Write a Go program to Print the Array items in the odd index position (not the actual odd position) using For loop. Here, the for loop (for i := 1; i < len(odarray); i += 2) starts iteration at one and increments by two up to an array length. Within the loop, we print all the array items at an odd position.
package main import "fmt" func main() { odarray := []int{11, 12, 32, 45, 65, 90, 70, 80} fmt.Println("The List of Array Items in Odd Index Position = ") for i := 1; i < len(odarray); i += 2 { fmt.Println(odarray[i]) } }
The List of Array Items in Odd Index Position =
12
45
90
80
Golang Program to Print Array Items in Odd Index Position using the For Loop range
We used an extra if statement (if i%2 != 0) to check whether the index position divisible by two not equals to zero, which means it is an odd index position. Next, print that number.
package main import "fmt" func main() { odarray := []int{11, 12, 32, 45, 65, 90, 70, 80} fmt.Println("The List of Array Items in Odd Index Position = ") for i, _ := range odarray { if i%2 != 0 { fmt.Println(odarray[i]) } } }
The List of Array Items in Odd Index Position =
12
45
90
80
This Golang program allows entering the array size and items and pints the elements at an odd index position.
package main import "fmt" func main() { var size int fmt.Print("Enter the Even Odd Array Size = ") fmt.Scan(&size) odarray := make([]int, size) fmt.Print("Enter the Even Odd Array Items = ") for i := 0; i < size; i++ { fmt.Scan(&odarray[i]) } fmt.Println("The List of Array Items in Odd index Position = ") for i := 1; i < len(odarray); i += 2 { fmt.Println(odarray[i]) } }
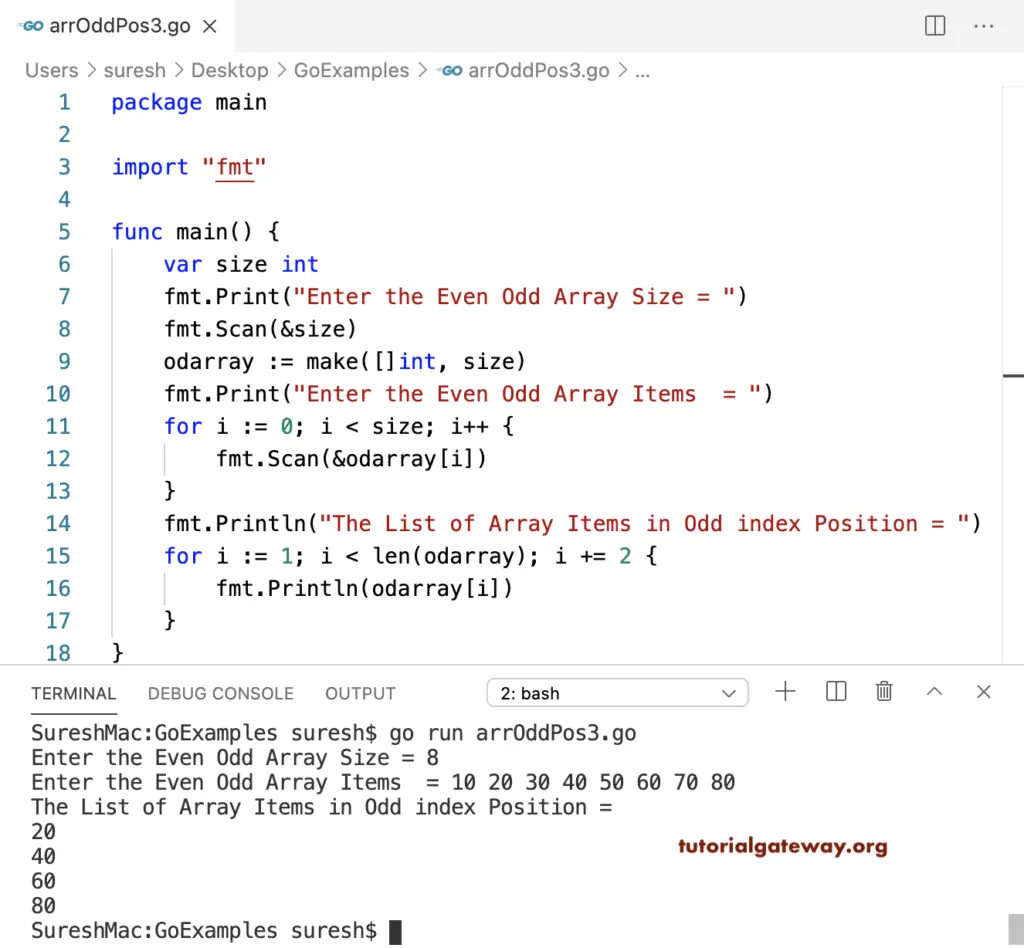