The If statement in C programming is one of the most useful decision-making expressions in real-time programming. The C If condition allows the compiler to test the condition first, and then, depending upon the result, it will execute the statements. Whether the test condition is true, then only statements within the if condition is performed by the compiler.
If Statement in C syntax
The basic syntax of the If statement in C programming has a simple structure:
if (test condition) { Statement1; Statement2; Statement3; …………. …………. Statementn; }
From the above code, whether the test condition in the If clause is true, the code block (Statement1, Statement2, Statement3, ……., Statementn) will be executed. Otherwise, all these lines will skip.
If statement in C Example
This example program checks for the positive number using the if statement.
For the single printf function, curly brackets are not required in C Programming. But for multiple lines of code, it is mandatory.
#include <stdio.h> int main() { int number; printf("Enter any integer Value\n"); scanf("%d",&number); if( number >= 1 ) { printf("You Have Entered Positive Integer\n"); } return 0; }
It is always good practice to use curly brackets following the C If statement.
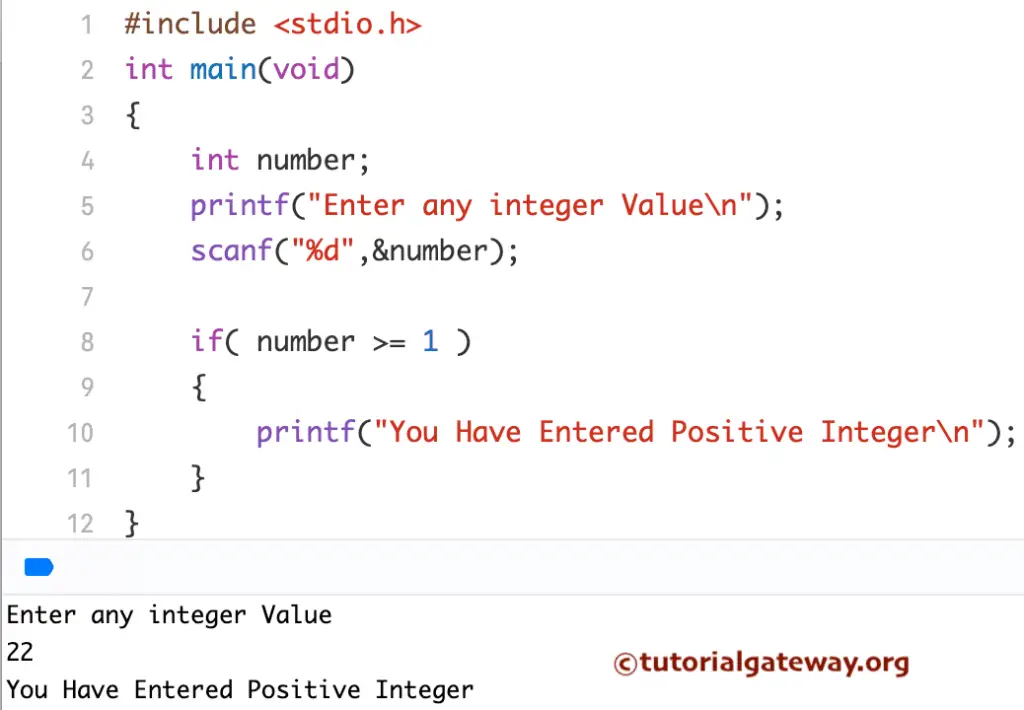
When you look at the above if condition, the Value stored in the number variable is greater than or equal to 0. That’s why it printed (print function) inside the curly brackets ({}}.
From the above example, what happens when the condition fails? (number < 1).
Enter any integer Value
-22
Program ended with exit code: 0
It prints nothing because we don’t have anything to print after the if block. I hope you are confused with the result, so let us see the flow chart.
If Condition Flow Chart
The flow chart of an If statement in C programming is as shown below:
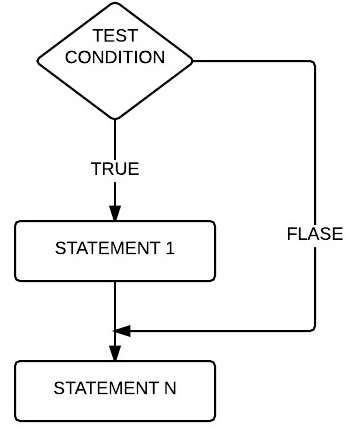
Whether the test condition is true, STATEMENT1 is executed, followed by STATEMENTN. When the condition is False, STATEMENTN executes because it is out of the if block. It has nothing to do with the condition result.
#include <stdio.h> int main() { int number; printf("Enter any integer Value\n"); scanf("%d",&number); if( number >= 1 ) { printf("You Have Entered Positive Integer\n"); } printf("This Message is not coming from IF STATEMENT\n"); return 0; }

You can observe from the above output it printed both the printf functions because 22 is greater than 1. Let’s try the negative values to fail the condition deliberately.
Enter any integer Value
-13
This Message is not coming from IF STATEMENT
If condition (number < 1) fails here, it prints nothing from the C If statement block. So, it wrote only one printf, which is outside of the block.