Write a Go Program to Print Reversed Mirrored Right Angled Triangle Star Pattern. In this Golang Reversed Mirrored Right Triangle example, the nested for loops iterates rows from start to end. The if statement (if j < i) checks whether the column value is less than the row. If True, it prints empty space; otherwise, print stars.
package main import "fmt" func main() { var i, j, rows int fmt.Print("Reversed Mirrored Right Triangle Rows = ") fmt.Scanln(&rows) fmt.Println("Reversed Mirrored Right Triangle Star Pattern") for i = 1; i <= rows; i++ { for j = 1; j <= rows; j++ { if j < i { fmt.Print(" ") } else { fmt.Print("*") } } fmt.Println() } }
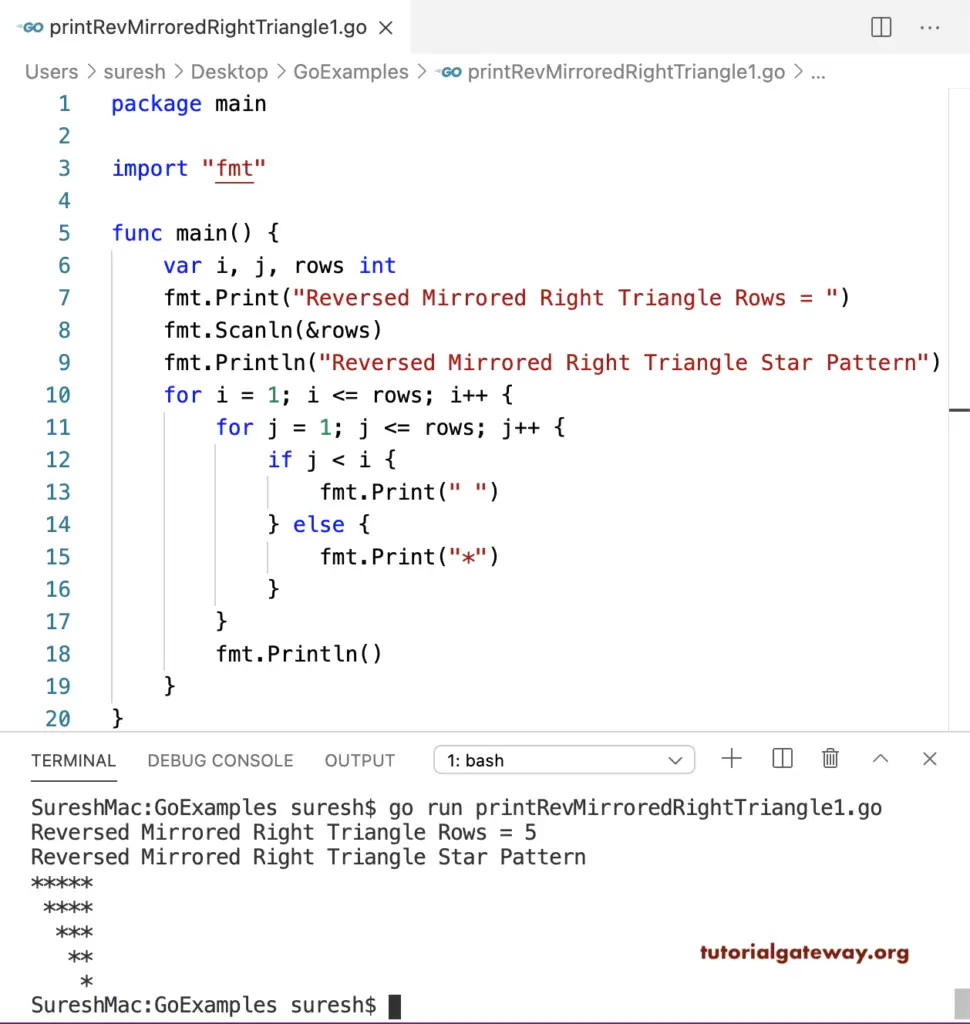
This Golang program allows entering a symbol and prints that symbol in Reversed Mirrored Right angled Triangle pattern.
package main import "fmt" func main() { var i, j, rows int var ch string fmt.Print("Rows for Reversed Mirrored Right Triangle = ") fmt.Scanln(&rows) fmt.Print("Symbol for Reversed Mirrored Right Triangle = ") fmt.Scanln(&ch) fmt.Println("Reversed Mirrored Right Triangle Pattern") for i = 1; i <= rows; i++ { for j = 1; j <= rows; j++ { if j < i { fmt.Print(" ") } else { fmt.Printf("%s", ch) } } fmt.Println() } }
Rows for Reversed Mirrored Right Triangle = 11
Symbol for Reversed Mirrored Right Triangle = #
Reversed Mirrored Right Triangle Pattern
###########
##########
#########
########
#######
######
#####
####
###
##
#