Write a Go Program to check or find whether the given character is an Alphabet or not. The unicode package has an IsLetter function to check the alphabet. We used the If else statement (if unicode.IsLetter(alr)) along with IsLetter to find the given Rune is an alphabet or not.
package main import ( "bufio" "fmt" "os" "unicode" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Any Character to Check Alphabet = ") alr, _, _ := reader.ReadRune() if unicode.IsLetter(alr) { fmt.Printf("%c is an Alphabet\n", alr) } else { fmt.Printf("%c is Not a Alphabet\n", alr) } }
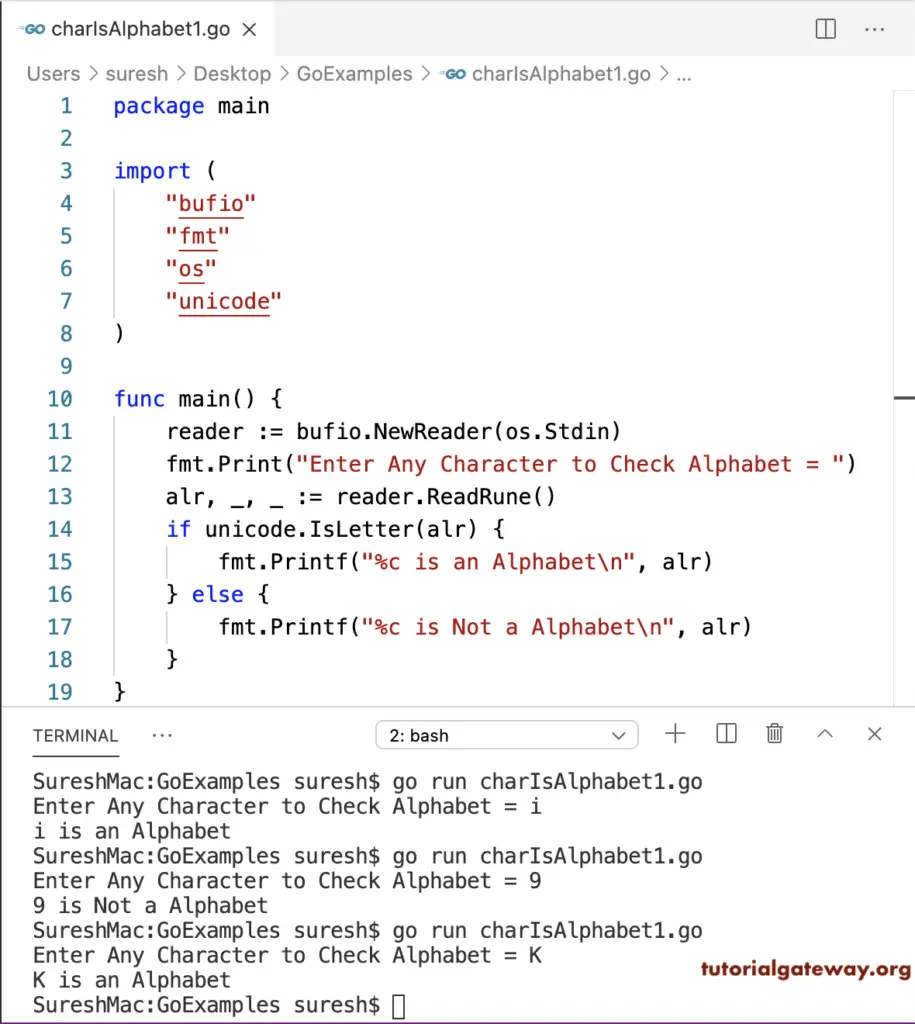
Go Program to Find Character is an Alphabet
In this Go example, we convert the given byte character to Rune (if unicode.IsLetter(rune(alch))) and then use the IsLetter function.
package main import ( "bufio" "fmt" "os" "unicode" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Any Character to Check Alphabet = ") alch, _ := reader.ReadByte() if unicode.IsLetter(rune(alch)) { fmt.Printf("%c is an Alphabet\n", alch) } else { fmt.Printf("%c is Not a Alphabet\n", alch) } }
SureshMac:GoExamples suresh$ go run charIsAlphabet2.go
Enter Any Character to Check Alphabet = Y
Y is an Alphabet
SureshMac:GoExamples suresh$ go run charIsAlphabet2.go
Enter Any Character to Check Alphabet = ?
? is Not a Alphabet
SureshMac:GoExamples suresh$ go run charIsAlphabet2.go
Enter Any Character to Check Alphabet = q
q is an Alphabet
Any character that is between the a or A to Z or Z called an alphabet. We used the If condition (if alch >= ‘a’ && alch <= ‘z’ || alch >= ‘A’ && alch <= ‘Z’) to check whether the character is between a to z.
package main import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Any Character to Check Alphabet = ") alch, _ := reader.ReadByte() if alch >= 'a' && alch <= 'z' || alch >= 'A' && alch <= 'Z' { fmt.Printf("%c is an Alphabet\n", alch) } else { fmt.Printf("%c is Not a Alphabet\n", alch) } }
SureshMac:GoExamples suresh$ go run charIsAlphabet3.go
Enter Any Character to Check Alphabet = s
s is an Alphabet
SureshMac:GoExamples suresh$ go run charIsAlphabet3.go
Enter Any Character to Check Alphabet = K
K is an Alphabet
SureshMac:GoExamples suresh$ go run charIsAlphabet3.go
Enter Any Character to Check Alphabet = 8
8 is Not a Alphabet
This Golang Program uses the ASCII codes (if alch >= 65 && alch <= 90 || alch >= 97 && alch <= 122) to find or check whether the character is an Alphabet or not.
package main import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Any Character to Check Alphabet = ") alch, _ := reader.ReadByte() if alch >= 65 && alch <= 90 || alch >= 97 && alch <= 122 { fmt.Printf("%c is an Alphabet\n", alch) } else { fmt.Printf("%c is Not a Alphabet\n", alch) } }
SureshMac:GoExamples suresh$ go run charIsAlphabet4.go
Enter Any Character to Check Alphabet = q
q is an Alphabet
SureshMac:GoExamples suresh$ go run charIsAlphabet4.go
Enter Any Character to Check Alphabet = P
P is an Alphabet
SureshMac:GoExamples suresh$ go run charIsAlphabet4.go
Enter Any Character to Check Alphabet = @
@ is Not a Alphabet