Write a Go Program to Print Exponentially Increased Star Pattern. In this Golang Exponentially Increased Star Pattern example, the first for loop iterates from start to end. The second for loop (for j = 1; j <= math.Pow(2, i); j++) iterates from 1 to 2 power i value. Within the loop, we are printing the star.
package main import ( "fmt" "math" ) func main() { var i, j, rows float64 fmt.Print("Enter Total Rows = ") fmt.Scanln(&rows) fmt.Println("Exponentially Increasing Star Pattern") for i = 0; i <= rows; i++ { for j = 1; j <= math.Pow(2, i); j++ { fmt.Print("* ") } fmt.Println() } }
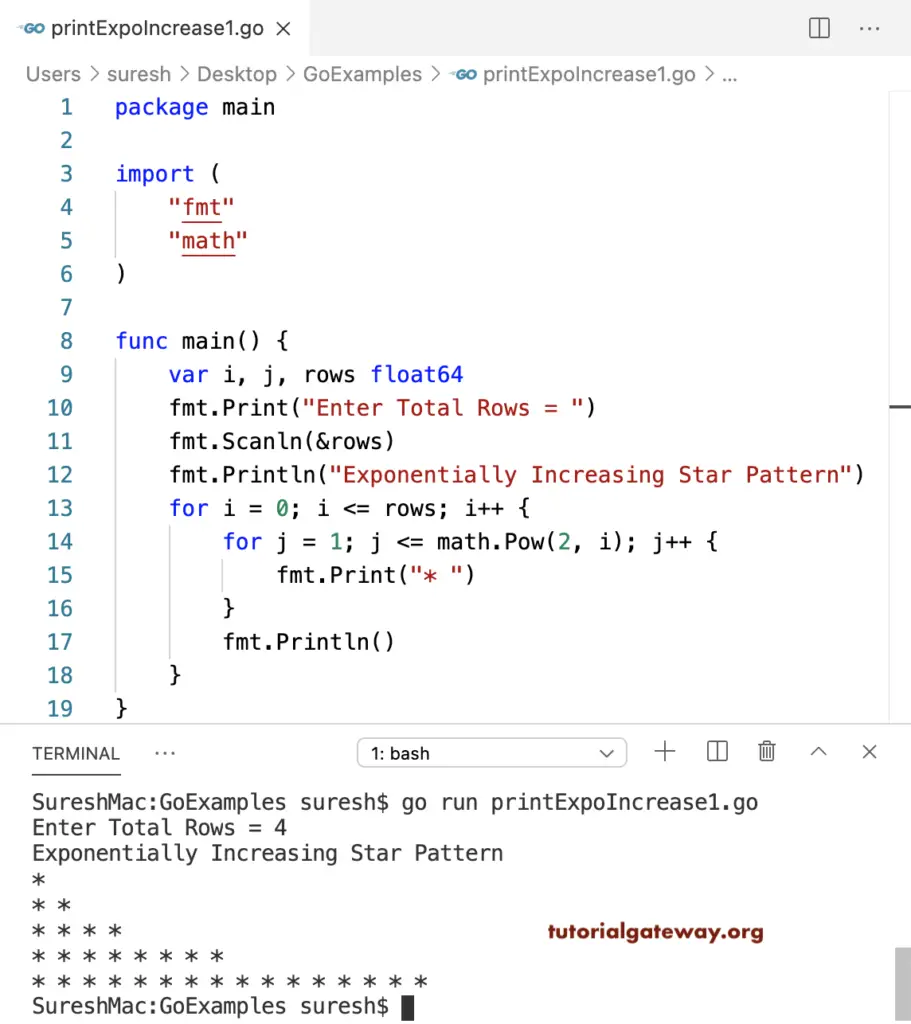
This Golang program allows entering any symbol and prints the exponentially increased pattern of that symbol.
package main import ( "fmt" "math" ) func main() { var i, j, rows float64 var ch string fmt.Print("Enter Total Rows = ") fmt.Scanln(&rows) fmt.Print("Enter The Symbol = ") fmt.Scanln(&ch) fmt.Println("Exponentially Increasing Star Pattern") for i = 0; i <= rows; i++ { for j = 1; j <= math.Pow(2, i); j++ { fmt.Printf("%s ", ch) } fmt.Println() } }
Enter Total Rows = 5
Enter The Symbol = $
Exponentially Increasing Star Pattern
$
$ $
$ $ $ $
$ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $