Write a Java Program to Find the Largest Array Number with an example or program to print or return the largest element or item in a given array. To get the largest number, we must consider the starting index position value as the max value and check the same against the remaining items. If any item is higher, replace it and print the output.
Java Program to Find Largest Array Number using for loop
In this example, we allow the user to enter the lrg_arr size and the items. Next, we assigned the lrg_arr first array item to the Largest variable. Then, we compare each item with this variable. If the Largest is less than any other lrg_arr item, we replace the variable value with that lrg_arr item.
For all this, we used the for loop and if statement.
import java.util.Scanner; public class Simple { private static Scanner sc; public static void main(String[] args) { int Size, i, Lar, Posi = 0; sc = new Scanner(System.in); System.out.print("\nPlease Enter the LRG size : "); Size = sc.nextInt(); int[] lrg_arr = new int[Size]; System.out.format("\nEnter LRG %d elements : ", Size); for(i = 0; i < Size; i++) { lrg_arr[i] = sc.nextInt(); } Lar = lrg_arr[0]; for(i = 1; i < Size; i++) { if(Lar < lrg_arr[i]) { Lar = lrg_arr[i]; Posi = i; } } System.out.format("\nLargest element in LRG Array = %d", Lar); System.out.format("\nIndex position of the Largest element = %d", Posi); } }
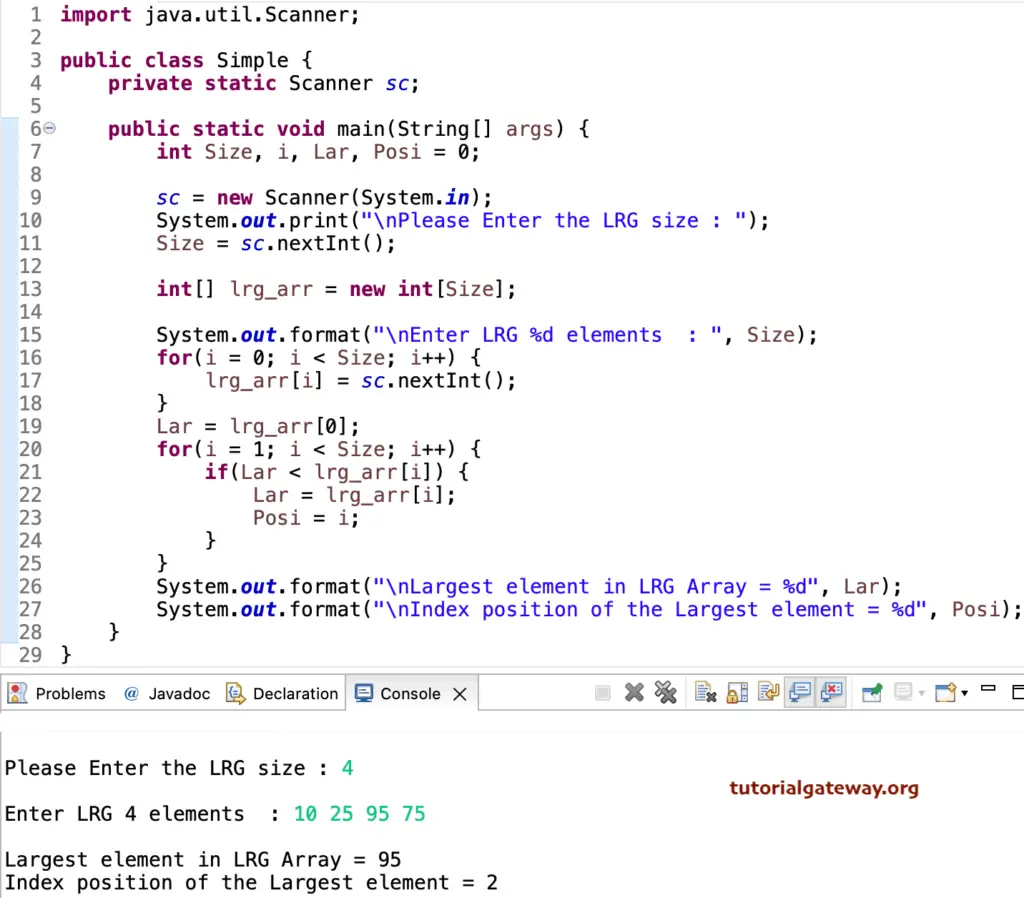
The Largest Array Number using functions
In this programming example, we created a separate function to return the largest item in the given array. Please refer to the C Program to get the highest number articles to understand the For loop program iteration.
import java.util.Scanner; public class LarElemUsingMethods { private static Scanner sc; static int Size, i, Lst, Position = 0; public static void main(String[] args) { int[] lrg_arr = new int[10]; sc = new Scanner(System.in); System.out.print("\nPlease Enter the LRG size : "); Size = sc.nextInt(); System.out.format("\nEnter LRG %d elements : ", Size); for(i = 0; i < Size; i++) { lrg_arr[i] = sc.nextInt(); } Lst = LstElent(lrg_arr); System.out.format("\nLargest element in LRG = %d", Lst); } public static int LstElent(int[] lrg_arr ) { Lst = lrg_arr[0]; for(i = 1; i < Size; i++) { if(Lst < lrg_arr[i]) { Lst = lrg_arr[i]; Position = i; } } return Lst; } }
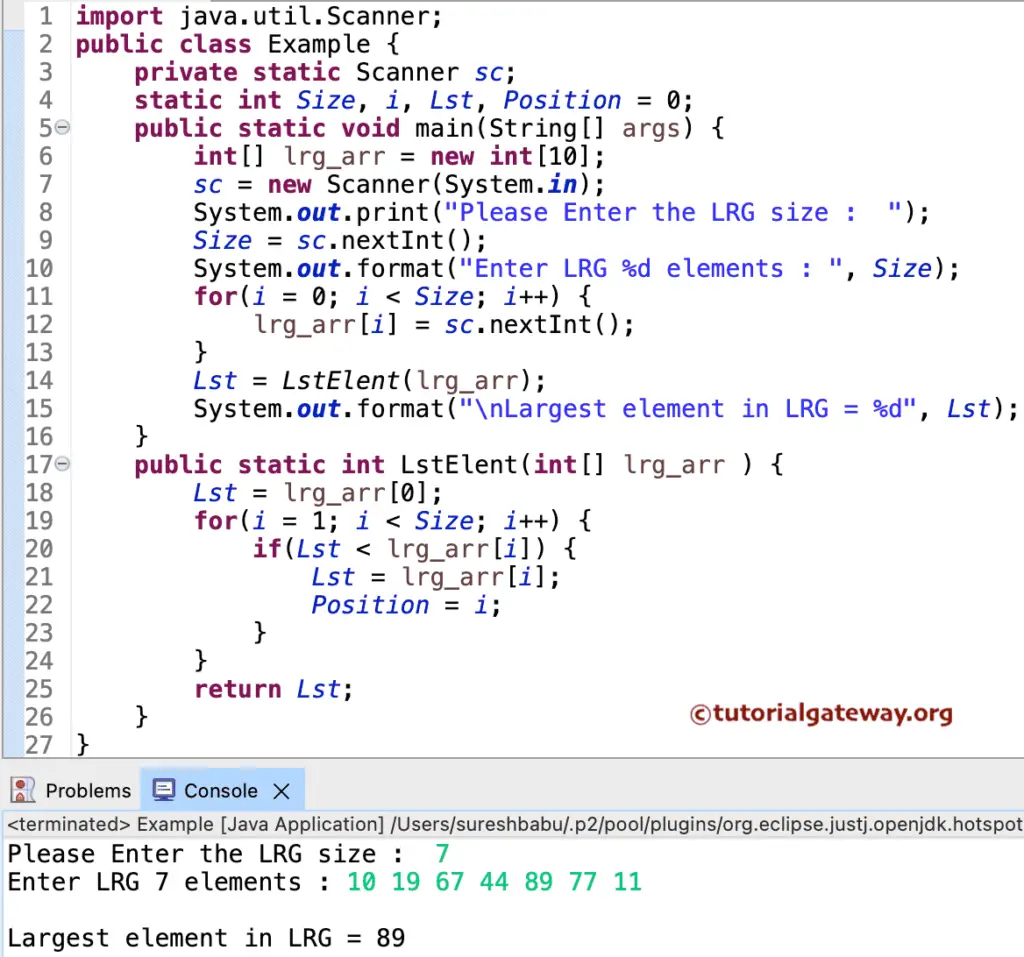