Write a Go Program to Print the Last Character in a String. We use the string index position to access the last character. Here, strLastChar[len(strLastChar) – 1] returns the Last character in a given string strLastChar.
package main import "fmt" func main() { var strLastChar string strLastChar = "Tutorial Gateway" fmt.Println(strLastChar) LastChar := strLastChar[len(strLastChar)-1] fmt.Printf("The Last Character in this String = %c\n", LastChar) }
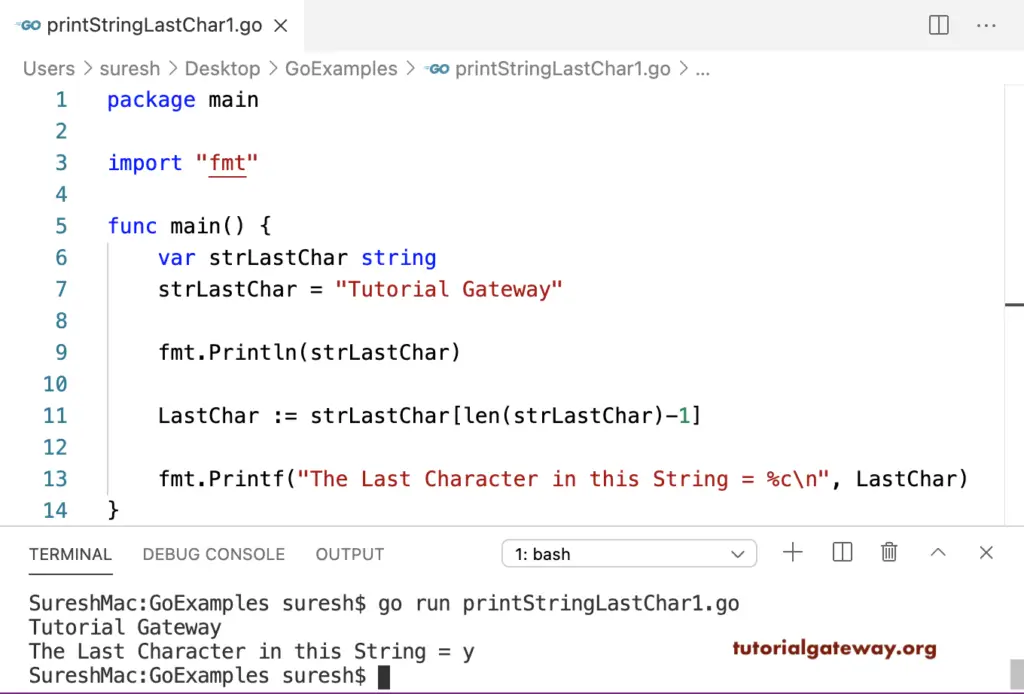
The above string code returns the last byte from the string as an output. However, by slicing the given Golang string, it prints the Last string character.
package main import "fmt" func main() { var strLastChar string strLastChar = "Golang Programs" fmt.Println(strLastChar) LastChar := strLastChar[len(strLastChar)-1:] fmt.Println("The Last Character in this String = ", LastChar) }
Golang Programs
The Last Character in this String = s