Write a Go Program to Print Floyd’s Triangle. In this Floyd’s Triangle example, the first for loop iterates from start to end. The second for loop (for j = 1; j <= i; j++) iterates from 1 to i value. Within the loop, we are printing the number and increment the number.
package main import "fmt" func main() { var i, j, rows int number := 1 fmt.Print("Enter Total Rows to Print Floyd's Triangle = ") fmt.Scanln(&rows) fmt.Println("Floyd's Triangle") for i = 1; i <= rows; i++ { for j = 1; j <= i; j++ { fmt.Printf("%d ", number) number++ } fmt.Println() } }
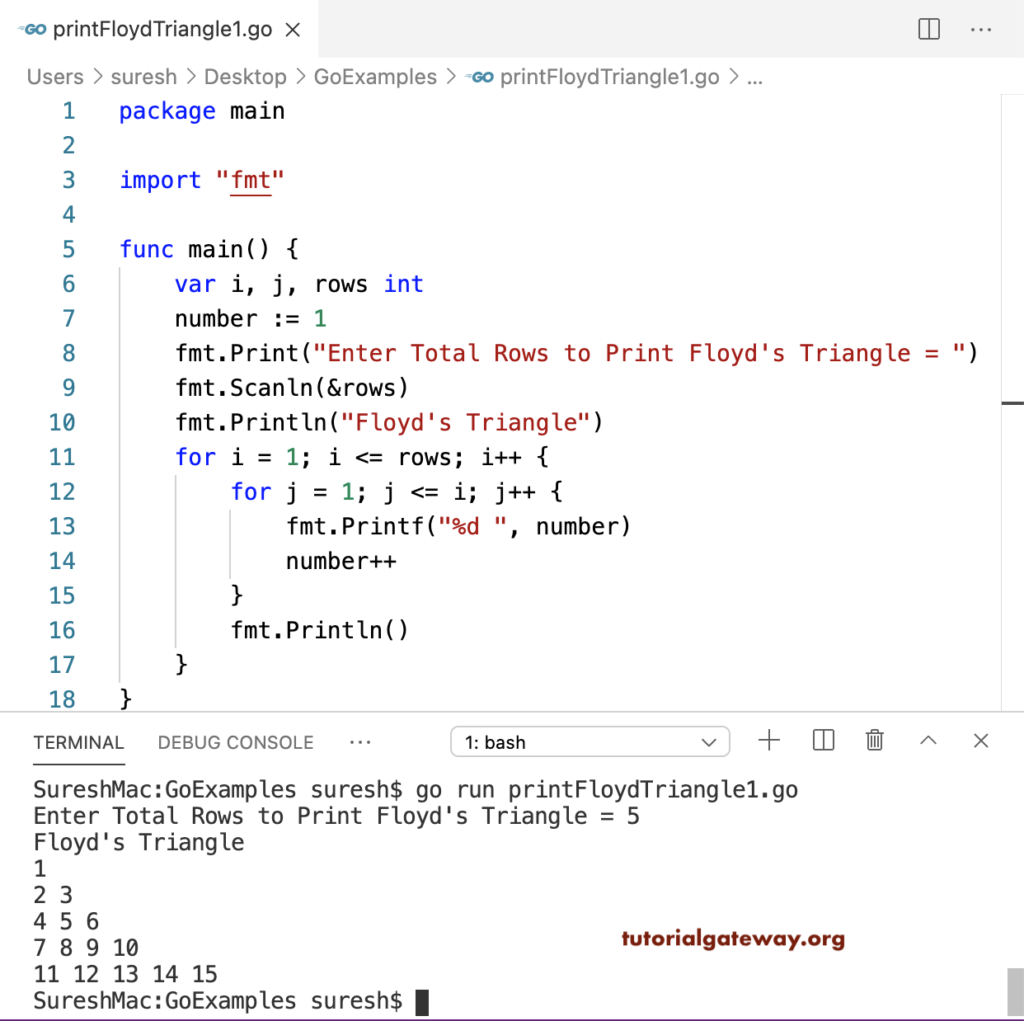
This Golang program allows entering the starting number and prints the Floyd’s Triangle from that number.
package main import "fmt" func main() { var i, j, rows int var number int fmt.Print("Enter Total Rows to Print Floyd's Triangle = ") fmt.Scanln(&rows) fmt.Print("Enter The Starting Number = ") fmt.Scanln(&number) fmt.Println("Floyd's Triangle") for i = 1; i <= rows; i++ { for j = 1; j <= i; j++ { fmt.Printf("%d ", number) number++ } fmt.Println() } }
Enter Total Rows to Print Floyd's Triangle = 7
Enter The Starting Number = 10
Floyd's Triangle
10
11 12
13 14 15
16 17 18 19
20 21 22 23 24
25 26 27 28 29 30
31 32 33 34 35 36 37
In this Go Floyd’s Triangle example, we replaced the number with a star symbol.
package main import "fmt" func main() { var i, j, rows int fmt.Print("Enter Total Rows to Print Floyd's Triangle = ") fmt.Scanln(&rows) fmt.Println("Floyd's Triangle") for i = 1; i <= rows; i++ { for j = 1; j <= i; j++ { fmt.Print("* ") } fmt.Println() } }
Enter Total Rows to Print Floyd's Triangle = 10
Floyd's Triangle
*
* *
* * *
* * * *
* * * * *
* * * * * *
* * * * * * *
* * * * * * * *
* * * * * * * * *
* * * * * * * * * *