This Go program to find the Sum of Odd Numbers from 1 to n uses the for loop to iterate between 1 and n. Inside the loop, if statement filters the odd numbers and adds total to odd total.
package main import "fmt" func main() { var oddnum, Oddtotal int fmt.Print("Enter the Number to find Odd Sum = ") fmt.Scanln(&oddnum) Oddtotal = 0 fmt.Println("List of Odd Numbers from 1 to ", oddnum, " are = ") for x := 1; x <= oddnum; x++ { if x%2 != 0 { fmt.Print(x, "\t") Oddtotal = Oddtotal + x } } fmt.Println("\nSum of Odd Numbers from 1 to ", oddnum, " = ", Oddtotal) }
Enter the Number to find Odd Sum = 10
List of Odd Numbers from 1 to 10 are =
1 3 5 7 9
Sum of Odd Numbers from 1 to 10 = 25
Golang Program to find Sum of Odd Numbers.
In this Golang program, we altered the for loop to start from 1 and increment by 2 to calculate the sum of odd numbers.
package main import "fmt" func main() { var oddnum, Oddtotal int fmt.Print("Enter the Number to find Odd Sum = ") fmt.Scanln(&oddnum) Oddtotal = 0 fmt.Println("List of Odd Numbers from 1 to ", oddnum, " are = ") for x := 1; x <= oddnum; x = x + 2 { fmt.Print(x, "\t") Oddtotal = Oddtotal + x } fmt.Println("\nSum of Odd Numbers from 1 to ", oddnum, " = ", Oddtotal) }
Enter the Number to find Odd Sum = 25
List of Odd Numbers from 1 to 25 are =
1 3 5 7 9 11 13 15 17 19 21 23 25
Sum of Odd Numbers from 1 to 25 = 169
This Go program calculates the sum of odd numbers from min to max. Here, we used an extra if statement to hold the user-entered value as odd by increment the even value (if any).
package main import "fmt" func main() { var Oddmin, Oddmax, Oddtotal int fmt.Print("Enter the Minimum Odd Number = ") fmt.Scanln(&Oddmin) fmt.Print("Enter the Maximum Odd Number = ") fmt.Scanln(&Oddmax) if Oddmin%2 == 0 { Oddmin++ } Oddtotal = 0 fmt.Println("Odd Numbers from ", Oddmin, " to ", Oddmax, " = ") for x := Oddmin; x <= Oddmax; x = x + 2 { fmt.Print(x, "\t") Oddtotal = Oddtotal + x } fmt.Println("\nSum of Odd Numbers from ", Oddmin, " to ", Oddmax, " = ", Oddtotal) }
Enter the Minimum Odd Number = 10
Enter the Maximum Odd Number = 50
Odd Numbers from 11 to 50 =
11 13 15 17 19 21 23 25 27 29 31 33 35 37 39 41 43 45 47 49
Sum of Odd Numbers from 11 to 50 = 600
It is identical to the first sum of odd Numbers example. However, it calculates the odd sum between the minimum and maximum limit.
package main import "fmt" func main() { var Oddmin, Oddmax, Oddtotal int fmt.Print("Enter the Minimum Odd Number = ") fmt.Scanln(&Oddmin) fmt.Print("Enter the Maximum Odd Number = ") fmt.Scanln(&Oddmax) Oddtotal = 0 fmt.Println("Odd Numbers from ", Oddmin, " to ", Oddmax, " = ") for x := Oddmin; x <= Oddmax; x++ { if x%2 != 0 { fmt.Print(x, "\t") Oddtotal = Oddtotal + x } } fmt.Println("\nSum of Odd Numbers from ", Oddmin, " to ", Oddmax, " = ", Oddtotal) }
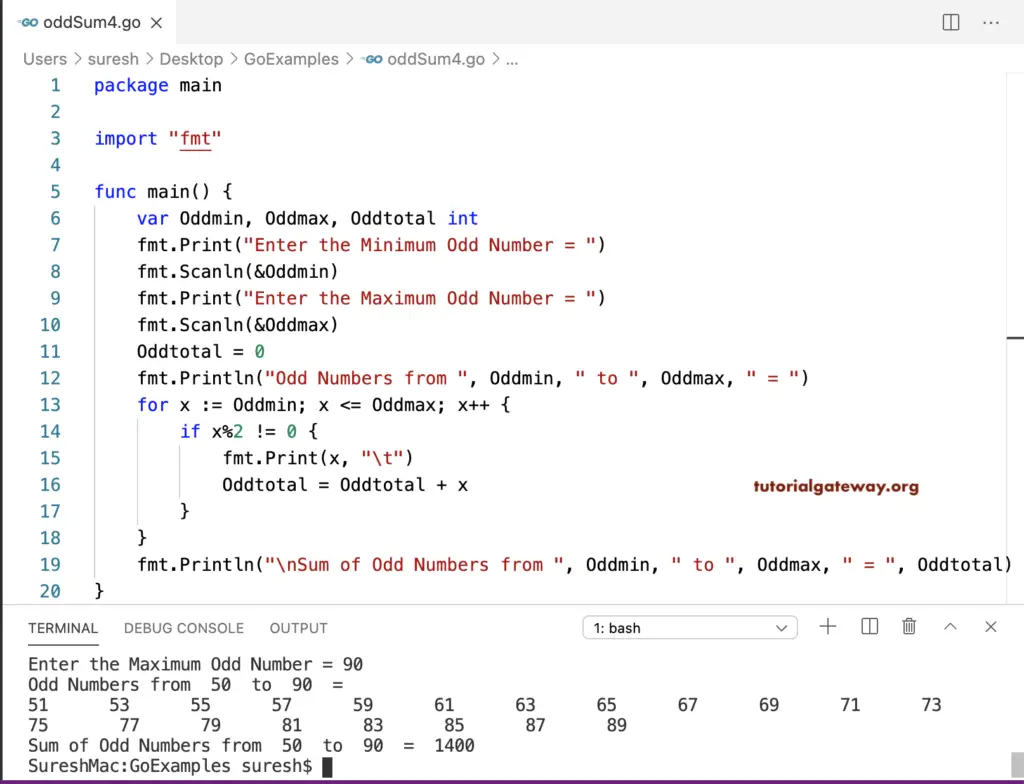