Write a Java Program to Convert Lowercase to Uppercase with an example. In this Java Lowercase to Uppercase example, we use the built-in function toUpperCase() on the given lowStr string.
import java.util.Scanner; public class LowerToUpper1 { private static Scanner sc; public static void main(String[] args) { String lowStr; sc= new Scanner(System.in); System.out.print("\nPlease Enter Lowercase String = "); lowStr = sc.nextLine(); String lowStr2 = lowStr.toUpperCase(); System.out.println("\nThe Uppercase String = " + lowStr2); } }
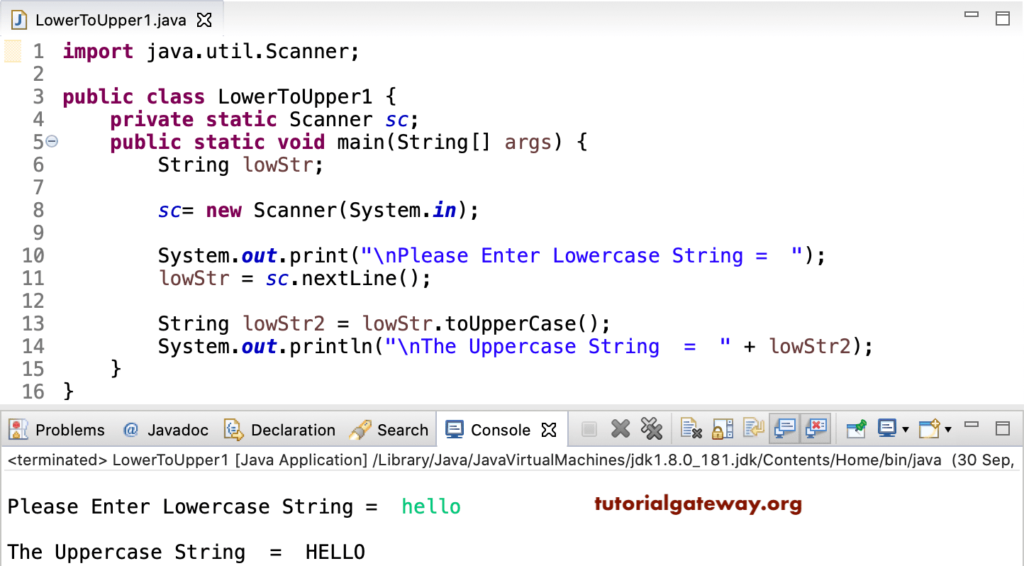
In this Convert Lowercase to Uppercase example, we first converted the lowStr string to ch character array using the toCharArray().
Next, we used a For loop to iterate the ch character array from start to end. Within the loop, we check whether the character at each index position is greater than or equal to a and less than or equal to z. If it is true, we are subtracting 32 to the current ASCII value. For example, a = 97, when we subtract 32, it becomes 65, and the ASCII value of A = 65.
import java.util.Scanner; public class LowerToUpper2 { private static Scanner sc; public static void main(String[] args) { String lowStr; int i; sc= new Scanner(System.in); System.out.print("\nPlease Enter Lowercase String = "); lowStr = sc.nextLine(); char[] ch = lowStr.toCharArray(); for(i = 0; i < ch.length; i++) { if(ch[i] >= 'a' && ch[i] <= 'z') { ch[i] = (char) ((int)ch[i] - 32);; } } System.out.print("\nThe Uppercase String = "); for(i = 0; i < ch.length; i++) { System.out.print(ch[i]); } } }
Java lowercase to uppercase output
Please Enter Lowercase String = java programming
The Uppercase String = JAVA PROGRAMMING
Java Program to Convert Lowercase to Uppercase Example 3
Here, we haven’t changed the lowStr string to char array. Next, we used the charAt function on the lowStr to get the character at the index position. Then, we assigned each character to uppStr2.
import java.util.Scanner; public class LowerToUpper3 { private static Scanner sc; public static void main(String[] args) { String lowStr, uppStr2 = ""; int i; sc= new Scanner(System.in); System.out.print("\nPlease Enter Lowercase String to convert = "); lowStr = sc.nextLine(); char ch; for(i = 0; i < lowStr.length(); i++) { if(lowStr.charAt(i) >= 'a' && lowStr.charAt(i) <= 'z') { ch = (char) (lowStr.charAt(i) - 32); } else { ch = (char) (lowStr.charAt(i)); } uppStr2 += ch; } System.out.print("\nThe Uppercase String = " + uppStr2); } }
Java convert lower to upper output
Please Enter Lowercase String to convert = tutorial gateway
The Uppercase String = TUTORIAL GATEWAY
In this Java Lowercase to Uppercase example, instead of assigning it to a new uppStr2 string, we print each character within the loop.
import java.util.Scanner; public class LowerToUpper4 { private static Scanner sc; public static void main(String[] args) { String lowStr; int i; sc= new Scanner(System.in); System.out.print("\nPlease Enter Lowercase String to convert = "); lowStr = sc.nextLine(); System.out.print("\nThe Uppercase String = "); for(i = 0; i < lowStr.length(); i++) { char ch = lowStr.charAt(i); if(ch >= 'a' && ch <= 'z') { ch = (char) (ch - 32); } System.out.print(ch); // Str2 += ch; } } }
Please Enter Lowercase String to convert = learn Java
The Uppercase String = LEARN JAVA
In this Java Program to Convert Lowercase to Uppercase using ASCII Values, we compare the ASCII values instead of comparing characters.
import java.util.Scanner; public class LowerToUpper5 { private static Scanner sc; public static void main(String[] args) { String lowStr, uppStr2 = ""; int i; sc= new Scanner(System.in); System.out.print("\nPlease Enter Lowercase String = "); lowStr = sc.nextLine(); for(i = 0; i < lowStr.length(); i++) { char ch = lowStr.charAt(i); if(ch >= 97 && ch <= 122) { ch = (char) (ch - 32); } uppStr2 += ch; } System.out.print("\nThe Uppercase String = " + uppStr2); } }
Please Enter Lowercase String = lowercase char
The Uppercase String = LOWERCASE CHAR
This Java code for Lowercase to Uppercase, we separated the logic using functions.
import java.util.Scanner; public class LowerToUpper6 { private static Scanner sc; public static void main(String[] args) { String lowStr, uppStr2; sc= new Scanner(System.in); System.out.print("\nPlease Enter Lowercase String = "); lowStr = sc.nextLine(); uppStr2 = toUpper(lowStr); System.out.print("\nThe Uppercase String = " + uppStr2); } public static String toUpper(String lowStr) { String uppStr2 = ""; for(int i = 0; i < lowStr.length(); i++) { char ch = lowStr.charAt(i); if(ch >= 97 && ch <= 122) { ch = (char) (ch - 32); } uppStr2 += ch; } return uppStr2; } }
Please Enter Lowercase String = java lowercase coding
The Uppercase String = JAVA LOWERCASE CODING