Write a Go Program to interchange the diagonal items in a Matrix. First, it (if rows == columns) checks whether the given Matrix is square. Next, we used the temp variable to interchange the Diagonal items. The last for loop prints the Matrix after interchanging the diagonals.
package main import "fmt" func main() { var i, j, rows, columns, temp int var digInterMat [10][10]int fmt.Print("Enter the Matrix rows and Columns = ") fmt.Scan(&rows, &columns) fmt.Println("Enter Matrix Items to Interchange diagonals = ") for i = 0; i < rows; i++ { for j = 0; j < columns; j++ { fmt.Scan(&digInterMat[i][j]) } } if rows == columns { for i = 0; i < rows; i++ { temp = digInterMat[i][i] digInterMat[i][i] = digInterMat[i][rows-i-1] digInterMat[i][rows-i-1] = temp } fmt.Println("*** The Matrix Items after Interchanging Diagonals ***") for i = 0; i < rows; i++ { for j = 0; j < columns; j++ { fmt.Print(digInterMat[i][j], " ") } fmt.Println() } } else { fmt.Println("The Given Matrix is Not a Square Matrix") } }
Enter the Matrix rows and Columns = 2 2
Enter Matrix Items to Interchange diagonals =
1 2
9 8
*** The Matrix Items after Interchanging Diagonals ***
2 1
8 9
Golang Program to Interchange Matrix Diagonals using the For loop range
We removed the extra If else to check for square Matrix because we initialized the Matrix as 3 * 3.
package main import "fmt" func main() { var temp int var digInterMat [3][3]int fmt.Println("Enter Matrix Items to Interchange diagonals = ") for i, rows := range digInterMat { for j := range rows { fmt.Scan(&digInterMat[i][j]) } } for i := range digInterMat { temp = digInterMat[i][i] digInterMat[i][i] = digInterMat[i][3-i-1] //rows = 3 digInterMat[i][3-i-1] = temp } fmt.Println("*** The Matrix Items after Interchanging Diagonals ***") for i, rows := range digInterMat { for j := range rows { fmt.Print(digInterMat[i][j], " ") } fmt.Println() } }
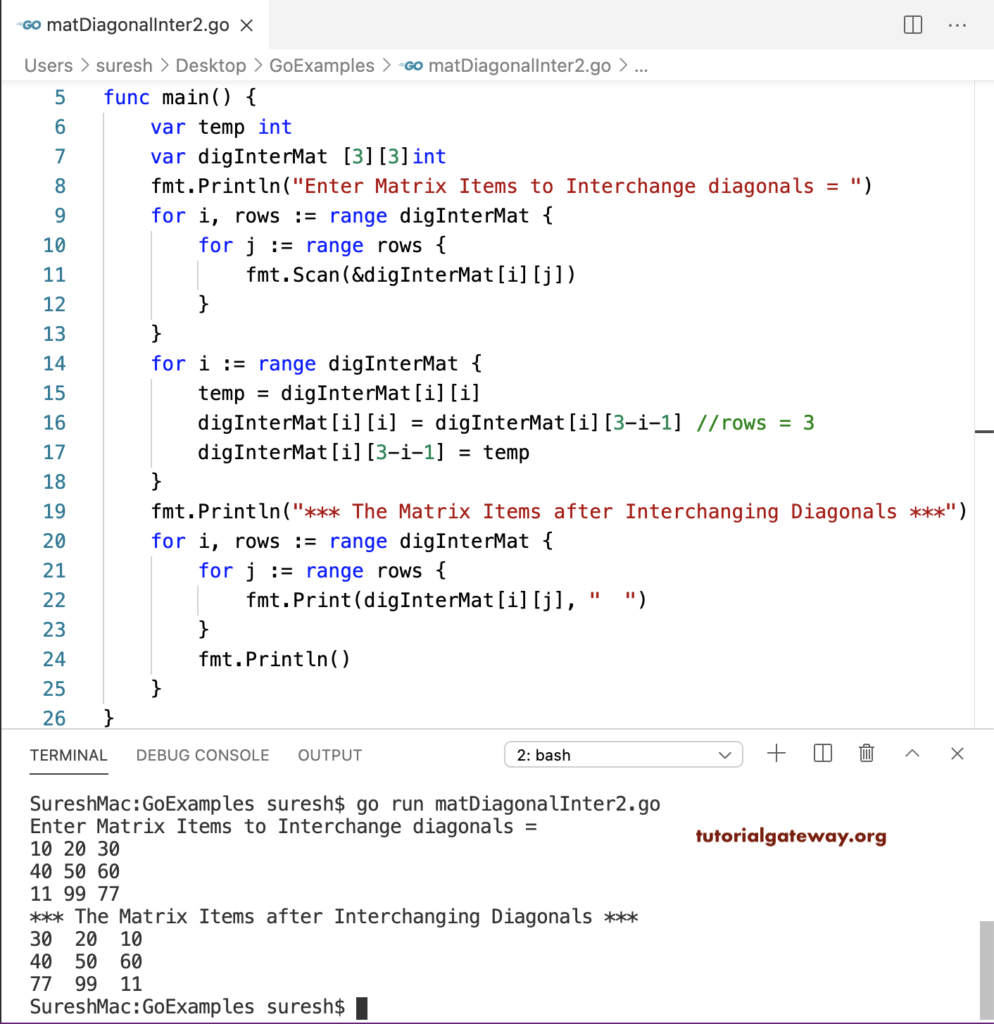