This Go program to print even numbers from 1 to n uses the for loop that starts at one and ends at evenum. Inside it, the if statement checks whether the remainder of the number divisible by two equals zero. If True, it prints that even number as output.
package main import "fmt" func main() { var evnum int fmt.Print("Enter the Number to Print Even's = ") fmt.Scanln(&evnum) fmt.Println("Even Numbers from 1 to ", evnum, " are = ") for i := 1; i <= evnum; i++ { if i%2 == 0 { fmt.Print(i, "\t") } } fmt.Println() }
Enter the Number to Print Even's = 10
Even Numbers from 1 to 10 are =
2 4 6 8 10
Golang Program to Print Even Numbers from 1 to N
In this Golang program, for loop starts at two and increments by two. It manes, all the numbers will be even because we are skipping the odd numbers.
package main import "fmt" func main() { var evnum, i int fmt.Print("Enter the Number to Print Even's = ") fmt.Scanln(&evnum) fmt.Println("Even Numbers from 1 to ", evnum, " are = ") for i = 2; i <= evnum; i = i + 2 { fmt.Print(i, "\t") } fmt.Println() }
Enter the Number to Print Even's = 20
Even Numbers from 1 to 20 are =
2 4 6 8 10 12 14 16 18 20
It is another way of writing for loops to display even numbers.
package main import "fmt" func main() { var evnum int fmt.Print("Enter the Number to Print Even's = ") fmt.Scanln(&evnum) i := 2 fmt.Println("Even Numbers from 1 to ", evnum, " are = ") for i <= evnum { fmt.Print(i, "\t") i = i + 2 } fmt.Println() }
Enter the Number to Print Even's = 30
Even Numbers from 1 to 30 are =
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30
This Go program returns the even numbers from minimum to maximum. The first if statement checks whether the minimum value is odd, and if it is true, the minimum value incremented by 1. So it becomes an even number. We incremented this value within the for loop by two so that all the numbers will be even. No requirement for an additional if statement for every number.
package main import "fmt" func main() { var min, max int fmt.Print("Enter the Minimum to Start Printing Even's = ") fmt.Scanln(&min) fmt.Print("Enter the Maximum to End Printing Even's = ") fmt.Scanln(&max) if min%2 != 0 { min++ } fmt.Print("The Even Numbers from ", min, " to ", max, " are \n") for i := min; i <= max; i = i + 2 { fmt.Print(i, "\t") } fmt.Println() }
Enter the Minimum to Start Printing Even's = 10
Enter the Maximum to End Printing Even's = 40
The Even Numbers from 10 to 40 are
10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40
Go Program to Print Even Numbers using Functions. This Golang example is the same as the first Even Numbers example. However, it prints the even numbers among the minimum and maximum limit value.
package main import "fmt" func main() { var min, max int fmt.Print("Enter the Minimum to Start Printing Even's = ") fmt.Scanln(&min) fmt.Print("Enter the Maximum to End Printing Even's = ") fmt.Scanln(&max) fmt.Print("The Even Numbers from ", min, " to ", max, " are \n") for i := min; i <= max; i++ { if i%2 == 0 { fmt.Print(i, "\t") } } }
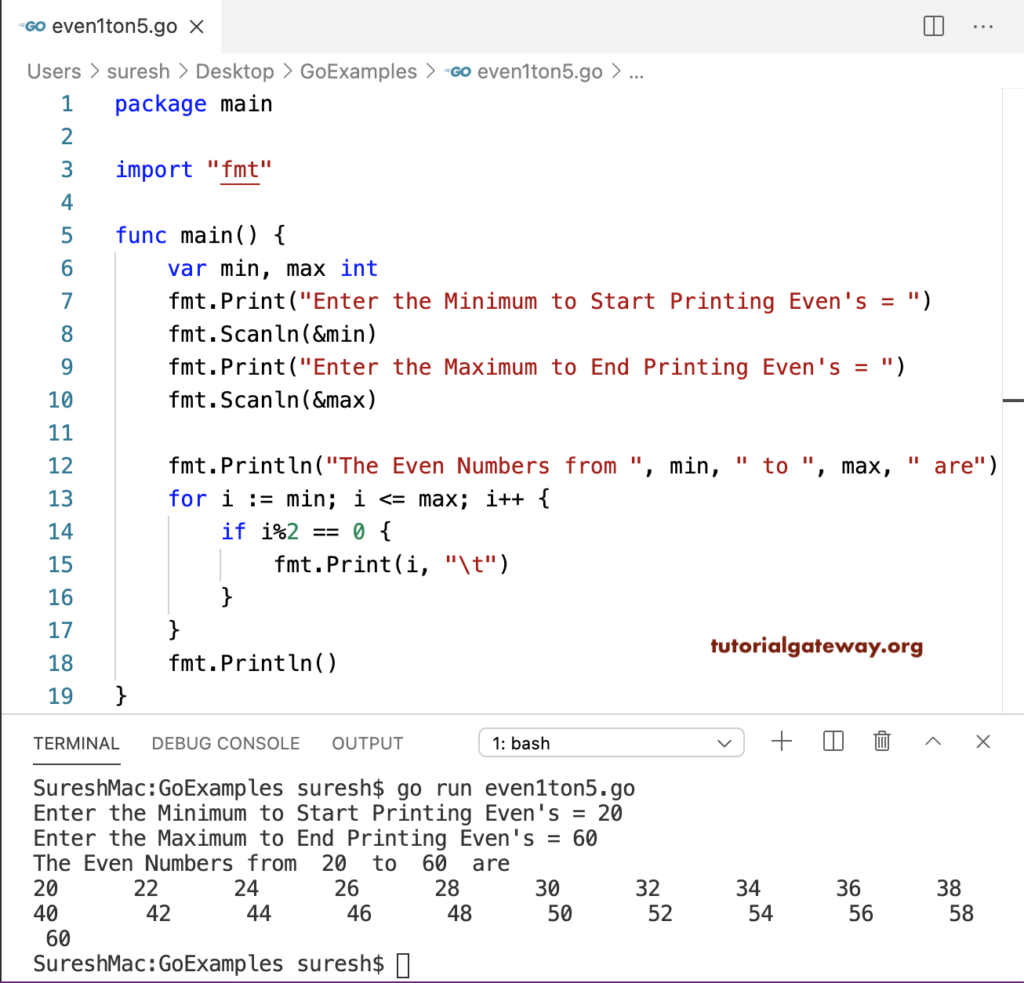