Write a Go program to Find Volume and Surface Area of a Cuboid. The math formula to calculate the cuboid volume, surface area, and lateral surface area are
- Total Surface Area of a Cuboid = 2lw + 2lh + 2wh, where l = length, w = width, and h = height
- Volume of a Cuboid = lbh
- The Lateral Surface Area of a Cuboid = 2h (l + w)
package main import "fmt" func main() { var cublen, cubWidth, cubHeight, cubSA, cubVolume, cubLA float32 fmt.Print("Enter the Length of a Cuboid = ") fmt.Scanln(&cublen) fmt.Print("Enter the Width of a Cuboid = ") fmt.Scanln(&cubWidth) fmt.Print("Enter the Height of a Cuboid = ") fmt.Scanln(&cubHeight) cubSA = 2 * (cublen*cubWidth + cublen*cubHeight + cubWidth*cubHeight) cubVolume = cublen * cubWidth * cubHeight cubLA = 2 * cubHeight * (cublen + cubWidth) fmt.Println("\nThe Volume of a Cuboid = ", cubVolume) fmt.Println("The Surface Area of a Cuboid = ", cubSA) fmt.Println("Lateral Surface Area of a Cuboid = ", cubLA) }
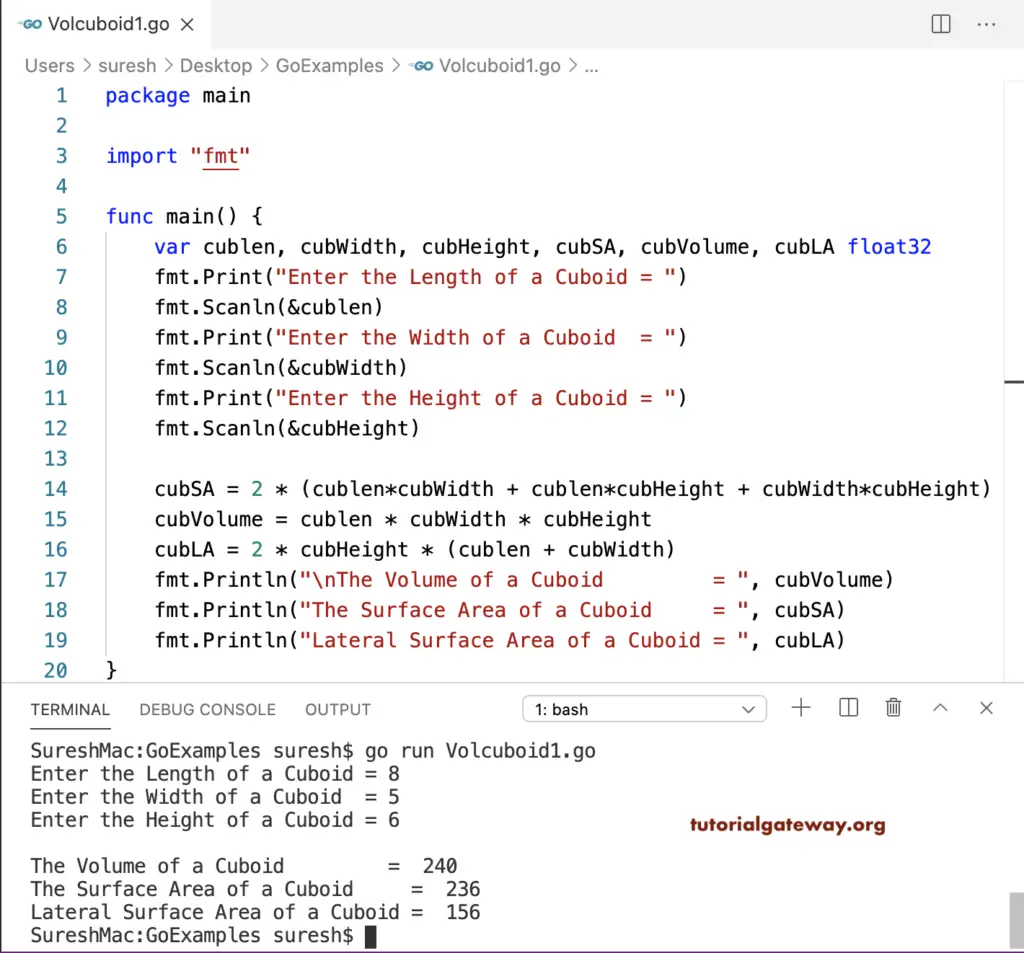