In this Go program to print array items, we declared an array of five integer values. We can use the println statement to print the array items.
package main import "fmt" func main() { numArr := [5]int{10, 20, 30, 50, 70} fmt.Println(numArr) }
[10 20 30 50 70]
Go Program to Print Array Items using For Loop
The Golang for loop iterate s from zero to 5 (max size) (for i := 0; i < 5; i++) and prints the array items.
package main import "fmt" func main() { numArr := [5]int{10, 20, 30, 50, 70} fmt.Print("\nThe List of Array Elements in numArr = ") for i := 0; i < 5; i++ { fmt.Print(numArr[i], " ") } fmt.Println() }
The List of Array Elements in numArr = 10 20 30 50 70
In this Golang example, we used the length of an array (for i := 0; i < len(numArr); i++) as the for loop condition.
package main import "fmt" func main() { numArr := [7]int{22, 19, 15, 62, 37, 50, 70} fmt.Println("The List of Array Elements in numArr = ") for i := 0; i < len(numArr); i++ { fmt.Println("The Array Item at ", i, "Index Position = ", numArr[i]) } }
The List of Array Elements in numArr =
The Array Item at 0 Index Position = 22
The Array Item at 1 Index Position = 19
The Array Item at 2 Index Position = 15
The Array Item at 3 Index Position = 62
The Array Item at 4 Index Position = 37
The Array Item at 5 Index Position = 50
The Array Item at 6 Index Position = 70
This Go program allows users to enter the size and the array items. Next, we print those array items.
package main import "fmt" func main() { var arrsize, i int var numArr [10]int fmt.Print("Enter the Even Array Size = ") fmt.Scan(&arrsize) fmt.Print("Enter the Even Array Items = ") for i = 0; i < arrsize; i++ { fmt.Scan(&numArr[i]) } fmt.Println("**** The List of Array Elements in numArr ****") for i = 0; i < arrsize; i++ { fmt.Println("The Array Item at ", i, "Index Position = ", numArr[i]) } }
Enter the Even Array Size = 5
Enter the Even Array Items = 2 5 7 9 3
**** The List of Array Elements in numArr ****
The Array Item at 0 Index Position = 2
The Array Item at 1 Index Position = 5
The Array Item at 2 Index Position = 7
The Array Item at 3 Index Position = 9
The Array Item at 4 Index Position = 3
This Golang Program returns the Array Items using For Loop Range (for i, val := range numArr). Here, i is the index position, and val is the actual value.
package main import "fmt" func main() { var arrsize int fmt.Print("Enter the Even Array Size = ") fmt.Scan(&arrsize) numArr := make([]int, arrsize) fmt.Print("Enter the Even Array Items = ") for i := 0; i < arrsize; i++ { fmt.Scan(&numArr[i]) } fmt.Println("**** The List of Array Elements in numArr ****") for i, val := range numArr { fmt.Println("The Array Item at ", i, "Index Position = ", val) } }
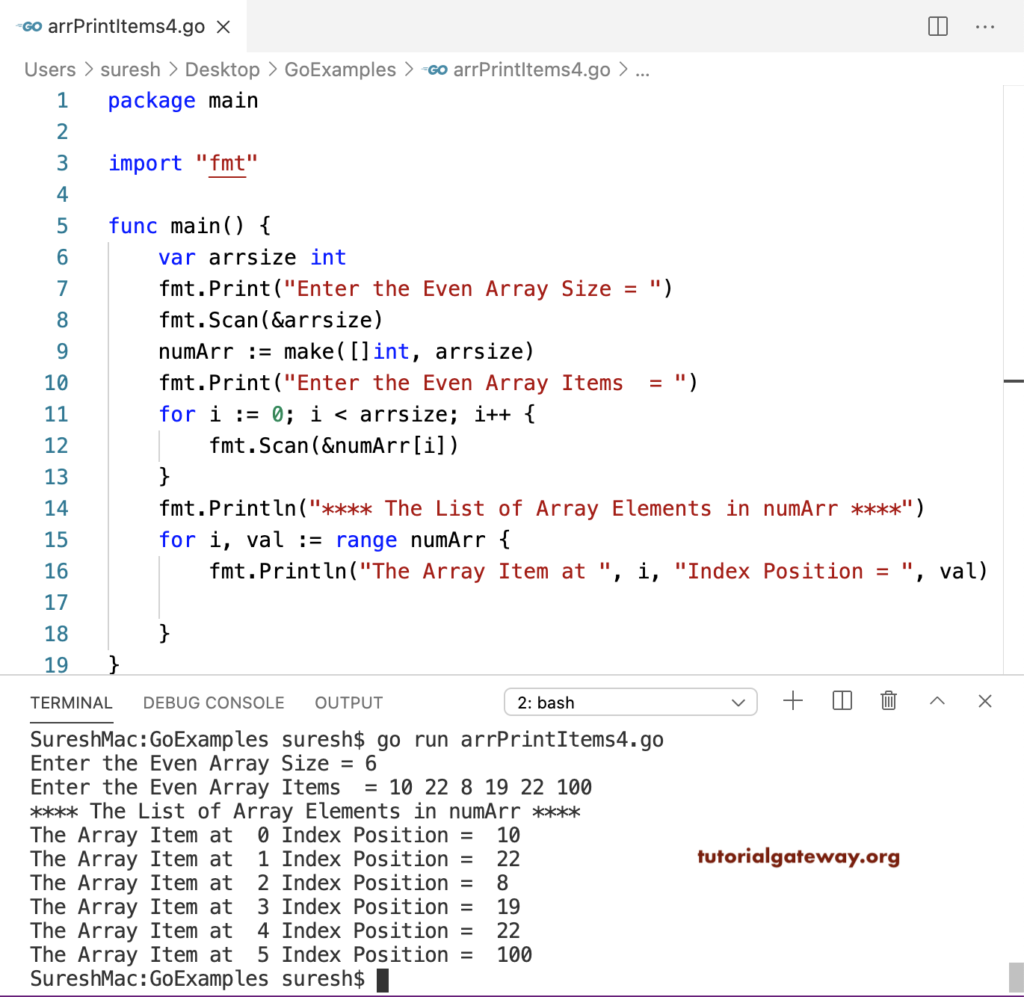