Write a Go Program to Find the Sum of Each Matrix Row. It uses the nested for loop and finds the sum of each row in a given matrix.
package main import "fmt" func main() { var i, j, rows, columns int var rowSumMat [10][10]int fmt.Print("Enter the Matrix rows and Columns = ") fmt.Scan(&rows, &columns) fmt.Println("Enter the Matrix Items to find the Row Sum = ") for i = 0; i < rows; i++ { for j = 0; j < columns; j++ { fmt.Scan(&rowSumMat[i][j]) } } for i = 0; i < rows; i++ { sum := 0 for j = 0; j < columns; j++ { sum = sum + rowSumMat[i][j] } fmt.Println("The Sum of Matrix ", i+1, " Row Elements = ", sum) } }
Enter the Matrix rows and Columns =
2 2
Enter the Matrix Items to find the Row Sum =
10 20
30 80
The Sum of Matrix 1 Row Elements = 30
The Sum of Matrix 2 Row Elements = 110
Golang Program to Find the Sum of Each Row in a Matrix using For loop range
package main import "fmt" func main() { var rowSumMat [3][3]int fmt.Println("Enter the Matrix Items to find the Row Sum = ") for i, rows := range rowSumMat { for j := range rows { fmt.Scan(&rowSumMat[i][j]) } } for i, rows := range rowSumMat { sum := 0 for j := range rows { sum = sum + rowSumMat[i][j] } fmt.Println("The Sum of Matrix ", i+1, " Row Elements = ", sum) } }
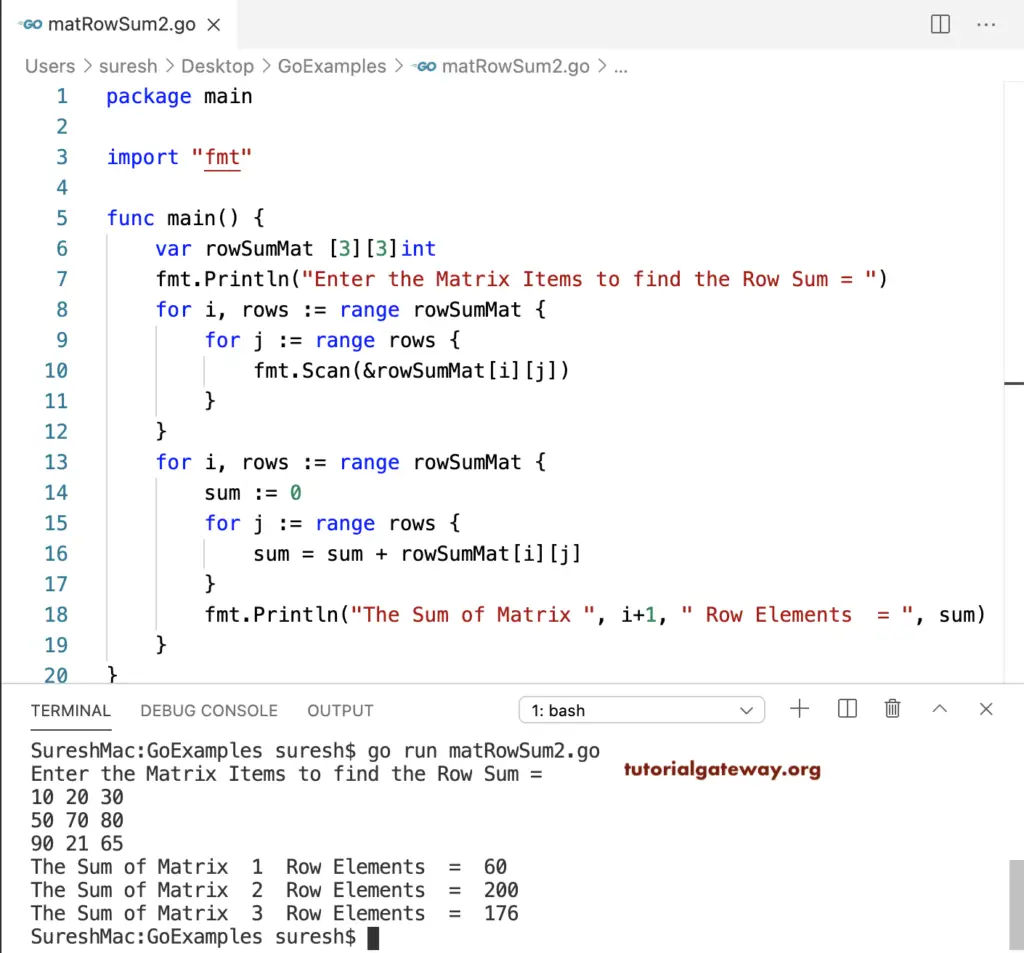