This Go program to find factors of a number uses a for loop that starts from 1 and iterates until n. The If statement (factorsnum%i == 0) checks whether the remainder of the factorsNum and I value is equal o zero. If True, then print that factorial number.
package main import "fmt" func main() { var factorsnum, i int fmt.Print("Enter any Number to find Factors = ") fmt.Scanln(&factorsnum) fmt.Println("The Factors of the ", factorsnum, " are = ") for i = 1; i <= factorsnum; i++ { if factorsnum%i == 0 { fmt.Println(i) } } }
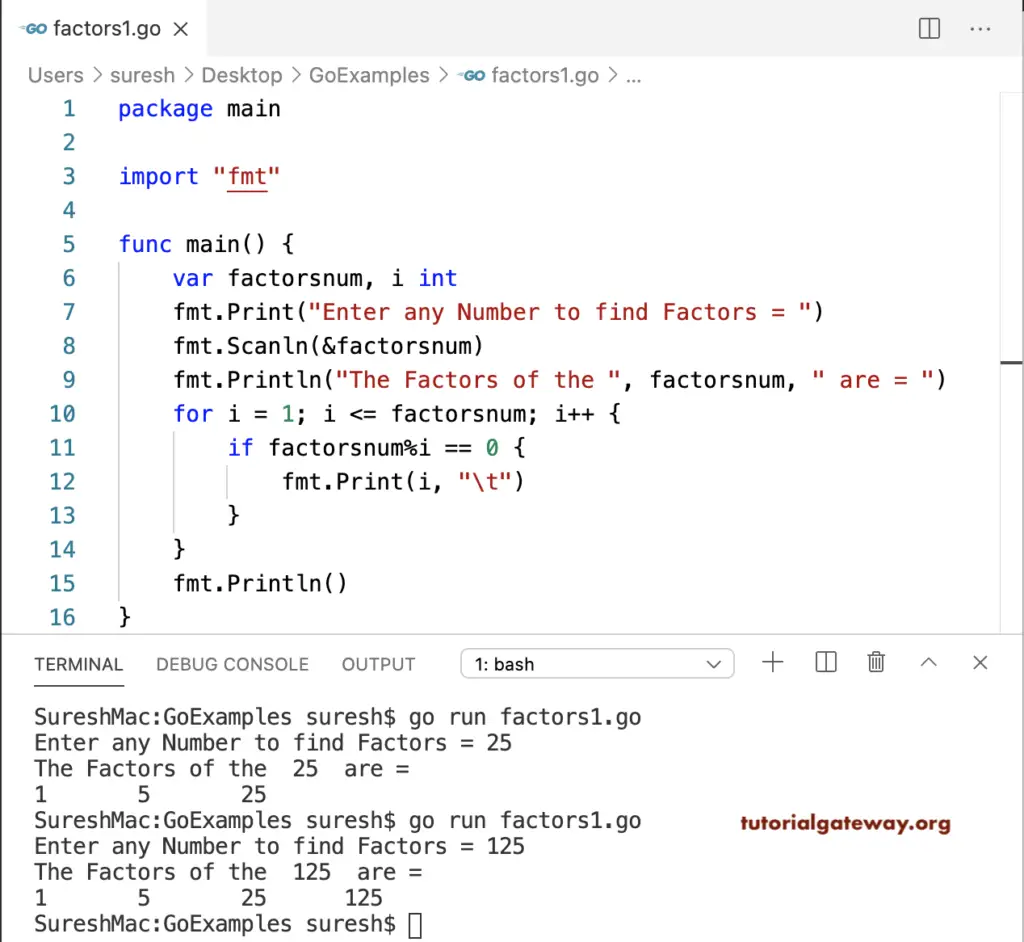
Golang Program to find Factors of a Numbers using Functions.
package main import "fmt" func findFactors(factorsnum int) { for i := 1; i <= factorsnum; i++ { if factorsnum%i == 0 { fmt.Println(i) } } } func main() { var factorsnum int fmt.Print("Enter any Number to find Factors = ") fmt.Scanln(&factorsnum) fmt.Println("The Factors of the ", factorsnum, " are = ") findFactors(factorsnum) }
SureshMac:Goexamples suresh$ go run factors2.go
Enter any Number to find Factors = 50
The Factors of the 50 are =
1
2
5
10
25
50
SureshMac:Goexamples suresh$ go run factors2.go
Enter any Number to find Factors = 76
The Factors of the 76 are =
1
2
4
19
38
76