We write the traditional or common Hello World program in any programming language. In this golang tutorial also, we start writing the hello world program in our Visual Studio Code Editor. You can use any of your favorite editors to write your programs.
The following golang program code will print the Hello World message as an output of the terminal. Let me save this code as helloworld.go. Here, go is the extension of the program that we write in golang.
package main import "fmt" func main() { fmt.Println("Hello World") }
To run this example, go to the terminal and navigate to the folder where you saved the example using the cd command. Next, type go run program_Name.go. Here, it is
go run helloworld.go
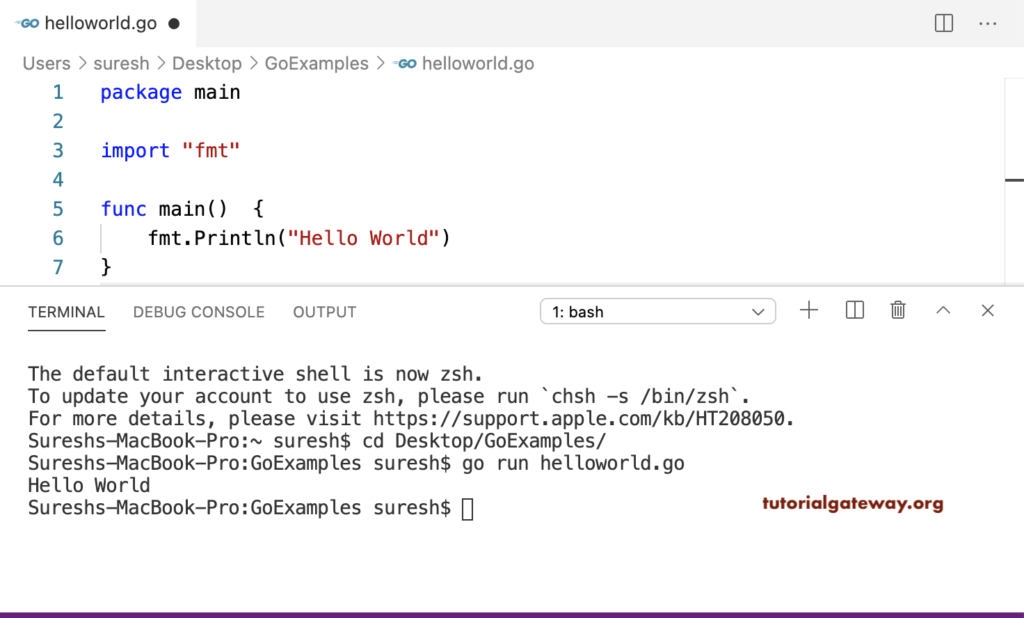
golang Hello World Program Analysis
The Go compiler executes the code from top to bottom. Every Go program must start with the package declaration.
package main
In the next line, we are importing the fmt (format) package into the example. It is the standard library that contains the common input and output functions.
Every function starts with the keyword func. Within this main function, we used the Println function to print the output. If you don’t use the import “fmt”, you can’t use this Println function.
Golang Hello World Program 2
In this simple program, we used for loop to print the hello world message five times.
package main import "fmt" func main() { for i := 1; i <= 5; i++ { fmt.Println("Hello World") } }
Hello World
Hello World
Hello World
Hello World
Hello World