This Go program to find Sum of Even and Odd Numbers from 1 to n uses If else to check whether it is even or not. The for loop helps the GC to iterate between 1 and n. If a condition results in True, the number added to an even total. Otherwise, it adds to the odd total.
package main import "fmt" func main() { var eonum, eventotal, oddtotal int fmt.Print("Enter the Number to find Even and Odd Sum = ") fmt.Scanln(&eonum) eventotal = 0 oddtotal = 0 for x := 1; x <= eonum; x++ { if x%2 == 0 { eventotal = eventotal + x } else { oddtotal = oddtotal + x } } fmt.Println("\nSum of Even Numbers from 1 to ", eonum, " = ", eventotal) fmt.Println("\nSum of Odd Numbers from 1 to ", eonum, " = ", oddtotal) }
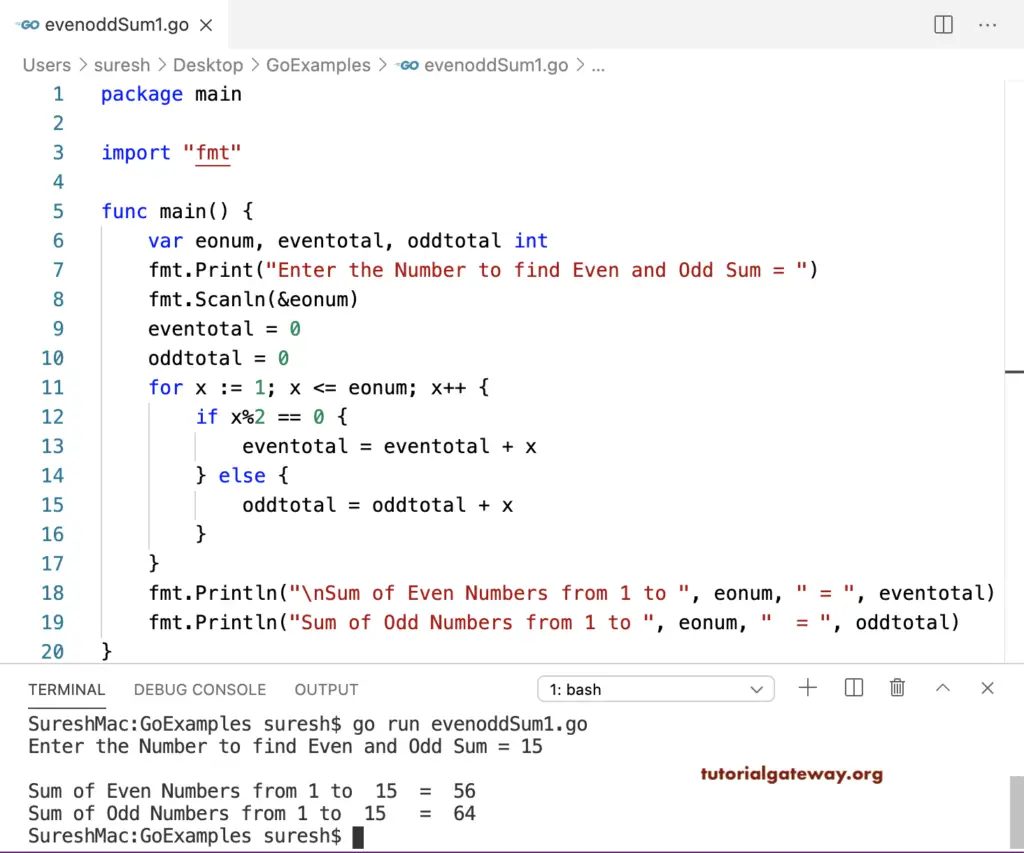
Golang Program to find Sum of Even and Odd Numbers.
This Golang program accepts min to max and calculates the sum of even and odd numbers between the minimum and maximum limit.
package main import "fmt" func main() { var minnum, maxnum, eventotal, oddtotal, x int fmt.Print("Enter the Minimum Number to find Even and Odd Sum = ") fmt.Scanln(&minnum) fmt.Print("Enter the Maximum Number to find Even and Odd Sum = ") fmt.Scanln(&maxnum) eventotal = 0 oddtotal = 0 for x <= maxnum { if x%2 == 0 { eventotal = eventotal + x } else { oddtotal = oddtotal + x } x++ } fmt.Println("\nSum of Even Numbers = ", eventotal) fmt.Println("Sum of Odd Numbers = ", oddtotal) }
Enter the Minimum Number to find Even and Odd Sum = 20
Enter the Maximum Number to find Even and Odd Sum = 50
Sum of Even Numbers = 650
Sum of Odd Numbers = 625
Go Program to calculate the Sum of Even and Odd Numbers using Function. Here, we declared a function that returns both the even and odd sum.
package main import "fmt" func evenOddSum(minnum int, maxnum int) (int, int) { var x, eventotal, oddtotal int eventotal = 0 oddtotal = 0 for x = minnum; x <= maxnum; x++ { if x%2 == 0 { eventotal = eventotal + x } else { oddtotal = oddtotal + x } } return eventotal, oddtotal } func main() { var minnum, maxnum, eventotal, oddtotal int fmt.Print("Enter the Minimum Number to find Even and Odd Sum = ") fmt.Scanln(&minnum) fmt.Print("Enter the Maximum Number to find Even and Odd Sum = ") fmt.Scanln(&maxnum) eventotal, oddtotal = evenOddSum(minnum, maxnum) fmt.Println("\nSum of Even Numbers = ", eventotal) fmt.Println("Sum of Odd Numbers = ", oddtotal) }
Enter the Minimum Number to find Even and Odd Sum = 10
Enter the Maximum Number to find Even and Odd Sum = 70
Sum of Even Numbers = 1240
Sum of Odd Numbers = 1200