In this Go program, the iteration of the for loop starts at A and ends at Z to return or display the alphabets between uppercase A to Z.
package main import ( "fmt" ) func main() { fmt.Println("The List of Alphabets from A to Z are :") for ch := 'A'; ch <= 'Z'; ch++ { fmt.Printf("%c ", ch) } fmt.Println() }
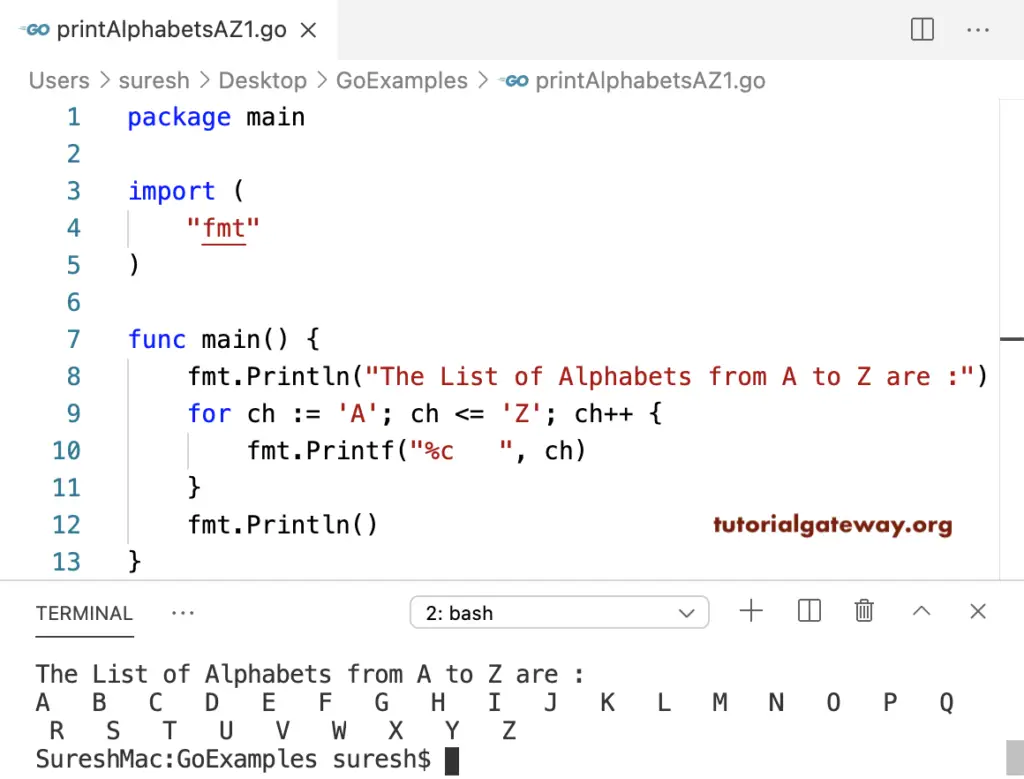
In this Golang Program, we used the for loop to iterate the ASCII codes (for ch := 65; ch <= 90; ch++) and display the Alphabets from A to Z.
package main import ( "fmt" ) func main() { fmt.Println("The List of Alphabets from A to Z are :") for ch := 65; ch <= 90; ch++ { fmt.Printf("%c ", ch) } fmt.Println() }
The List of Alphabets from A to Z are :
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
This Go example allows entering the starting uppercase character (for ch := stch; ch <= ‘Z’; ch++) and prints the alphabets from that to Z.
package main import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter the Starting Character = ") stch, _ := reader.ReadByte() fmt.Printf("The List of Alphabets from %c to Z are :\n", stch) for ch := stch; ch <= 'Z'; ch++ { fmt.Printf("%c ", ch) } fmt.Println() }
Enter the Starting Character = K
The List of Alphabets from K to Z are :
K L M N O P Q R S T U V W X Y Z