Write a Java Program to Sort Array in Ascending Order without using the Built-in function and using the Sort() method with an example.
Java Program to Sort Array in Ascending Order
In this program, we are using the Array sort method to sort the elements in ascending order.
import java.util.Arrays; import java.util.Scanner; public class OrderAsc1 { private static Scanner sc; public static void main(String[] args) { int Size, i; sc = new Scanner(System.in); System.out.print(" Please Enter Number of elements in an array : "); Size = sc.nextInt(); int [] a = new int[Size]; System.out.print(" Please Enter " + Size + " elements of an Array : "); for (i = 0; i < Size; i++) { a[i] = sc.nextInt(); } Arrays.sort(a); System.out.println("\n Result : "); for (int Number: a) { System.out.print(Number + " "); } } }
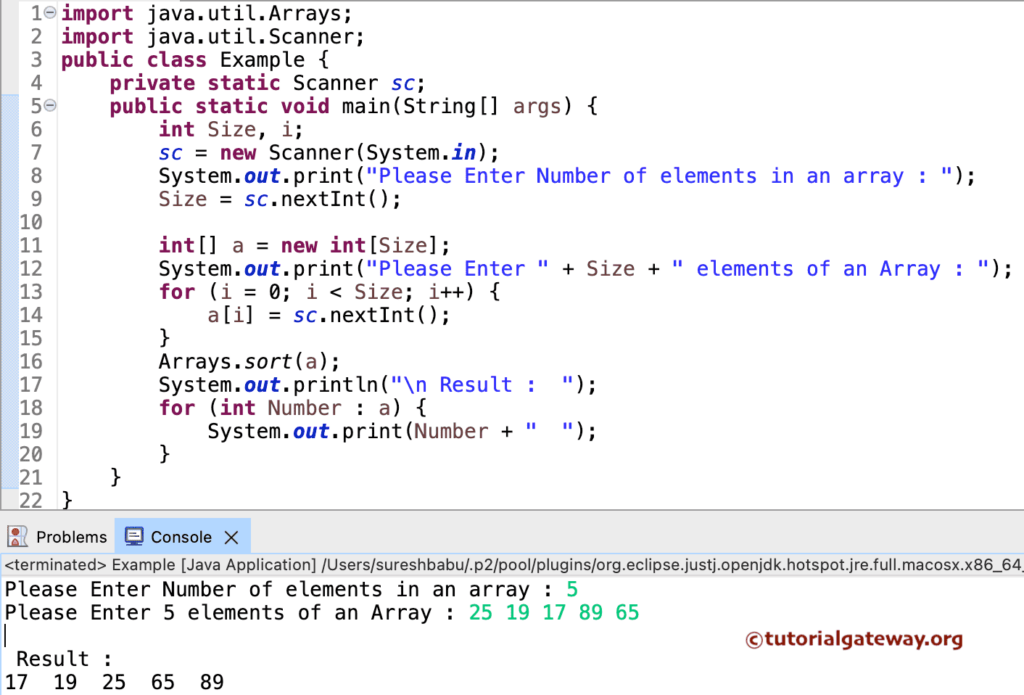
Java Program to Sort Array in Ascending Order using Temp Variable
This Java program allows the user to enter the size and the One Dimensional Array elements. Next, it will sort the array element in ascending order using For Loop.
import java.util.Scanner; public class SortAscending2 { private static Scanner sc; public static void main(String[] args) { int Size, i, j, Temp; sc = new Scanner(System.in); System.out.print(" Please Enter Number of elements in an array : "); Size = sc.nextInt(); int [] a = new int[Size]; System.out.print(" Please Enter " + Size + " elements of an Array : "); for (i = 0; i < Size; i++) { a[i] = sc.nextInt(); } for (i = 0; i < Size; i++) { for (j = i + 1; j < Size; j++) { if(a[i] > a[j]) { Temp = a[i]; a[i] = a[j]; a[j] = Temp; } } } System.out.print("\n Result Array after Ascending Order : "); for (i = 0; i < Size; i++) { System.out.print(a[i] + " "); } } }
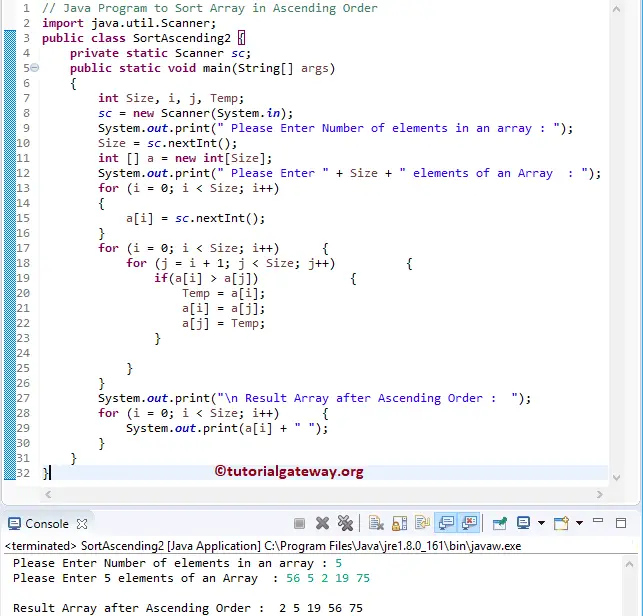
User inserted values are a[5] = {56, 5, 2, 19, 75}
First For Loop – First Iteration: for (i = 0; 0 < 5; 0++)
The value of i will be 0, and the condition (i < 5) is True. So, it will enter into the inner or Java Nested For Loop.
Second For Loop – First Iteration: for (j = 0 + 1; 1 < 5; 1++)
The condition (1 < 5) is True. So, it will enter into If Statement.
if(a[i] > a[j]) => if(56 > 5) – Condition is True in this Sort Array in Ascending Order example.
Temp = a[i] = a[0]
Temp = 56
a[i] = a[j]. It means a[0] = a[1] = 5
a[j] = Temp
a[1] = 56
Second For Loop – Second Iteration: for (j = 2; 2 < 5; 2++)
The condition (i < 5) is True.
if(a[i] > a[j]) => if(5 > 2) – Condition is True
Temp = a[0] = 5
a[0] = a[2] = 2
a[j] = Temp => a[2] = 5
Do the same for the remaining iterations until the condition (i < 5) fails.
Next for loop is to print the elements after sorting. I suggest you refer to the Java Program to Print Array Elements article to understand the same
Java Program to Sort Array in Ascending Order using Functions
This program is the same as above. But, this time, we separated the logic to sort array elements in ascending order using Method.
import java.util.Scanner; public class OrderAsc3 { private static Scanner sc; public static void main(String[] args) { int Size, i; sc = new Scanner(System.in); System.out.print(" Please Enter Number of elements : "); Size = sc.nextInt(); int [] a = new int[Size]; System.out.print(" Please Enter " + Size + " elements : "); for (i = 0; i < Size; i++) { a[i] = sc.nextInt(); } AscOrdering(a, Size); System.out.print("\n Result : "); printAttay(a, Size); } public static void AscOrdering(int[] a, int Size) { int i, j, Temp; for (i = 0; i < Size; i++) { for (j = i + 1; j < Size; j++) { if(a[i] > a[j]) { Temp = a[i]; a[i] = a[j]; a[j] = Temp; } } } } public static void printAttay(int[] A, int S) { int i; for (i = 0; i < S; i++) { System.out.print(A[i] + " "); } } }
Please Enter Number of elements : 10
Please Enter 10 elements : 89 56 42 25 365 14 198 241 12 5
Result : 5 12 14 25 42 56 89 198 241 365