Write a Java Program to Sort Array in Descending Order without using the Built-in function (temp variable) and using the Sort method with an example.
Java Program to Sort Array in Descending Order
In this program, we are using the Collections.reverseOrder() and Array.sort method to sort the array elements in descending order.
import java.util.Arrays; import java.util.Collections; import java.util.Scanner; import java.util.Comparator; public class Example1 { private static Scanner sc; public static void main(String[] args) { int Size, i; sc = new Scanner(System.in); System.out.print(" Please Enter Number of elements : "); Size = sc.nextInt(); Object [] a = new Object[Size]; System.out.print(" Please Enter " + Size + " elements : "); for (i = 0; i < Size; i++) { a[i] = sc.nextInt(); } Comparator<Object> cms = Collections.reverseOrder(); Arrays.sort(a, cms); System.out.println("\n Result : "); for (Object Number: a) { System.out.print(Number + " "); } } }
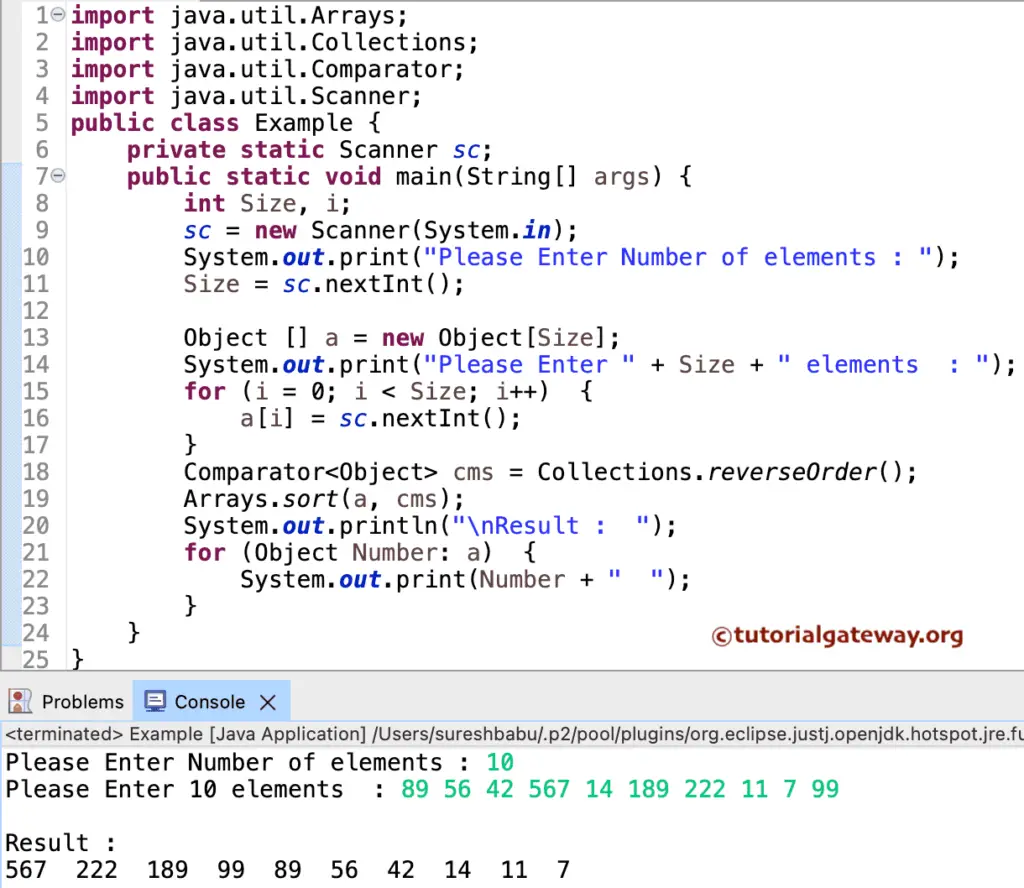
Please refer to the sort article.
Java Program to Sort Array in Descending Order using Temp Variable
This Java program allows the user to enter the size and Array of elements. Next, it will sort the array element in descending order using For Loop.
import java.util.Scanner; public class SortDescending2 { private static Scanner sc; public static void main(String[] args) { int Size, i, j, Temp; sc = new Scanner(System.in); System.out.print(" Please Enter Number of elements in an array : "); Size = sc.nextInt(); int [] a = new int[Size]; System.out.print(" Please Enter " + Size + " elements of an Array : "); for (i = 0; i < Size; i++) { a[i] = sc.nextInt(); } for (i = 0; i < Size; i++) { for (j = i + 1; j < Size; j++) { if(a[i] < a[j]) { Temp = a[i]; a[i] = a[j]; a[j] = Temp; } } } System.out.print("\n Result of a Descending Array : "); for (i = 0; i < Size; i++) { System.out.print(a[i] + " "); } } }
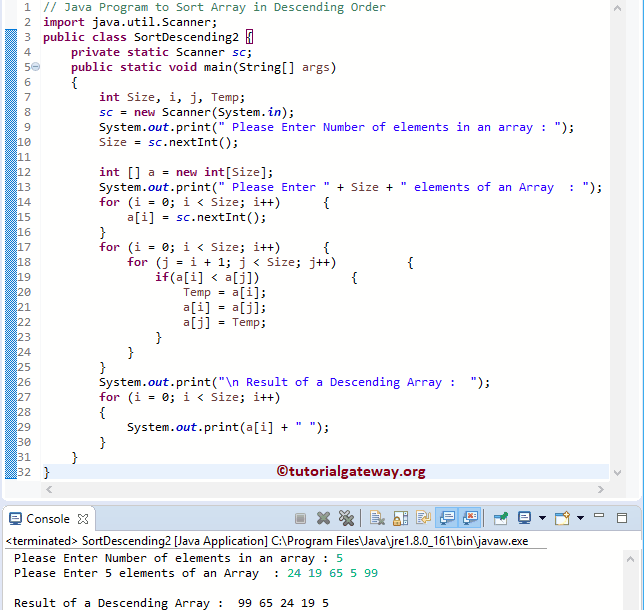
User inserted values in this Java program are a[5] = {24, 19, 65, 5, 99}
First For Loop – First Iteration: for (i = 0; 0 < 5; 0++)
The value of i will be 0, and the condition (i < 5) is True. So, it will enter into the inner or Nested For Loop.
Second For Loop – First Iteration: for (j = 0 + 1; 1 < 5; 1++). The condition (1 < 5) is True. So, it will enter into the If Statement.
if(a[i] < a[j]) => if(24 < 19) – Condition is False
Second For Loop – Second Iteration: for (j = 2; 2 < 5; 2++). Here, the condition (i < 5) is True.
if(a[i] < a[j]) => if(24 < 65) – Condition is True
Temp = a[0] = 24
a[0] = a[2] = 65
a[j] = Temp => a[2] = 24
Do the same for the remaining iterations until the condition (i < 5) fails.
The next for loop is to print the elements. I suggest you refer to the Java Program to Print Array Elements article to understand the same.
Java Program to Sort Array in Descending Order using Functions
This program is the same as above. Still, this time we separated the logic to sort elements of an array in descending order using the Method. And we used one more function to print the items in it.
import java.util.Scanner; public class Example3 { private static Scanner sc; public static void main(String[] args) { int Size, i; sc = new Scanner(System.in); System.out.print(" Please Enter Number of elements : "); Size = sc.nextInt(); int [] a = new int[Size]; System.out.print(" Please Enter " + Size + " elements : "); for (i = 0; i < Size; i++) { a[i] = sc.nextInt(); } OrderDesc(a, Size); System.out.print("\n Result : "); printAttay(a, Size); } public static void OrderDesc(int[] a, int Size) { int i, j, Temp; for (i = 0; i < Size; i++) { for (j = i + 1; j < Size; j++) { if(a[i] < a[j]) { Temp = a[i]; a[i] = a[j]; a[j] = Temp; } } } } public static void printAttay(int[] A, int Size) { int i; for (i = 0; i < Size; i++) { System.out.print(A[i] + " "); } } }
Please Enter Number of elements : 8
Please Enter 8 elements : 25 95 86 15 88 77 5 126
Result : 126 95 88 86 77 25 15 5