Write a Go Program to Find Product of Digits in a Number using for loop. The for loop condition keeps the number is greater than zero. Within the loop,
- prodReminder = prodNum % 10 -> returns the last digit from a number.
- product = product * prodReminder -> Multiplies the last digit with product.
- prodNum = prodNum / 10 – It removes the last digit from the prodNum.
package main import "fmt" func main() { var prodNum, product, prodReminder int fmt.Print("Enter the Number to find the Digits Product = ") fmt.Scanln(&prodNum) for product = 1; prodNum > 0; prodNum = prodNum / 10 { prodReminder = prodNum % 10 product = product * prodReminder } fmt.Println("The Product of Digits in this Number = ", product) }
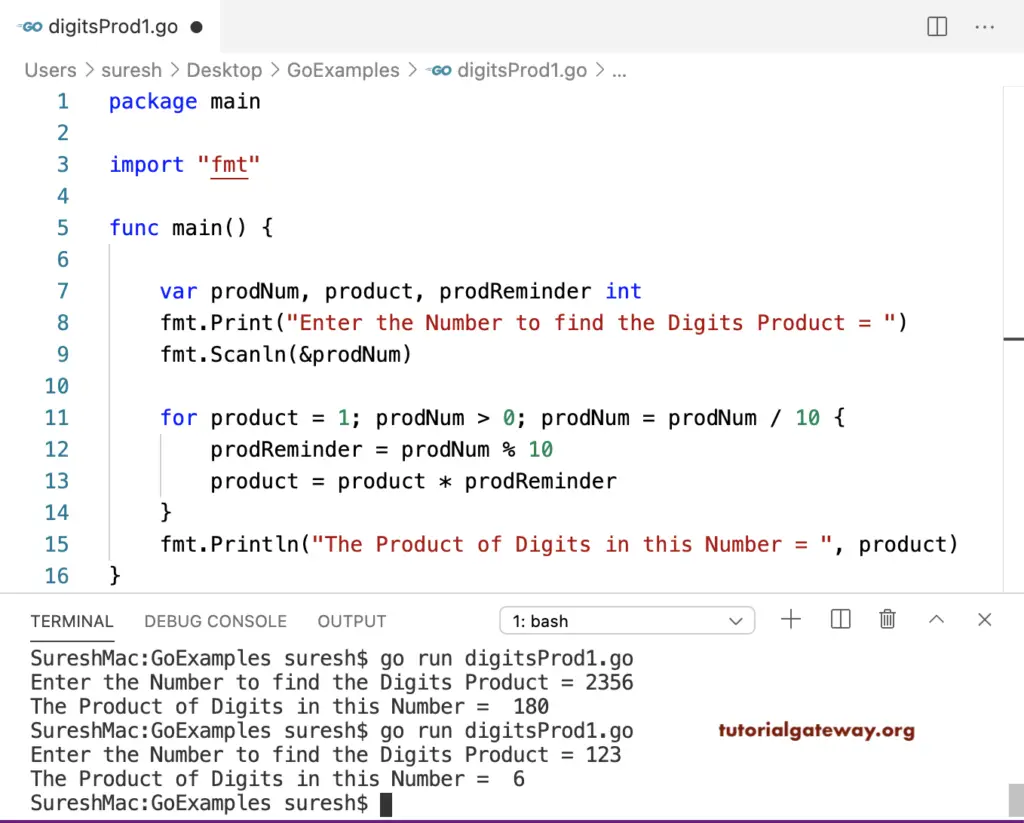
Go Program to find the product of digits in a number using a function
package main import "fmt" var product int func digitsProduct(prodNum int) int { var prodReminder int for product = 1; prodNum > 0; prodNum = prodNum / 10 { prodReminder = prodNum % 10 product = product * prodReminder } return product } func main() { var prodNum int fmt.Print("Enter the Number to find the Digits Product = ") fmt.Scanln(&prodNum) product = digitsProduct(prodNum) fmt.Println("The Product of Digits in this Number = ", product) }
SureshMac:GoExamples suresh$ go run digitsProd2.go
Enter the Number to find the Digits Product = 879
The Product of Digits in this Number = 504
SureshMac:GoExamples suresh$ go run digitsProd2.go
Enter the Number to find the Digits Product = 45
The Product of Digits in this Number = 20
This Golang program call digitsProduct(prodNum / 10) function recursively to calculate Digits’ product in a Number.
package main import "fmt" var product int = 1 func digitsProduct(prodNum int) int { if prodNum <= 0 { return 0 } product = product * (prodNum % 10) digitsProduct(prodNum / 10) return product } func main() { var prodNum int fmt.Print("Enter the Number to find the Digits Product = ") fmt.Scanln(&prodNum) product = digitsProduct(prodNum) fmt.Println("The Product of Digits in this Number = ", product) }
SureshMac:GoExamples suresh$ go run digitsProd3.go
Enter the Number to find the Digits Product = 4789
The Product of Digits in this Number = 2016
SureshMac:GoExamples suresh$ go run digitsProd3.go
Enter the Number to find the Digits Product = 22457
The Product of Digits in this Number = 560