This Go Program to find the Largest of Three Numbers uses the Else If statement. Within the Else if, we used the logical and operator to separate two conditions.
- a > b && a > c – Check whether a is greater than b and c. If True, a is largest.
- else if b > a && b > c – Check b is greater than a and c. If True, b is largest.
- else if c > a && c > b – Check c is greater than a and b. If True, c is largest. Otherwise, either of them is equal.
package main import "fmt" func main() { var a, b, c int fmt.Print("\nEnter the Three Numbers to find Largest = ") fmt.Scanln(&a, &b, &c) if a > b && a > c { fmt.Println(a, " is Greater Than ", b, " and ", c) } else if b > a && b > c { fmt.Println(b, " is Greater Than ", a, " and ", c) } else if c > a && c > b { fmt.Println(c, " is Greater Than ", a, " and ", b) } else { fmt.Println("Either of them are Equal ") } }
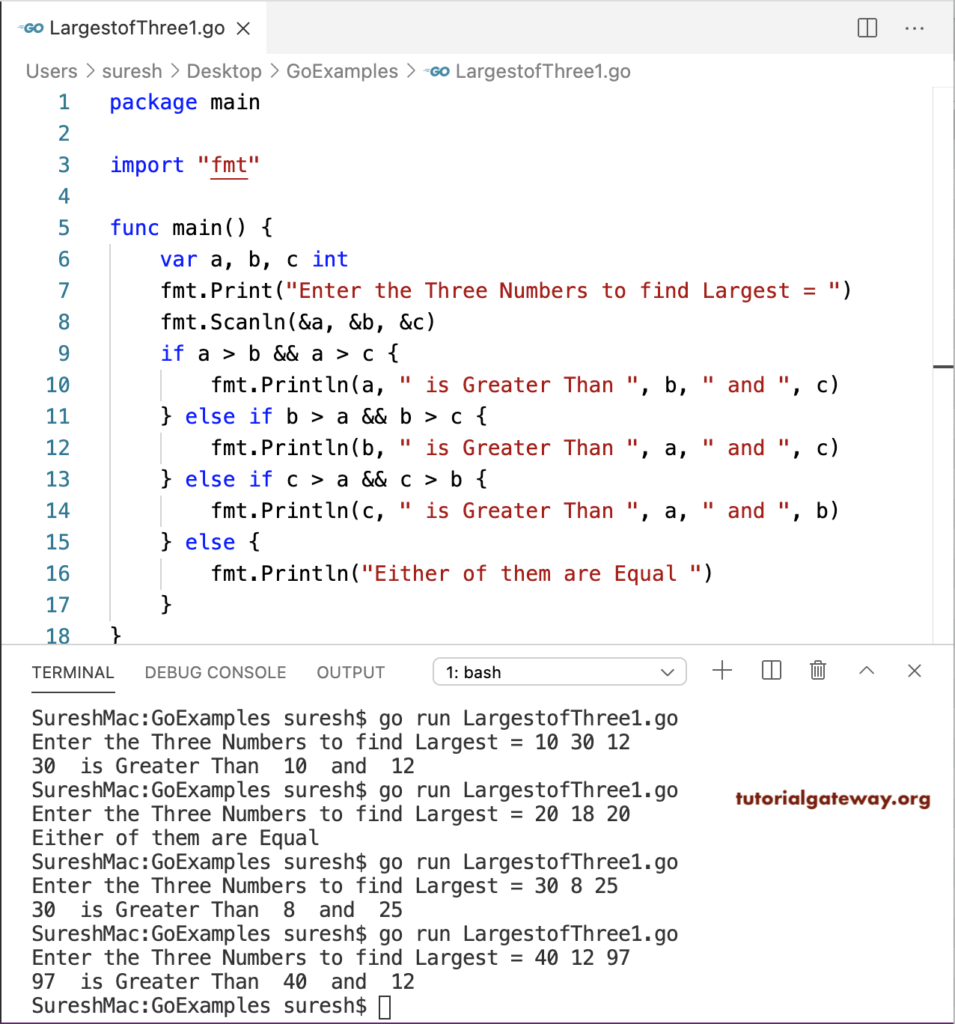
Go Program to find Largest of Three Numbers.
This Golang program uses the arithmetic operator and logical operator to print the Largest of Three Numbers.
package main import "fmt" func main() { var a, b, c int fmt.Print("\nEnter the Three Numbers to find Largest = ") fmt.Scanln(&a, &b, &c) if a-b > 0 && a-c > 0 { fmt.Println(a, " is Greater Than ", b, " and ", c) } else { if b-c > 0 { fmt.Println(b, " is Greater Than ", a, " and ", c) } else { fmt.Println(c, " is Greater Than ", a, " and ", b) } } }
SureshMac:Goexamples suresh$ go run LargestofThree2.go
Enter the Three Numbers to find Largest = 3 5 2
5 is Greater Than 3 and 2
SureshMac:Goexamples suresh$ go run LargestofThree2.go
Enter the Three Numbers to find Largest = 19 2 27
27 is Greater Than 19 and 2
SureshMac:Goexamples suresh$ go run LargestofThree2.go
Enter the Three Numbers to find Largest = 22 7 20
22 is Greater Than 7 and 20