Write a Go Program to Print 1 and 0 in Alternative Rows. In this Golang example, the nested for loop iterates user-entered rows and columns. Within the loop, the if statement (if i%2 != 0) checks whether row percentage two not equals zero. If true, print 1’s in that row; otherwise, print 0’s as row values.
package main import "fmt" func main() { var i, j, row, col int fmt.Print("Enter the Rows = ") fmt.Scanln(&row) fmt.Print("Enter the Columns = ") fmt.Scanln(&col) fmt.Println("Print 1, 0 as Alternative Rows") for i = 1; i <= row; i++ { for j = 1; j <= col; j++ { if i%2 != 0 { fmt.Print("1") } else { fmt.Print("0") } } fmt.Println() } }
Enter the Rows = 5
Enter the Columns = 10
Print 1, 0 as Alternative Rows
1111111111
0000000000
1111111111
0000000000
1111111111
In this Golang program, we removed the extra if statement to Print 1 and 0 in Alternative Rows.
package main import "fmt" func main() { var i, j, row, col int fmt.Print("Enter the Rows = ") fmt.Scanln(&row) fmt.Print("Enter the Columns = ") fmt.Scanln(&col) fmt.Println("Print 1, 0 as Alternative Rows") for i = 1; i <= row; i++ { for j = 1; j <= col; j++ { fmt.Printf("%d", i%2) } fmt.Println() } }
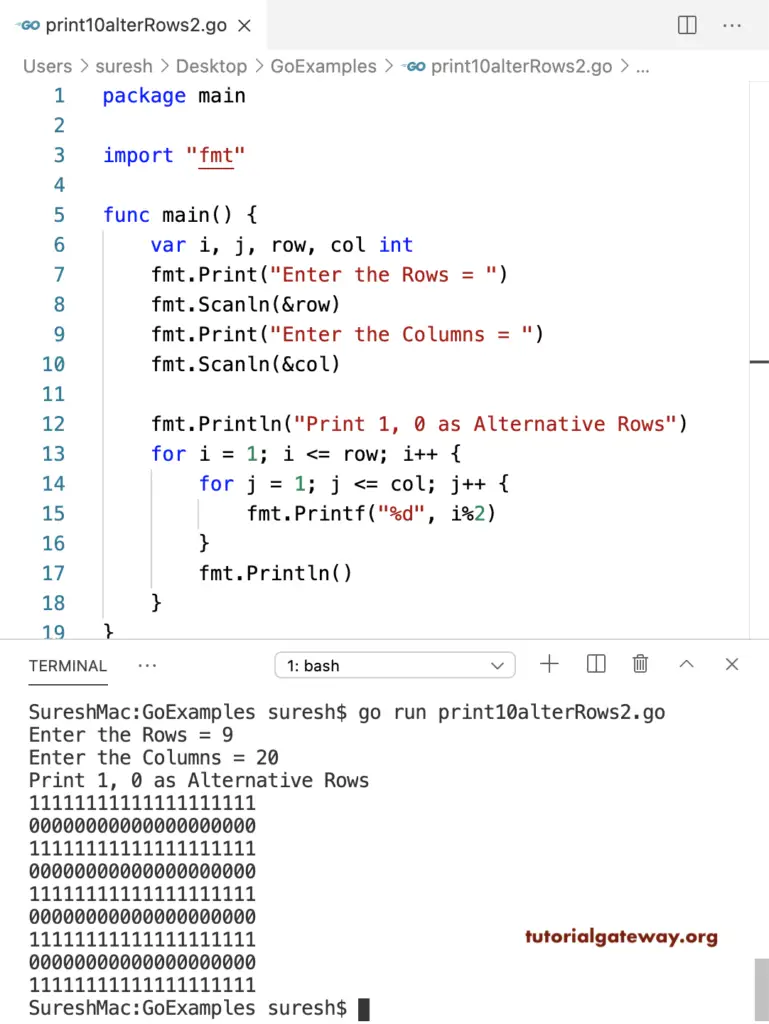
Go Program to Print 0 and 1 in Alternative Rows
We interchanged the 0 and 1 rows values within the If else statement.
package main import "fmt" func main() { var i, j, row, col int fmt.Print("Enter the Rows = ") fmt.Scanln(&row) fmt.Print("Enter the Columns = ") fmt.Scanln(&col) fmt.Println("Print 0, 1 as Alternative Rows") for i = 1; i <= row; i++ { for j = 1; j <= col; j++ { if i%2 != 0 { fmt.Print("0") } else { fmt.Print("1") } } fmt.Println() } }
Enter the Rows = 9
Enter the Columns = 30
Print 0, 1 as Alternative Rows
000000000000000000000000000000
111111111111111111111111111111
000000000000000000000000000000
111111111111111111111111111111
000000000000000000000000000000
111111111111111111111111111111
000000000000000000000000000000
111111111111111111111111111111
000000000000000000000000000000