A leap year contains 366 days, and any year divisible by four except the century years are called leap years. Furthermore, if the century year divisible by 400, then it is a leap year. In this Go program, we use the If else statement (if yr%400 == 0 || (yr%4 == 0 && yr%100 != 0)) and logical operators to check leap year or not.
package main import "fmt" func main() { var yr int fmt.Print("\nEnter the Year to Check for Leap = ") fmt.Scanln(&yr) if yr%400 == 0 || (yr%4 == 0 && yr%100 != 0) { fmt.Println(yr, " is a Leap Year") } else { fmt.Println(yr, " is Not a Leap Year") } }
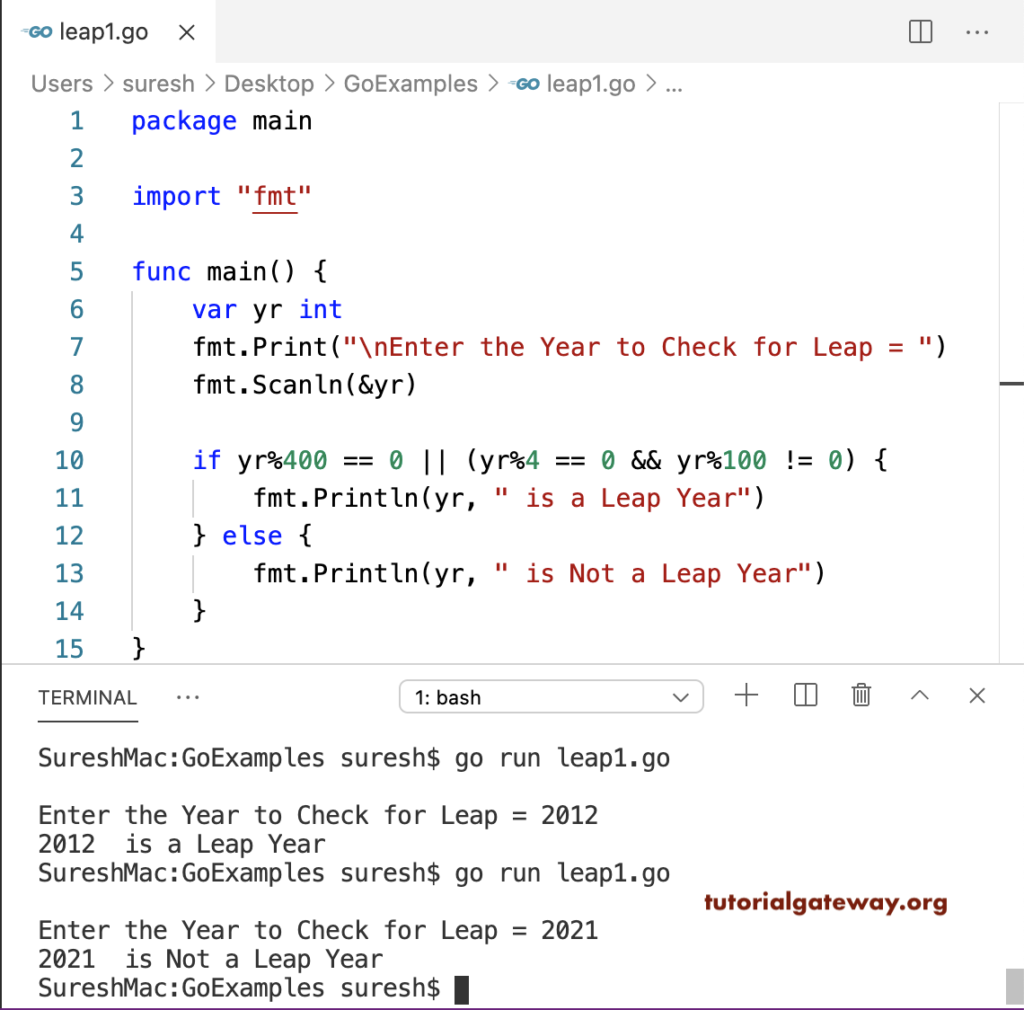
Golang Program to Check Leap year using Else If
package main import "fmt" func main() { var yr int fmt.Print("\nEnter the Year to Check for Leap = ") fmt.Scanln(&yr) if yr%400 == 0 { fmt.Println(yr, " is a Leap Year") } else if yr%100 == 0 { fmt.Println(yr, " is Not a Leap Year") } else if yr%4 == 0 { fmt.Println(yr, " is a Leap Year") } else { fmt.Println(yr, " is Not a Leap Year") } }
SureshMac:Goexamples suresh$ go run leap2.go
Enter the Year to Check for Leap = 1990
1990 is Not a Leap Year
SureshMac:Goexamples suresh$ go run leap2.go
Enter the Year to Check for Leap = 2020
2020 is a Leap Year
In this Go program, we used the Nested if statement to find whether the user-entered year is a leap year or not.
package main import "fmt" func main() { var yr int fmt.Print("\nEnter the Year to Check for Leap = ") fmt.Scanln(&yr) if yr%4 == 0 { if yr%100 == 0 { if yr%400 == 0 { fmt.Println(yr, " is a Leap Year") } else { fmt.Println(yr, " is Not a Leap Year") } } else { fmt.Println(yr, " is a Leap Year") } } else { fmt.Println(yr, " is Not a Leap Year") } }
SureshMac:Goexamples suresh$ go run leap3.go
Enter the Year to Check for Leap = 1200
1200 is a Leap Year
SureshMac:Goexamples suresh$ go run leap3.go
Enter the Year to Check for Leap = 2015
2015 is Not a Leap Year