How to write a C program to Reverse a String without using the strrev function with an example? To demonstrate this, we are going to use For Loop, While Loop, Functions, and Pointers.
C program to Reverse a String using for loop
This program allows the user to enter any character array. Next, it will use the For Loop to iterate each character and save the characters in inverse order.
#include <stdio.h> #include <string.h> int main() { char Str[100], RevStr[100]; int i, j, len; printf("\n Please Enter any Text : "); gets(Str); j = 0; len = strlen(Str); for (i = len - 1; i >= 0; i--) { RevStr[j++] = Str[i]; } RevStr[i] = '\0'; printf("\n And the Result of It is = %s", RevStr); return 0; }
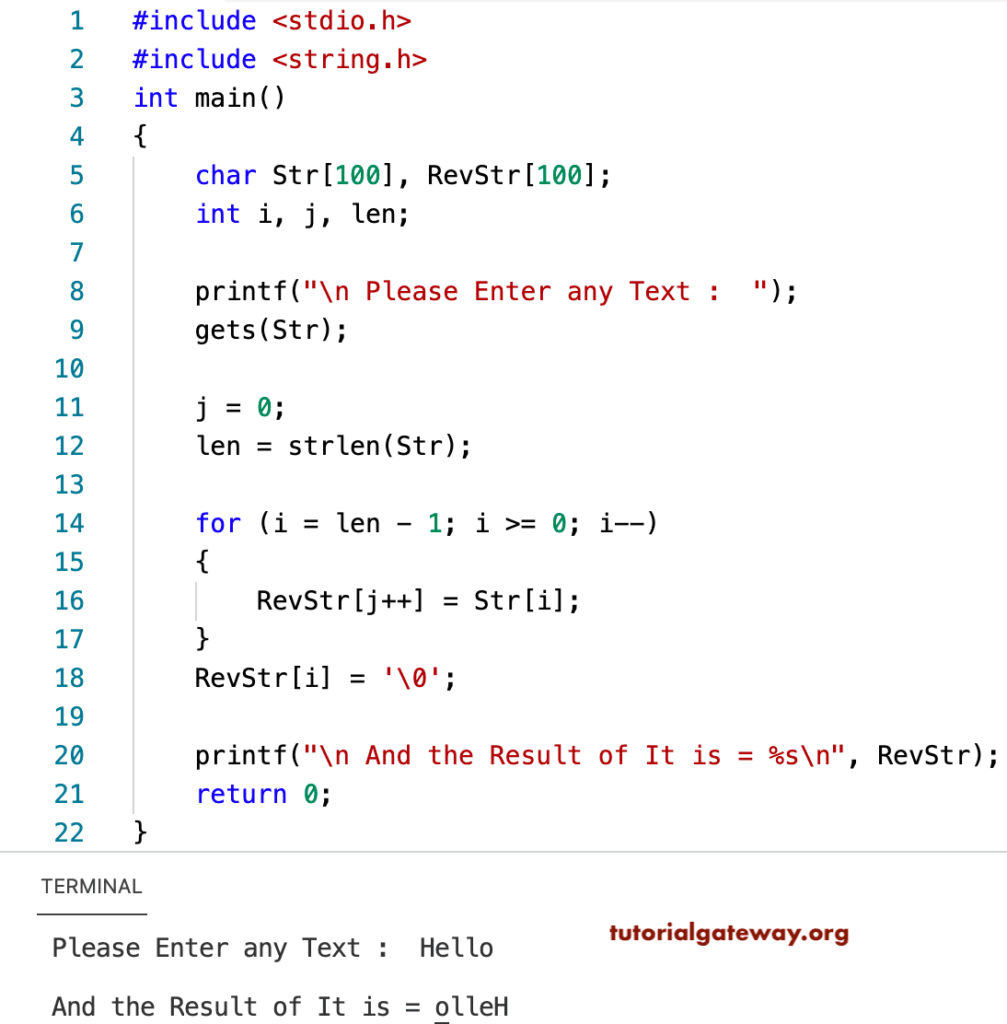
From the above C Programming screenshot, you can observe that,
str[] = Hello
j = 0
len = strlen(Str) = 5.
The strlen function is used to find the length of the input string. Let’s use that length in the programming flow. Please refer to the strrev function.
For Loop First Iteration: for (i = len – 1; i >= 0; i–)
=> for (i = 5 – 1; 4 >= 0; 4–)
The condition (4 >= 0) is True.
RevStr[j++] = Str[i]
RevStr[0] = Str[4] = 0
Second Iteration: for (i = 4 – 1; 3 >= 0; 3–)
The condition (3 >= 0) is True.
RevStr[1] = Str[3] = l
Third Iteration of a C program to Reverse a String: for (i = 3 – 1; 2 >= 0; 2–)
The condition (2 >= 0) is True.
RevStr[2] = Str[2] = l
Fourth Iteration: for (i = 2 – 1; 1 >= 0; 1–)
The condition (1 >= 0) is True.
RevStr[3] = Str[1] = e
Fifth Iteration: for (i = 1 – 1; 0 >= 0; 0–)
The condition (0 >= 0) is True.
RevStr[4] = Str[0] = H
Next, the condition inside the For Loop will fail.
String reverse Example 2
Instead of storing the results in a separate array, we directly printed the string characters in reverse order in this program.
#include <stdio.h> #include <string.h> int main() { char Str[100]; int i, ln; printf("\n Before : "); gets(Str); ln = strlen(Str); printf("\n After : "); for (i = ln - 1; i >= 0; i--) { printf("%c", Str[i]); } return 0; }
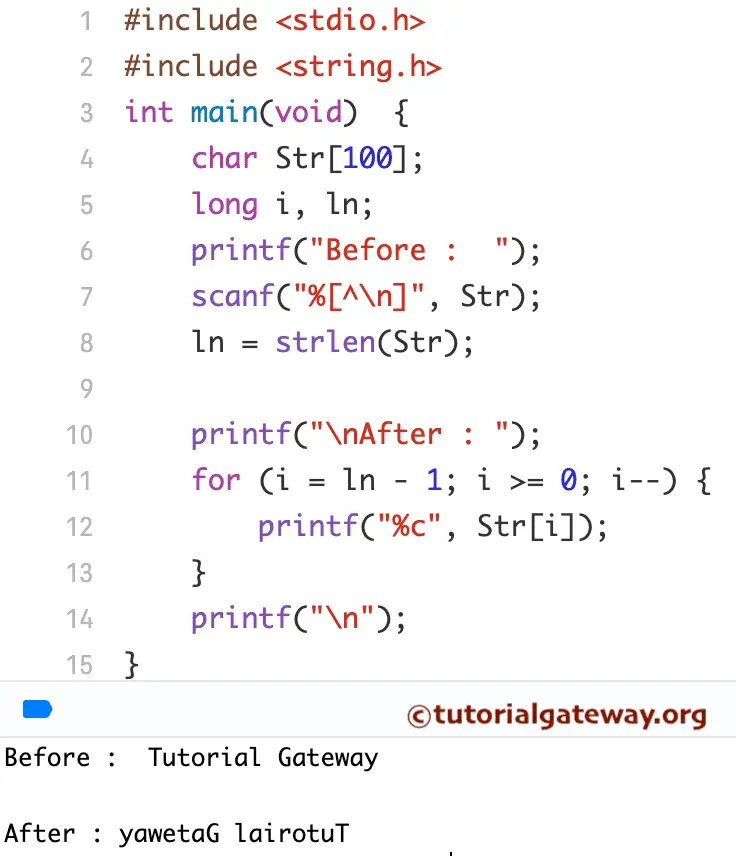
In this example, we use the temp variable to change the character’s location. It is something like a C program to reverse a string using the swapping technique.
/* using temp variable */ #include <stdio.h> #include <string.h> int main() { char Str[100], temp; int i, j, len; printf("\n Actual : "); gets(Str); len = strlen(Str) - 1; for (i = 0; i < strlen(Str)/2; i++) { temp = Str[i]; Str[i] = Str[len]; Str[len--] = temp; } printf("\n Result = %s", Str); return 0; }
Actual : C Programming
Result = gnimmargorP C
C program to Reverse a String Using Functions
This example is the same as above. However, we are using the recursive Functions to reverse the original and separate the logic from the main program this time.
#include <stdio.h> #include <string.h> void revText(char [], int, int); int main() { char Str[100], temp; int i, j, len; printf("\n Please Enter : "); gets(Str); len = strlen(Str); revText(Str, 0, len -1); printf("\n After = %s", Str); return 0; } void revText(char Str[], int i, int len) { char temp; temp = Str[i]; Str[i] = Str[len - i]; Str[len - i] = temp; if (i == len/2) { return; } revText(Str, i + 1, len); }
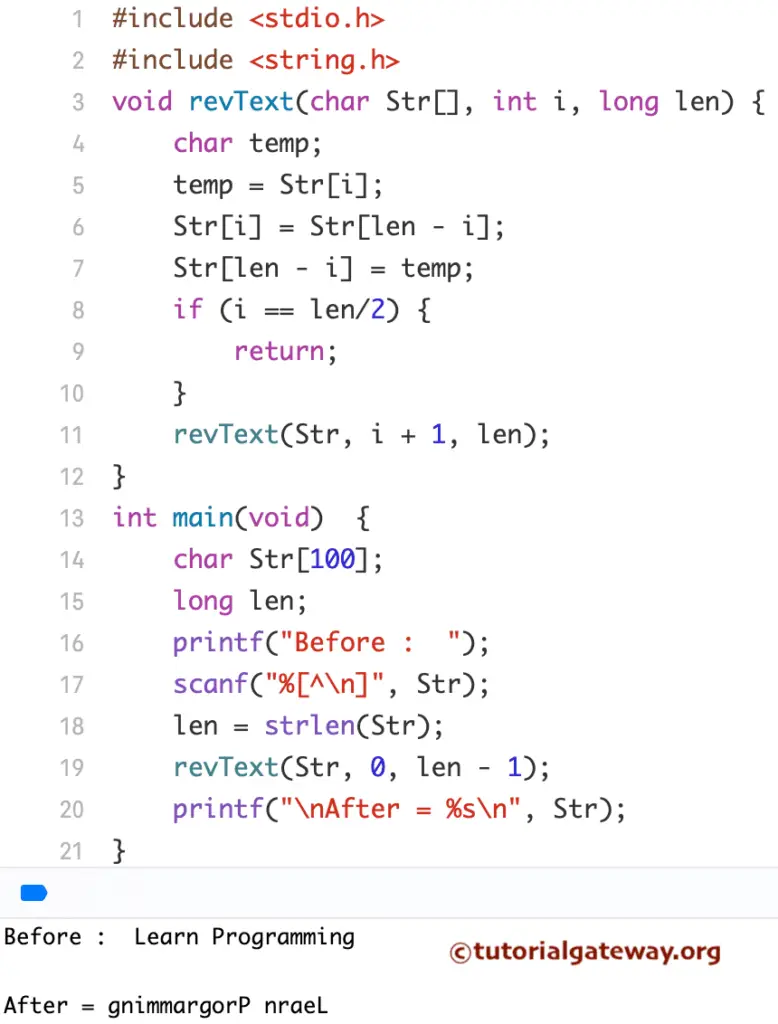
C program to Reverse a String Using Pointers
This program to reverse a string is the same as above, but we are using Pointers to separate the logic from the main function this time.
#include <stdio.h> #include <string.h> char* textRe(char *Str) { static int i = 0; static char RevStr[10]; if(*Str) { textRe(Str + 1); RevStr[i++] = *Str; } return RevStr; } int main() { char Str[100], temp; int i, j, len; printf("\n Enter : "); gets(Str); printf("\n Result = %s", textRe(Str)); return 0; }
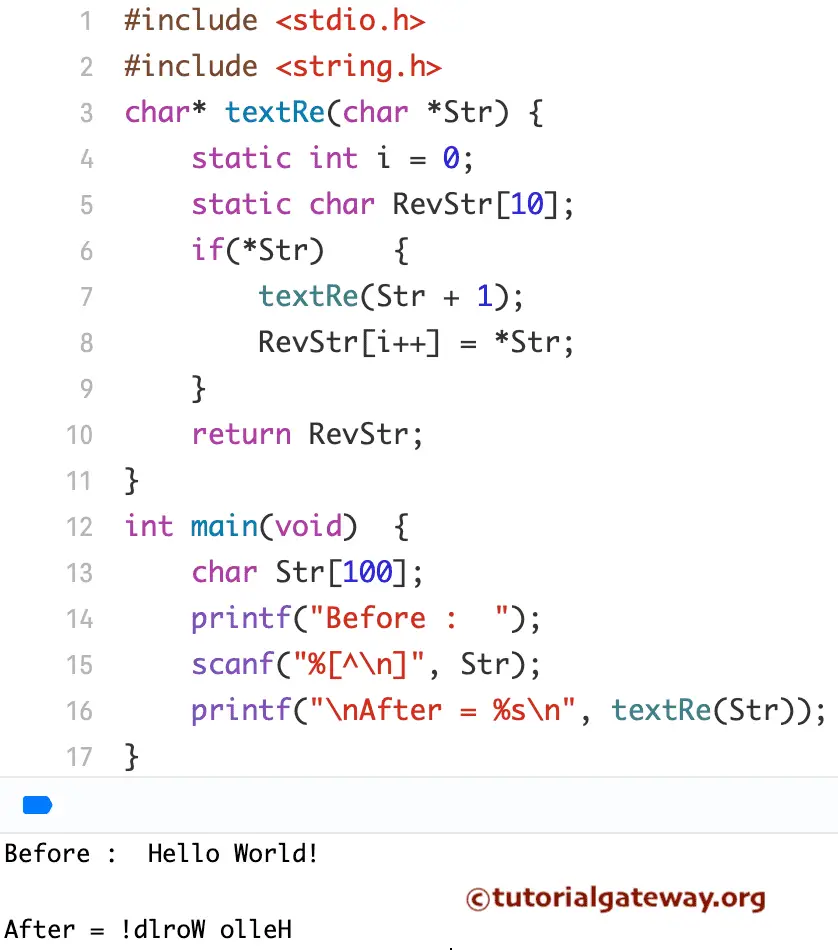