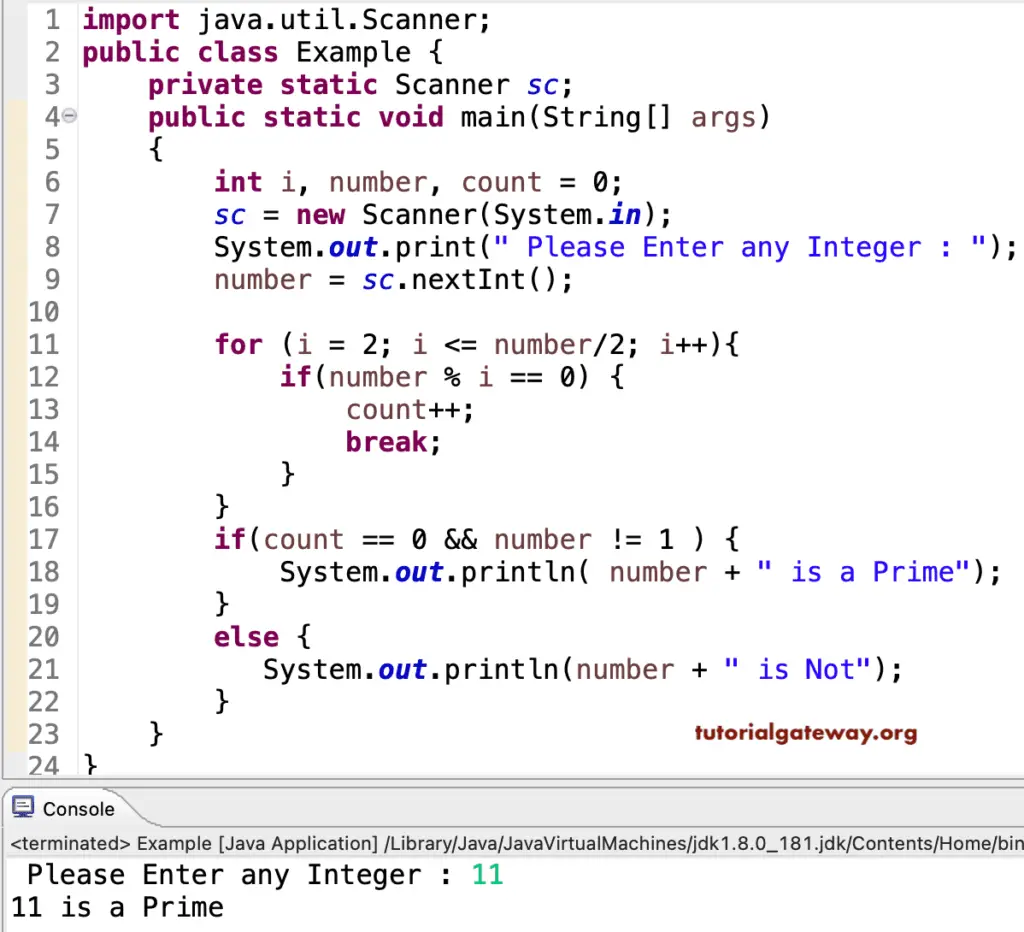
We initialized the integer i value to 2, and (i <= Number/2) condition to terminate when the condition fails.
Within the for loop, there is an If statement to check whether the Number divisible by i is exactly equal to 0 or not. If the condition is True, the Count is incremented, and the Break Statement is executed.
Next, we used another If statement to check whether the Count is Zero and the Number is Not equal to 1. Suppose it is True, then prime.
The user entered an integer in the above Java Program to Check the Prime Number example is 11.
First Iteration: for(i = 2; i <= 11/2; i++)
- Condition (2 <= 5.5) is True. So, it checks the If Statement.
- if(number % i == 0) => if (11%2 == 0) – Condition is False
- i++ means i becomes 3
Second Iteration: (i = 3; 3 <= 11/2; i++)
- Condition (3 <= 5.5) is True.
- if (11 % 3 == 0) – Condition is False,
- i become 4.
Third Iteration: (i = 4; 4 <= 11/2; i++)
- Condition (4 <= 5.5) is True.
- if (11 % 4 == 0) – Condition is False.
- i become 5
Fourth Iteration: (i = 5; 5 <= 11/2; i++)
- Condition (5 <= 5.5) is True.
- if (11 % 5 == 0) – Condition is False.
- i become 6
Fifth Iteration: (i = 6; 6 <= 11/2; i++). It means the condition inside the For loop (6 <= 5.5) is False. So, the compiler comes out of the For Loop.
Next, it will enter into the If statement. if(count == 0 && Number!= 1 ).
In all five iterations, the Java If statement failed, so Count Value has not incremented from initialized 0, and Number= 11. So, the condition is True, and the number is Prime.
Java Program to Check Prime Number using While Loop
This example checks whether the given number is a Prime or not using While Loop.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { int i = 2, num, count = 0; sc = new Scanner(System.in); System.out.print(" Please Enter any Value : "); num = sc.nextInt(); while(i <= num/2) { if(num % i == 0) { count++; break; } i++; } if(count == 0 && num != 1 ) { System.out.println( num + " is a Prime"); } else { System.out.println(num + " is Not"); } } }
We just replaced the For loop in the above example with the While loop. If you don’t know the Loop, please refer to WHILE LOOP.
output
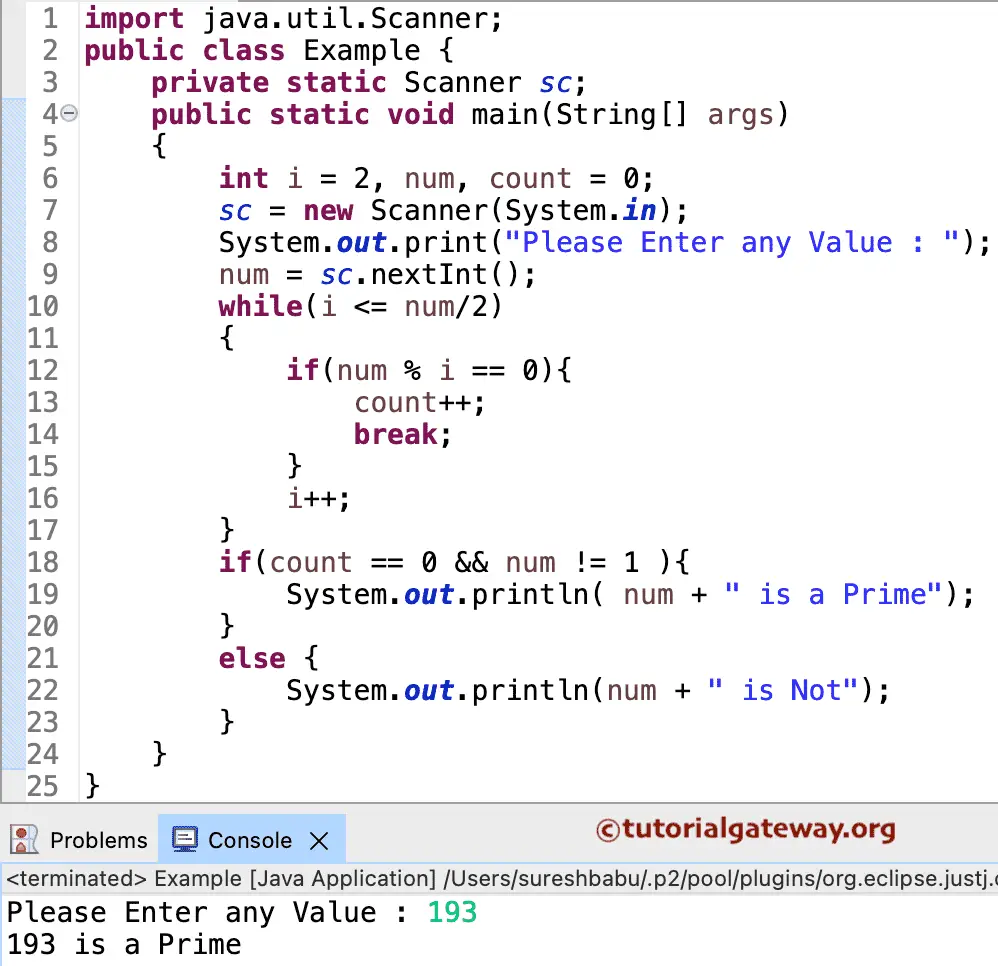
Let me enter another
Please Enter any Value : 32
32 is Not
Java Program to Check Prime Number Using Function
This program to find the prime number is the same as the first example. However, we separated the logic and placed it in a separate method.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { int num, cnt = 0; sc = new Scanner(System.in); System.out.print(" Please Enter any : "); num = sc.nextInt(); cnt = PriNu(num); if(cnt == 0 && num != 1 ) { System.out.println( num + " is a Prime"); } else { System.out.println(num + " is Not"); } } public static int PriNu(int num) { int i, cnt = 0; for (i = 2; i <= num/2; i++) { if(num % i == 0) { cnt++; break; } } return cnt; } }
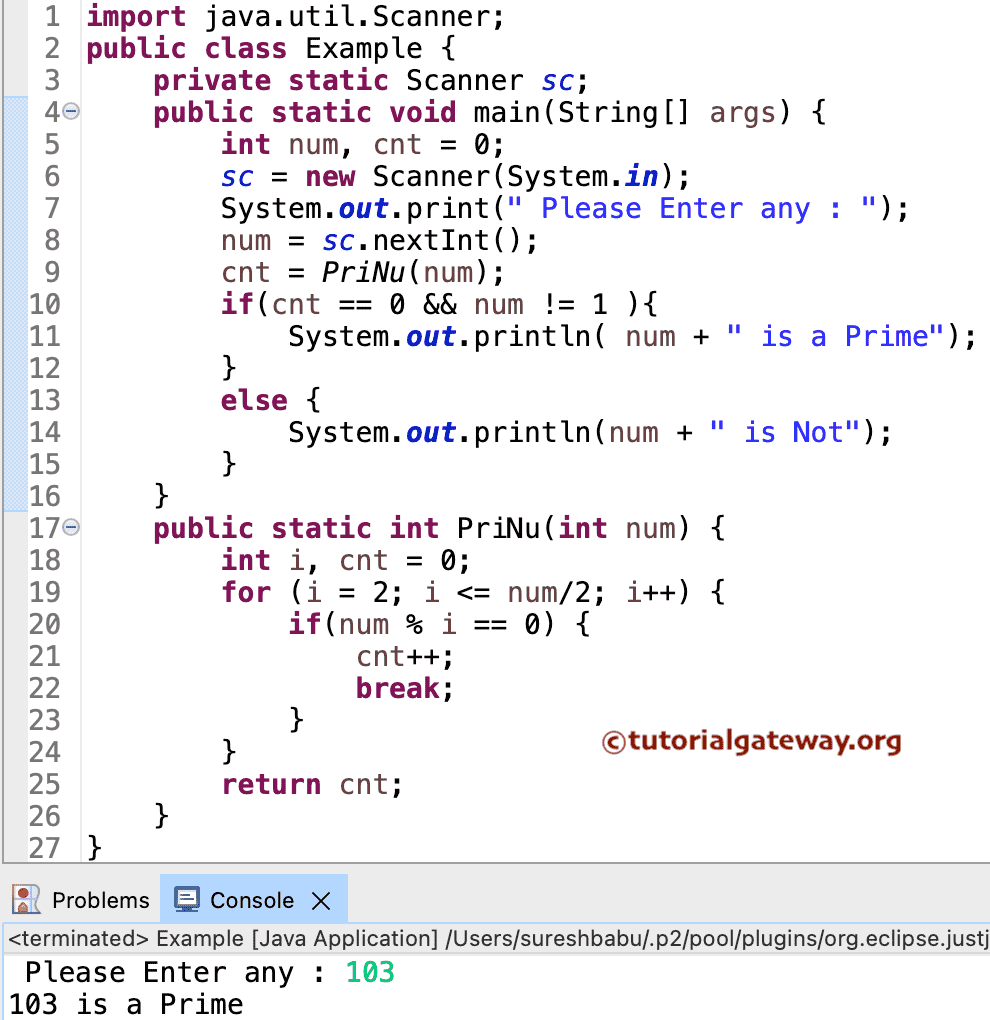
Output 2
Please Enter any : 242
242 is Not