Pointers in C programming are the most powerful concept because pointer variables contain or hold the address of another variable. C Pointers are beneficial to hold the address of the variable. Using the address, we can access the value inside the associated variable. Or we can manipulate the value stored in that address. So, any changes made in the pointer will reflect the original value.
In the computer world, the System will allocate the memory (in Bytes) as per the requirement for each variable we store. It means Every variable has value and memory. The Pointers in c example,
int x = 10; int *p;
x is the variable name.
10 is the value associated with or assigned to the x.
10 stored in some memory location. Say 1042
So, Pointer *p will assign to 1042 and access the value inside the x, i.e., 10.
C Pointers Syntax
The Syntax of the Pointers in the C programing language is shown below.
Data_Type *Pointer_Name;
Pointer_Name: Name of it.
(*): It tells that the variable is a pointer.
Data Type: The C pointers variable contains the address of others. So, there is no use in declaring its type. The Data Type in the syntax does not belong to the pointer. It belongs to the variable that is associated with the pointer. For instance,
int *x;
It means the pointer variable x is assigned to the integer only. If you assign the pointer x to float or character data type, then it will give a strange result. Say
int a; float b; x = &a; // Correct because we are assigning integer variable x = &b; //Wrong because we are assigning float variable
As we all know, &a is the address of the a, and x is the addition of the *x.
x = &a; means we are actually assigning the address of the a to x.
To access the value inside the C pointers variable, we can simply use *x.
int *x; *x = 20; printf("%d",*x)
To access the address of the pointers.
printf("%d", x)
C Pointers Example
This program allows the user to enter any positive integer. Using the address of the variable, access the user-entered value. Next, using the C pointers variable, change the user-entered value.
#include <stdio.h> int main() { int *P,Number; printf("\n Please Enter any Positive Integer"); scanf("%d", &Number); printf("\n Value Inside the Number = %d \n", Number); printf(" Address of the Number = %d\n",&Number); P = &Number; printf("\n Value Inside the Pointer P = %d \n", *P); printf(" Address of the Pointer P = %d\n", P); *P = 20; printf("\n Value Inside the Pointer P = %d \n", *P); printf(" Address of the Pointer P = %d\n", P); printf(" Value Inside the Number = %d \n", Number); printf(" Address of the Number = %d\n",&Number); return 0; }
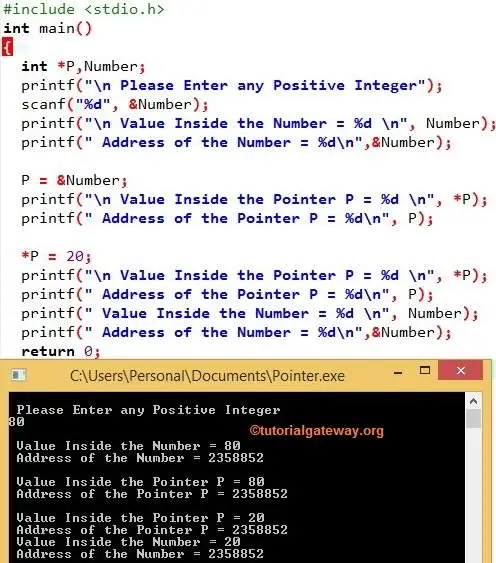
In this C example, we declared one integer Number and one pointer P of type integer.
The printf statement asks the user to Enter any Positive Integer. And the scanf statement assigns the user entered value to the Number we already declared.
The first statement will print the value inside the Number (User Entered Value), and the next one prints the declared number’s address.
In the Next line, We assigned the address of the Number to the address of the C pointers. Here, P is the address of the pointer that we already declared (*P), and &Number is the address of the Number.
P = &Number;
It prints the value inside the P. Here is the address of the P assigned to the address of the Number So, We are actually printing the User Entered Value, i.e., Number
printf("\n Value Inside the Pointer P = %d \n", *P);
Prints the Address of the Pointer, Which is obviously the same as the address of the Number
printf(" Address of the Pointer P = %d\n", P);
In the Next line, We are assigning 20 to the *P
*P = 20;
It means,
- Value inside the *P = 20
- From the above (P = &Number), The address of the pointers variable and Number var in C programming are the same. It means we are not only changing the value of *p, But We are also actually changing the data inside the Number variable (Number)
- It means the User entered value inside the Number will be changed to 20.
The last four printf statements will print the value and address of the Number and Pointer variables.