The Java If Statement is one of the most useful decision-making codes in real-world programming. The Java if statement allows the compiler to test the condition first, and depending on the result, it will execute the code block. Only the code within this will run when the test condition is true.
Java If Statement Syntax
If statement in Java Programming language has a simple structure that returns true or false:
if (test condition) { Statement1; Statement2; Statement3; …………. …………. StatementN; }
From the above Java If Statement code snippet, When the test condition is true, then Statement1, Statement2, Statement3, ……., StatementN will be executed. Otherwise, all of them inside the if statement will skip. Let us see the flow chart for a better understanding.
Flow Chart
The following picture will show the flow chart behind this Java If Statement.
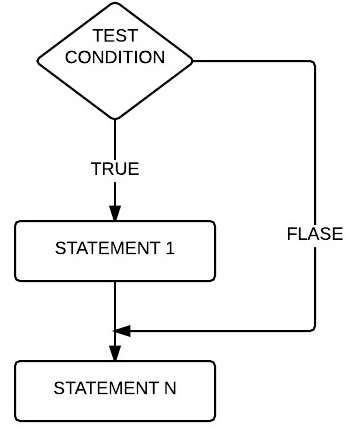
When the test condition is true, then STATEMENT1 is executed, followed by STATEMENTN. While the expression evaluates to False, then the STATEMENTN will run. Because it is out of the conditional block and it has nothing to do with the condition result, let us see one example for a better understanding.
Java If Statement Example
This if statement program allows the user to enter any positive integer, and it will check whether a number is Positive or Not.
package ConditionalStatements; import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { int Number; sc = new Scanner(System.in); System.out.println("Please Enter any integer Value: "); Number = sc.nextInt(); if (Number > 1) { System.out.println("\nYou have entered POSITIVE Number"); } } }
You can observe that we entered 25 as a Number, and this program will check whether 25 is greater than 1. As we all know that it is True, it is printing (System.out.println function) inside the curly brackets ({}}.
Please Enter any integer Value:
25
You have entered POSITIVE Number
Java If statements do not require the curly brackets to hold a single line, it is mandatory for multiple or groups of code lines. It is always good practice to use curly brackets following these conditional blocks. Let us change the value to check what happens when the condition fails. (number < 1).
Please Enter any integer Value:
-5
It prints nothing because we have nothing to print after the expression block. I guess you are confused about the result. Let us see one more example.
Java If Statement Example 2
This conditional program allows you to enter any positive integer, which will check whether a number is Positive or Not.
import java.util.Scanner; public class Sample { private static Scanner sc; public static void main(String[] args) { int Number; sc = new Scanner(System.in); System.out.println(" Please Enter any integer Value: "); Number = sc.nextInt(); if (Number > 1) { System.out.println("You have entered POSITIVE Number"); } System.out.println("This Message is coming from Outside"); } }
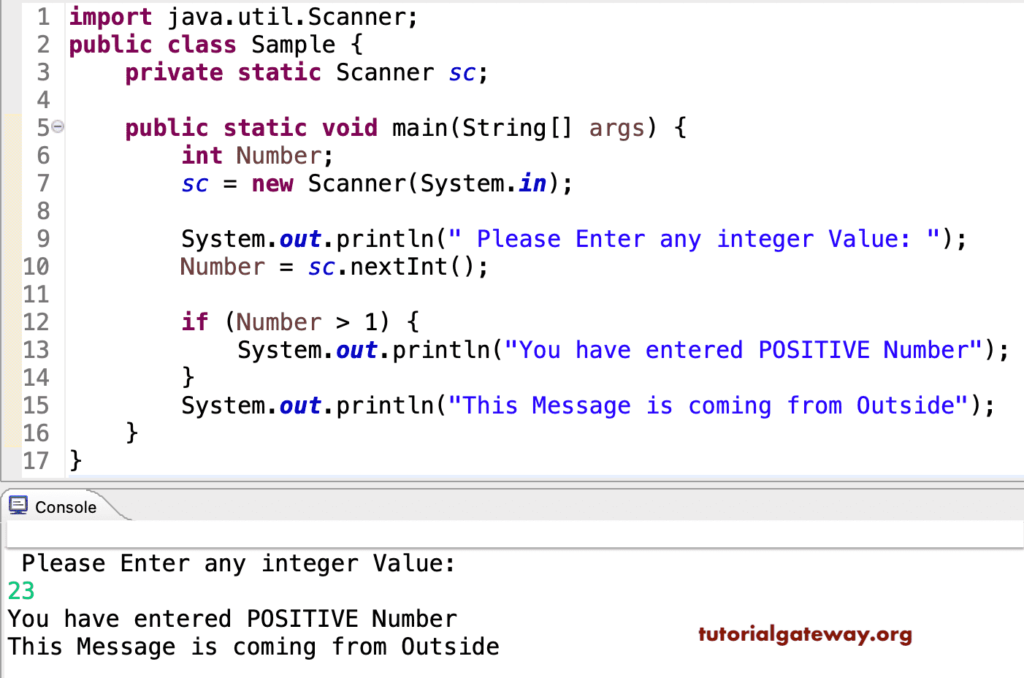
As you can observe from the above output, Javac printed both the System.out.println functions because 23 is greater than 1. Let us try the negative values to fail the condition deliberately.
Please Enter any integer Value:
-50
This Message is coming from Outside
Here, the Condition failed (number < 1). It prints nothing from the If statement block, so it printed only one System.out.println function outside the block.
Comments are closed.