Write a Go Program to print the upper triangle of a Matrix. In this Go Matrix Upper Triangle example, the nested for loop iterates the matrix row and columns. Inside the loop, if statement (if j >= i) verifies whether the column value is greater than or equal to row value. If True, print that item as the upper triangle matrix item, and if the condition results in false, print zero.
package main import "fmt" func main() { var i, j, rows, columns int var upTriangleMat [10][10]int fmt.Print("Enter the Upper Matrix rows and Columns = ") fmt.Scan(&rows, &columns) fmt.Println("Enter Matrix Items to Print Upper Triangle = ") for i = 0; i < rows; i++ { for j = 0; j < columns; j++ { fmt.Scan(&upTriangleMat[i][j]) } } for i = 0; i < rows; i++ { fmt.Println() for j = 0; j < columns; j++ { if j >= i { fmt.Print(upTriangleMat[i][j], " ") } else { fmt.Print("0 ") } } } fmt.Println() }
Enter the Upper Matrix rows and Columns = 2 2
Enter Matrix Items to Print Upper Triangle =
10 20
30 40
10 20
0 40
Golang Program to print the Upper Triangle Matrix using For loop range.
package main import "fmt" func main() { var upTriangleMat [3][3]int fmt.Println("Enter Matrix Items to Print Upper Triangle = ") for i, rows := range upTriangleMat { for j := range rows { fmt.Scan(&upTriangleMat[i][j]) } } for i, rows := range upTriangleMat { fmt.Println() for j := range rows { if j >= i { fmt.Print(upTriangleMat[i][j], " ") } else { fmt.Print("0 ") } } } fmt.Println() }
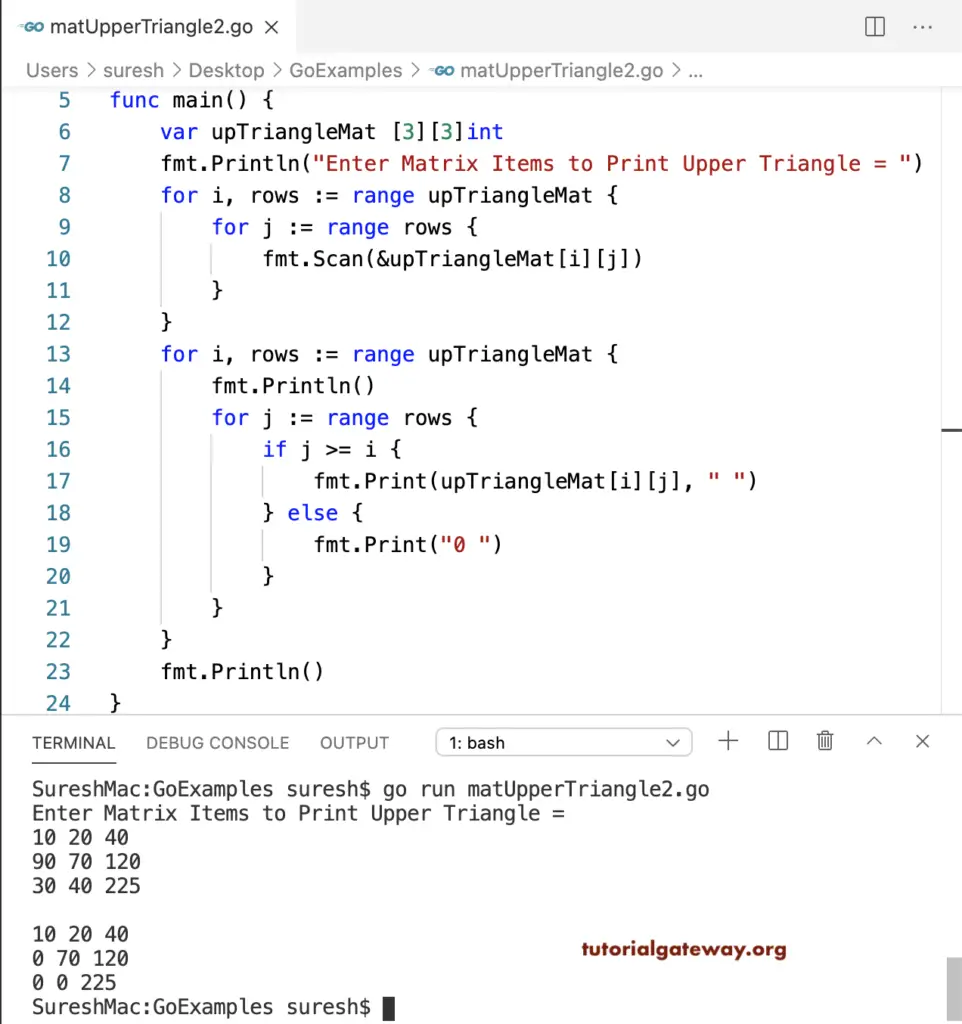