Write a Go Program to Find the Sum of the opposite diagonal items in a Matrix. In this Go Matrix Opposite Diagonal example, we use for loop to iterate the Matrix row items and calculates (oppDiagsum = oppDiagsum + oppDiSumMat[i][rows-i-1]) the sum of opposite diagonal items.
package main import "fmt" func main() { var i, j, rows, columns int var oppDiSumMat [10][10]int fmt.Print("Enter the Matrix rows and Columns = ") fmt.Scan(&rows, &columns) fmt.Println("Enter Matrix Items to find Opposite Diagonal Sum = ") for i = 0; i < rows; i++ { for j = 0; j < columns; j++ { fmt.Scan(&oppDiSumMat[i][j]) } } oppDiagsum := 0 for i = 0; i < rows; i++ { oppDiagsum = oppDiagsum + oppDiSumMat[i][rows-i-1] } fmt.Println("The Sum of Matrix Diagonal Elements = ", oppDiagsum) }
Enter the Matrix rows and Columns = 2 2
Enter Matrix Items to find Opposite Diagonal Sum =
10 20
30 40
The Sum of Matrix Diagonal Elements = 50
Golang Program to Find the Sum of the Opposite Diagonal elements in a Matrix using For loop range.
package main import "fmt" func main() { var oppDiSumMat [3][3]int fmt.Println("Enter Matrix Items to find Opposite Diagonal Sum = ") for i, rows := range oppDiSumMat { for j := range rows { fmt.Scan(&oppDiSumMat[i][j]) } } oppDiagsum := 0 for k := range oppDiSumMat { oppDiagsum = oppDiagsum + oppDiSumMat[k][3-k-1] } fmt.Println("The Sum of Matrix Diagonal Elements = ", oppDiagsum) }
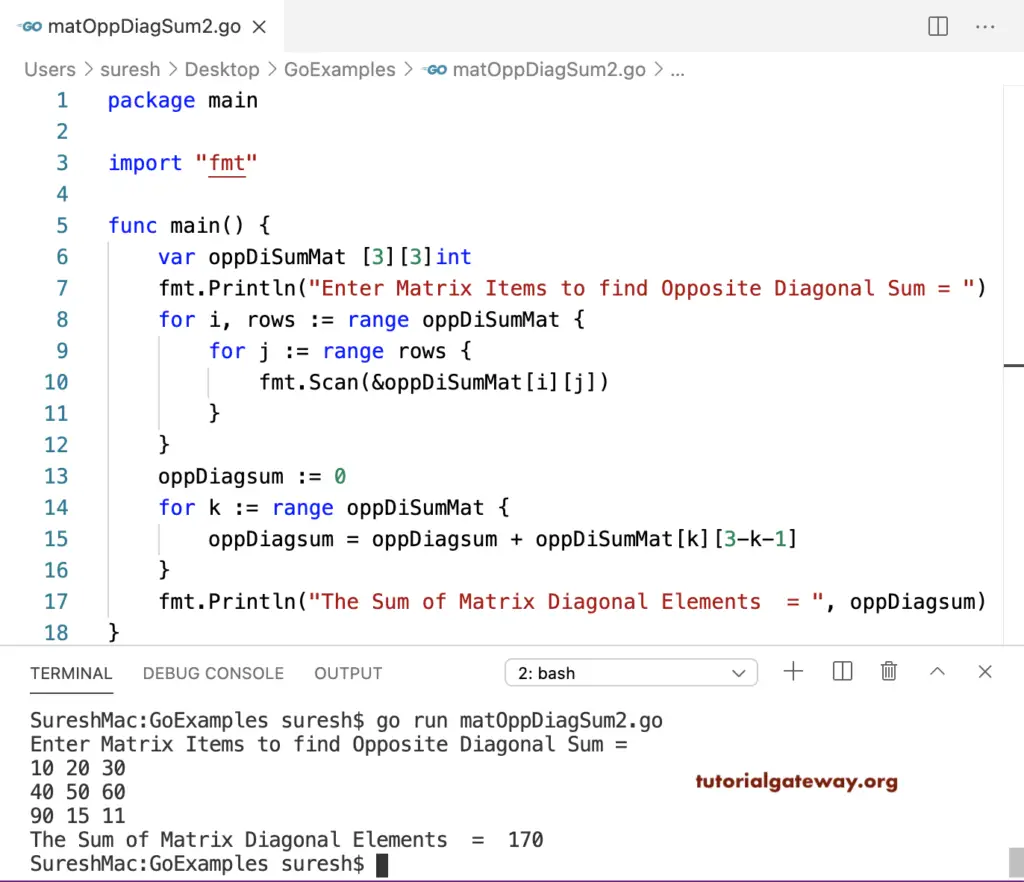