The goto statement in C Programming is useful to alter the flow of a program. When the C compiler reaches the goto statement, then it will jump unconditionally ( both forward and backward ) to the location specified in it (we called it as a label).
Unlike the Break and Continue, the goto statement in C Programming doesn’t require any If condition to perform.
C Goto Statement Syntax
The label specified after the goto statement in C is the location where we place the code block to execute. From the below syntax, you can understand that we can place the label anywhere in the program. It doesn’t matter if you put it before the goto or after.
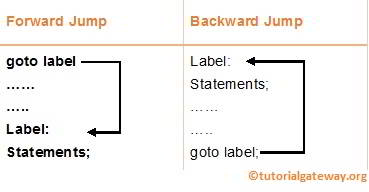
Goto Statement in C Example
This program for goto allows the user to enter his/her individual subject marks. Next, it will check whether the person passes or fail using Goto Statement.
#include <stdio.h> int main() { int Totalmarks; printf(" \n Please Enter your Subject Marks \n "); scanf("%d", & Totalmarks); if(Totalmarks >= 50) { goto Pass; } else goto Fail; Pass: printf(" \n Congratulation! You made it\n"); Fail: printf(" \n Better Luck Next Time\n"); return 0; }
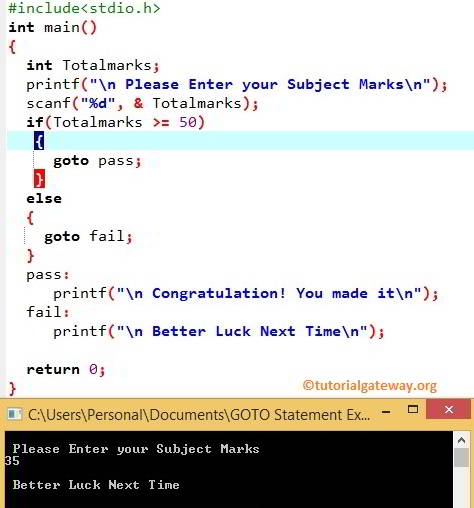
In the above program, Pass and Fail are the labels we used. First, we declared the integer variable Totalmarks.
In the next line, the Printf function will ask the user to enter his/her total marks. The scanf function will store the user-specified value in the Totalmarks variable.
Please refer to Break, Continue, and If condition articles in C Programming.
In the next line, we used If Else to check whether the user entered value is greater than or equal to 50.
If the condition is TRUE, the C Goto statement inside the If block will take the compiler to the Pass label and execute the block of code inside the Pass label.
printf(" \n Congratulation! You made it\n");
Else (If the condition is FALSE), the code inside the Else block will take the compiler to the Fail label. Next, it executes the code block inside the Fail label.
printf(" \n Better Luck Next Time\n");
NOTE: Although all the programming languages support goto statements. However, it is always good practice to avoid using it or at least minimize its usage. Instead of using this, we can use alternatives such as Break and Continue.