Write a Go Program to Print 1 and 0 in Alternative Columns. In this Golang example, we used nested for loop to iterate user-entered rows and columns. Within the loop, the if statement (if j%2 == 0) checks whether the column is even. If true, print 0’s in that column; otherwise, print 1’s as column value.
package main import "fmt" func main() { var i, j, row, col int fmt.Print("Enter the Rows and Columns = ") fmt.Scanln(&row, &col) fmt.Println("Print 1, 0 as Alternative Columns") for i = 1; i <= row; i++ { for j = 1; j <= col; j++ { if j%2 == 0 { fmt.Print("0") } else { fmt.Print("1") } } fmt.Println() } }
Enter the Rows and Columns = 7 10
Print 1, 0 as Alternative Columns
1010101010
1010101010
1010101010
1010101010
1010101010
1010101010
1010101010
In this Golang program, we removed extra if Statement to Print 1 and 0 in Alternative Columns.
package main import "fmt" func main() { var i, j, row, col int fmt.Print("Enter the Rows and Columns = ") fmt.Scanln(&row, &col) fmt.Println("Print 1, 0 as Alternative Columns") for i = 1; i <= row; i++ { for j = 1; j <= col; j++ { fmt.Printf("%d", j%2) } fmt.Println() } }
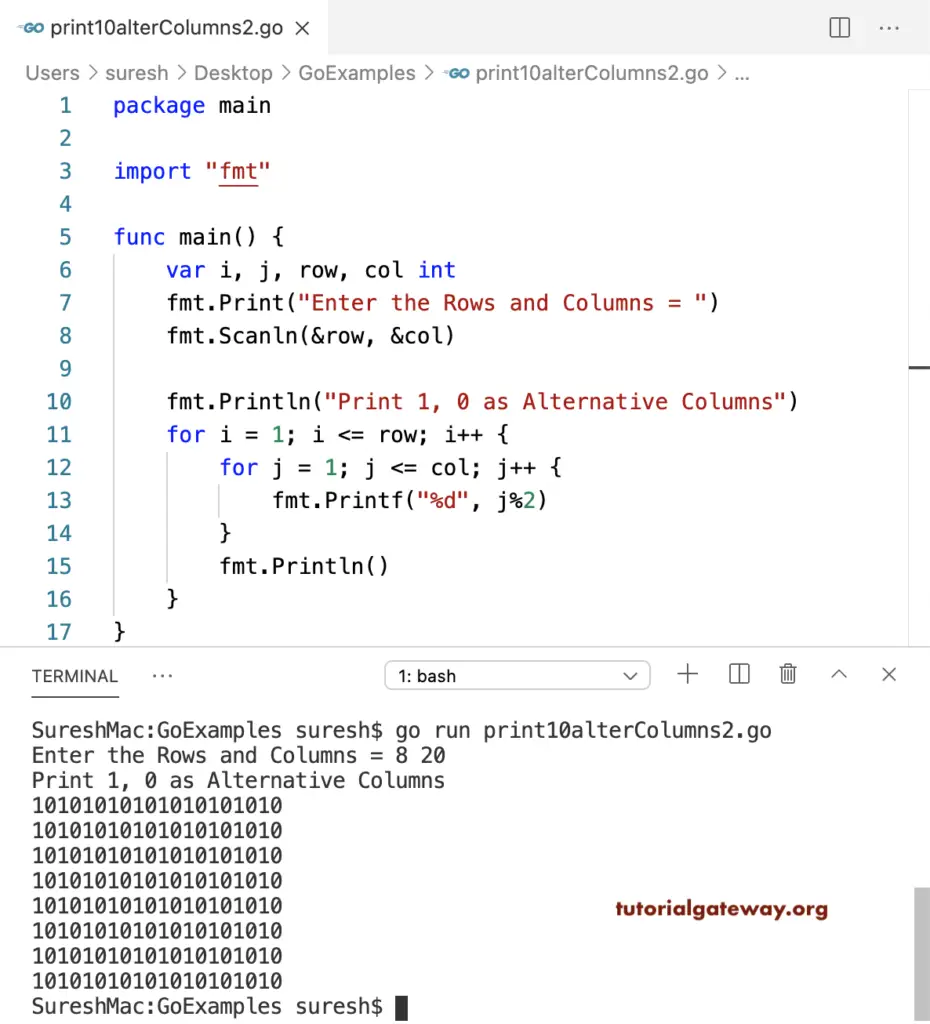
Go Program to Print 0 and 1 in Alternative Columns
We interchanged the 0 and 1 values within the If else statement.
package main import "fmt" func main() { var i, j, row, col int fmt.Print("Enter the Rows and Columns = ") fmt.Scanln(&row, &col) fmt.Println("Print 0, 1 as Alternative Columns") for i = 1; i <= row; i++ { for j = 1; j <= col; j++ { if j%2 == 0 { fmt.Print("1") } else { fmt.Print("0") } } fmt.Println() } }
Enter the Rows and Columns = 8 25
Print 0, 1 as Alternative Columns
0101010101010101010101010
0101010101010101010101010
0101010101010101010101010
0101010101010101010101010
0101010101010101010101010
0101010101010101010101010
0101010101010101010101010
0101010101010101010101010