How to write a C Program to find First Occurrence of a Character in a String with an example?.
C Program to find First Occurrence of a Character in a String Example 1
This program allows the user to enter a string (or character array), and a character value. Next, it will search and find the first occurrence of a character inside a string using If Else Statement.
/* C Program to find First Occurrence of a Character in a String */ #include <stdio.h> #include <string.h> int main() { char str[100], ch; int i, Flag; Flag = 0; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Character that you want to Search for : "); scanf("%c", &ch); for(i = 0; i <= strlen(str); i++) { if(str[i] == ch) { Flag++; break; } } if(Flag == 0) { printf("\n Sorry!! We haven't found the Search Character '%c' ", ch); } else { printf("\n The First Occurrence of the Search Element '%c' is at Position %d ", ch, i + 1); } return 0; }
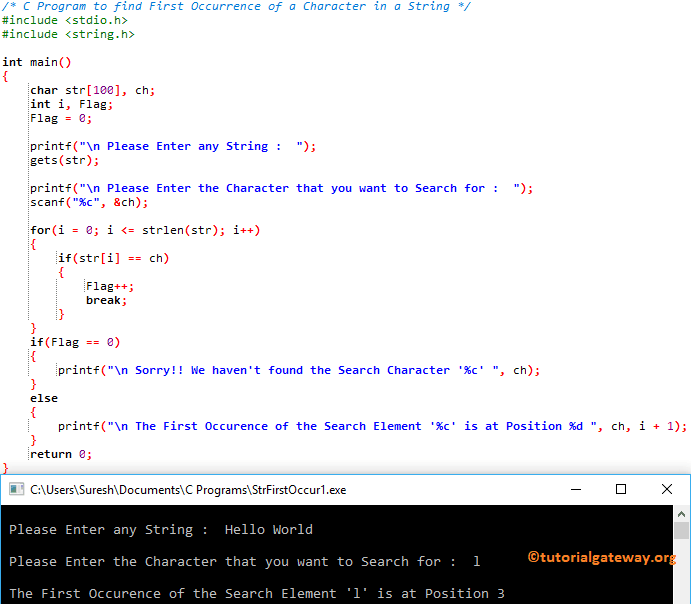
First, we used For Loop to iterate each character in a String.
for(i = 0; i <= strlen(str); i++) { if(str[i] == ch) { Flag++; break; } }
str[] = Hello World
ch = l
Flag = 0
Loop First Iteration: for(i = 0; i <= strlen(str); i++)
Within the For Loop, we used If statement to check whether the str[0] is equal to a user-specified character or not
if(str[i] == ch) => if(H == l)
The above condition is false. So, i will increment, and Flag is still 0.
Second Iteration: for(i = 1; 1 <= 11; 1++)
if(str[i] == ch) => if(e == l) – condition is false. So, i value will increment, and Flag is still 0.
Third Iteration: for(i = 2; 2 <= 11; 2++)
if(str[i] == ch) => if(l == l)
Above condition is True.
Flag++ means Flag = 1, and the break statement will exit from the loop
Next, we used the If Else Statement to check the Flag value is equal to 0. Here, the condition is False. So, the statement inside the else block will execute.
printf("\n The First Occurrence of the Search Element '%c' is at Position %d ", ch, i + 1);
Here, i is the index position (start with zero), and i + 1 is the actual position.
C Program to find First Occurrence of a Character in a String Example 2
This program is the same as above. Here, we just replaced the C Programming For Loop with While Loop.
/* C Program to find First Occurrence of a Character in a String */ #include <stdio.h> #include <string.h> int main() { char str[100], ch; int i, Flag; i = Flag = 0; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Character that you want to Search for : "); scanf("%c", &ch); while(str[i] != '\0') { if(str[i] == ch) { Flag++; break; } i++; } if(Flag == 0) { printf("\n Sorry!! We haven't found the Search Character '%c' ", ch); } else { printf("\n The First Occurence of the Search Element '%c' is at Position %d ", ch, i + 1); } return 0; }
Please Enter any String : tutorial gateway
Please Enter the Character that you want to Search for : a
The First Occurence of the Search Element 'a' is at Position 7
C Program to find First Occurrence of a Character in a String Example 3
This C program for first Character occurrence is the same as the first example. This time, we used the Functions concept to separate the logic.
/* C Program to find First Occurrence of a Character in a String */ #include <stdio.h> #include <string.h> int Find_FirstCharcater(char str[], char ch); int main() { char str[100], ch; int index; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Character that you want to Search for : "); scanf("%c", &ch); index = Find_FirstCharcater(str, ch); if(index == -1) { printf("\n Sorry!! We haven't found the Search Character '%c' ", ch); } else { printf("\n The First Occurrence of the Search Element '%c' is at Position %d ", ch, index + 1); } return 0; } int Find_FirstCharcater(char str[], char ch) { int i; for(i = 0; str[i] != '\0'; i++) { if(str[i] == ch) { return i; } } return -1; }
Please Enter any String : learn c programming
Please Enter the Character that you want to Search for : m
The First Occurrence of the Search Element 'm' is at Position 15
Program to find First Occurrence of a Character in a String Example 4
This program to find first character occurrence is the same as the first example. However, this time, we are using the concept of pointers.
/* C Program to find First Occurrence of a Character in a String */ #include <stdio.h> #include <string.h> int Find_FirstCharcater(char *str, char ch); int main() { char str[100], ch; int index; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Character that you want to Search for : "); scanf("%c", &ch); index = Find_FirstCharcater(str, ch); if(index == -1) { printf("\n Sorry!! We haven't found the Search Character '%c' ", ch); } else { printf("\n The First Occurrence of the Search Element '%c' is at Position %d ", ch, index + 1); } return 0; } int Find_FirstCharcater(char *str, char ch) { int i; while(*str) { if(*str == ch) { return i; } i++; str++; } return -1; }
Please Enter any String : programming examples
Please Enter the Character that you want to Search for : a
The First Occurrence of the Search Element 'a' is at Position 6