Write a Go program to swap two numbers using a temp variable and without using a temporary variable. In the below example, we allow users to enter two variables and use the temp variable to swap them.
package main import "fmt" func main() { var a, b, temp int fmt.Print("Enter the First = ") fmt.Scanln(&a) fmt.Print("Enter the Second = ") fmt.Scanln(&b) temp = a a = b b = temp fmt.Println("The First Number after = ", a) fmt.Println("The Second Number after = ", b) }
Enter the First = 10
Enter the Second = 20
The First Number after = 20
The Second Number after = 10
Go Program to Swap Two Numbers without Temp variable.
In this example, we used the Golang arithmetic operators to swap two numbers.
package main import "fmt" func main() { var a, b int fmt.Print("Enter the a Value = ") fmt.Scanln(&a) fmt.Print("Enter the b Value = ") fmt.Scanln(&b) a = a + b b = a - b a = a - b fmt.Println("a Value after Swap = ", a) fmt.Println("b Value after Swap = ", b) }
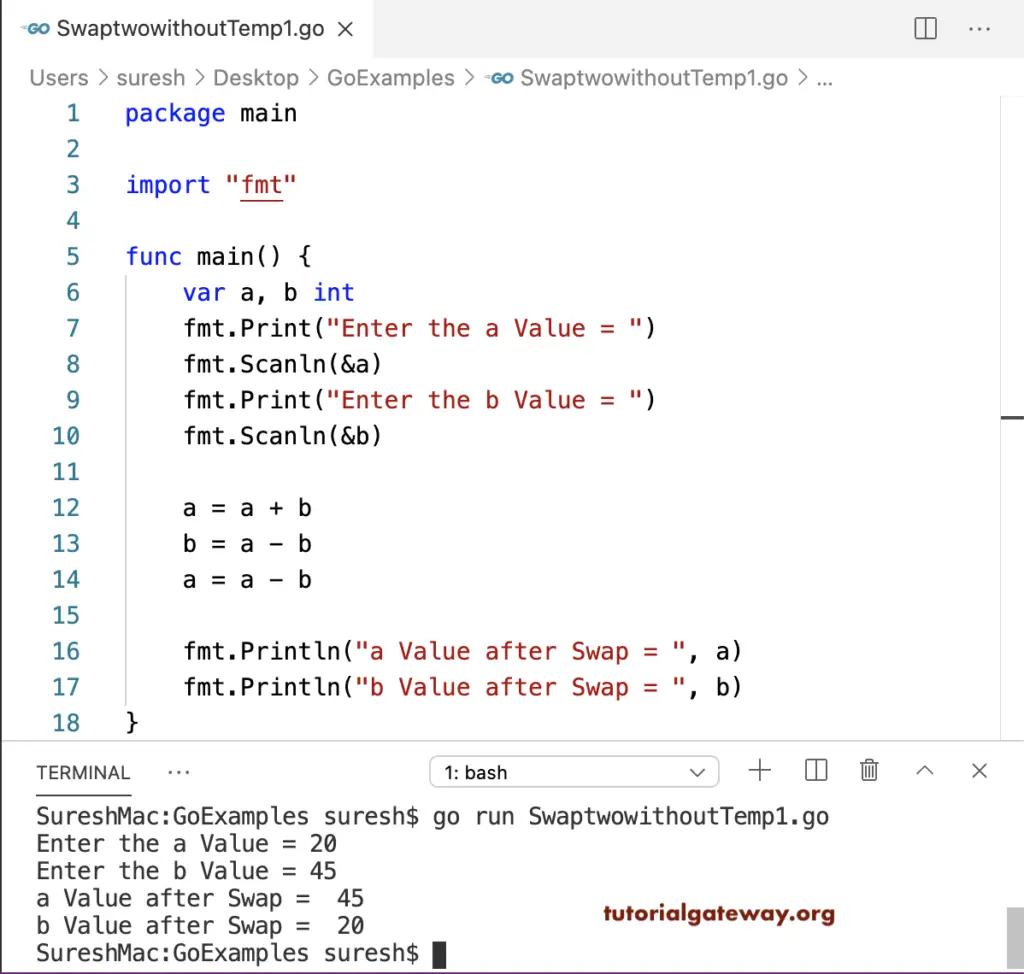
This Program will Swap two numbers using Bitwise Operators.
package main import "fmt" func main() { var a, b int fmt.Print("Enter the a Value = ") fmt.Scanln(&a) fmt.Print("Enter the b Value = ") fmt.Scanln(&b) a = a ^ b b = a ^ b a = a ^ b fmt.Println("a Value after = ", a) fmt.Println("b Value after = ", b) }
Enter the a Value = 99
Enter the b Value = 240
a Value after = 240
b Value after = 99