In this Go Program to add two arrays, we allow users to enter the size and the array items. Next, we used for loop (for i = 0; i < size; i++) to iterate the array items from 1 to size. Inside the loop, (additionArr[i] = addArr1[i] + addArr2[i]) we added the two array items at each index position.
package main import "fmt" func main() { var size, i int fmt.Print("Enter the Array Size = ") fmt.Scan(&size) addArr1 := make([]int, size) addArr2 := make([]int, size) additionArr := make([]int, size) fmt.Print("Enter the First Array Items to Perform Addition = ") for i = 0; i < size; i++ { fmt.Scan(&addArr1[i]) } fmt.Print("Enter the Second Array Items to Perform Addition = ") for i = 0; i < size; i++ { fmt.Scan(&addArr2[i]) } fmt.Print("The Sum or Addition of Two Arrays = ") for i = 0; i < size; i++ { additionArr[i] = addArr1[i] + addArr2[i] fmt.Print(additionArr[i], " ") } fmt.Println() }
Enter the Array Size = 5
Enter the First Array Items to Perform Addition = 1 2 3 4 5
Enter the Second Array Items to Perform Addition = 5 9 11 7 10
The Sum or Addition of Two Arrays = 6 11 14 11 15
Go Program to Add Two Arrays Example 2
In this Golang example, we used the for loop range (for x, _ := range addArr1) and performed the addition. You can also rewrite the for loop as (for x := range addArr1). To show you the syntax, we left that _.
package main import "fmt" func main() { var i int var addArr1 [5]int var addArr2 [5]int var additionArr [5]int fmt.Print("Enter the First Array Items to Perform Addition = ") for i = 0; i < 5; i++ { fmt.Scan(&addArr1[i]) } fmt.Print("Enter the Second Array Items to Perform Addition = ") for i = 0; i < 5; i++ { fmt.Scan(&addArr2[i]) } fmt.Print("The Sum or Addition of Two Arrays = ") for x, _ := range addArr1 { additionArr[x] = addArr1[x] + addArr2[x] fmt.Print(additionArr[x], " ") } fmt.Println() }
Enter the First Array Items to Perform Addition = 3 5 7 9 11
Enter the Second Array Items to Perform Addition = 22 99 88 9 3
The Sum or Addition of Two Arrays = 25 104 95 18 14
In this Golang program, to add two arrays, we used for loop range to insert and add the array items.
package main import "fmt" func main() { var size int fmt.Print("Enter the Multiplication Array Size = ") fmt.Scan(&size) addArr1 := make([]int, size) addArr2 := make([]int, size) additionArr := make([]int, size) fmt.Print("Enter the First Array Items to Perform Addition = ") for i := range addArr1 { fmt.Scan(&addArr1[i]) } fmt.Print("Enter the Second Array Items to Perform Addition = ") for j := range addArr2 { fmt.Scan(&addArr2[j]) } fmt.Print("The Sum or Addition of Two Arrays = ") for x := range additionArr { additionArr[x] = addArr1[x] + addArr2[x] fmt.Print(additionArr[x], " ") } fmt.Println() }
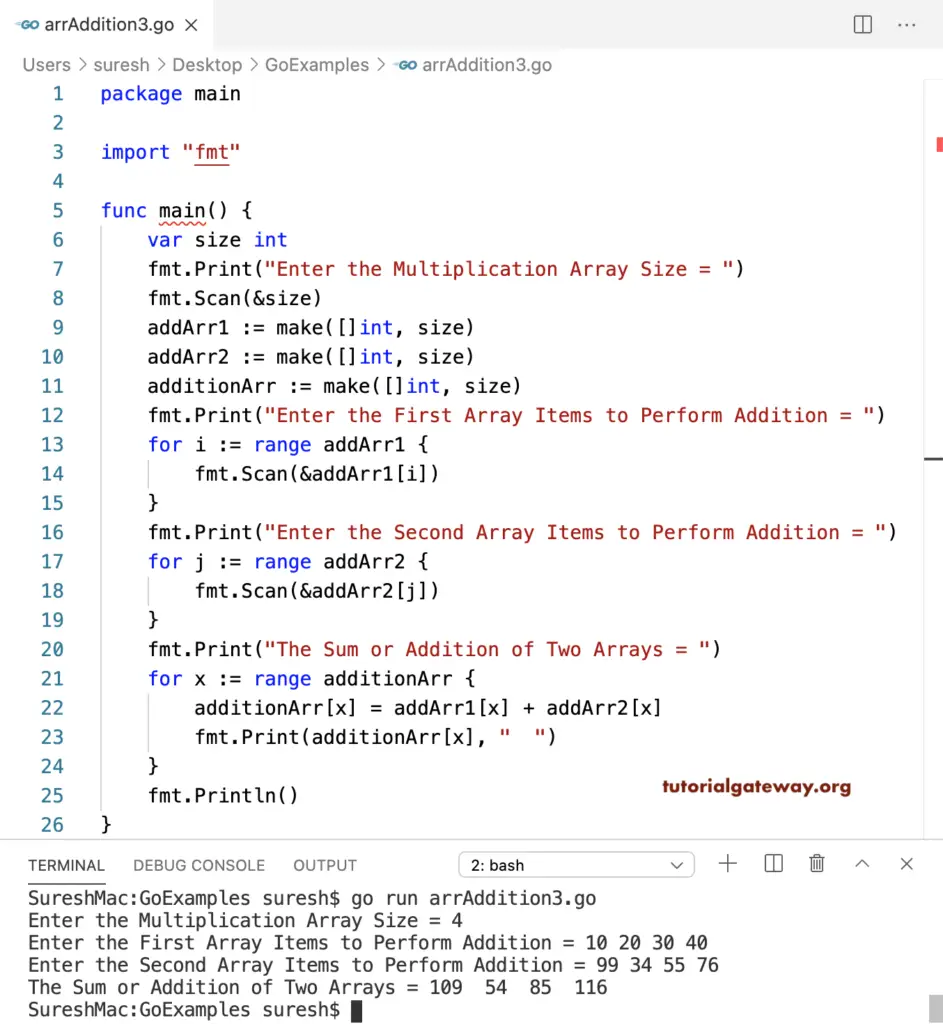