This Go program uses nested for loop to print multiplication tables of 9 and 10. The outer for loop to iterate values from 9 to 10, and the inner for loop to iterate from 1 to 20. If you want the multiplication table to display upto 20, change the nested for loop condition to j <= 20.
package main import "fmt" func main() { var i, j int fmt.Println("\nMultiplication Table of 9 and 10 are = ") for i = 9; i <= 10; i++ { for j = 1; j <= 10; j++ { fmt.Println(i, " * ", j, " = ", i*j) } fmt.Println("=======") } }
Multiplication Table of 9 and 10 are =
9 * 1 = 9
9 * 2 = 18
9 * 3 = 27
9 * 4 = 36
9 * 5 = 45
9 * 6 = 54
9 * 7 = 63
9 * 8 = 72
9 * 9 = 81
9 * 10 = 90
=======
10 * 1 = 10
10 * 2 = 20
10 * 3 = 30
10 * 4 = 40
10 * 5 = 50
10 * 6 = 60
10 * 7 = 70
10 * 8 = 80
10 * 9 = 90
10 * 10 = 100
=======
Golang program to Print Multiplication Table
This program allows entering any number less than 10. Next, it prints the multiplication tables from that number to 10.
package main import "fmt" func main() { var i, j int fmt.Print("\nEnter the Number Less than or Equal to 10 = ") fmt.Scanln(&i) fmt.Println("\nMultiplication Tables upto 10 are = ") for i <= 10 { for j = 1; j <= 10; j++ { fmt.Println(i, " * ", j, " = ", i*j) } i++ fmt.Println("=======") } }
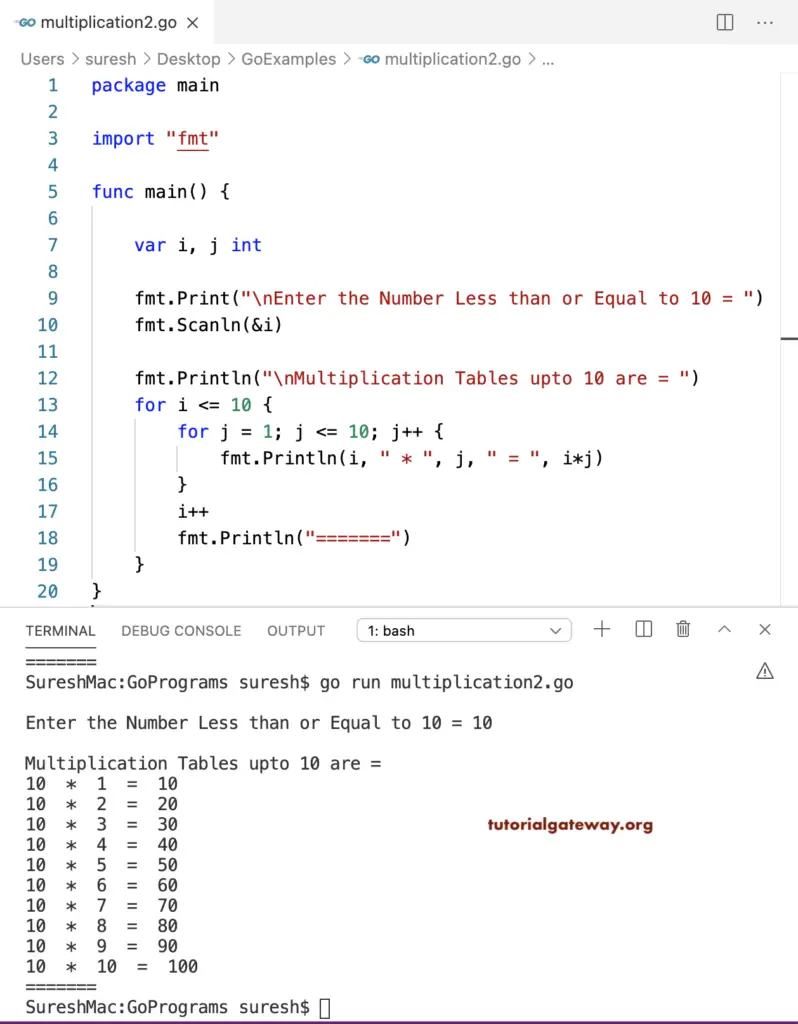