Write a Go Program to find whether the character is a digit or not. The unicode isDigit checks whether the Rune is a Digit. We used the If else statement (if unicode.IsDigit(r)) along with the unicode IsDigit function to find the given character is a digit or not.
package main import ( "bufio" "fmt" "os" "unicode" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Any Character to Check Digit = ") r, _, _ := reader.ReadRune() if unicode.IsDigit(r) { fmt.Printf("%c is a Digit\n", r) } else { fmt.Printf("%c is Not a Digit\n", r) } }
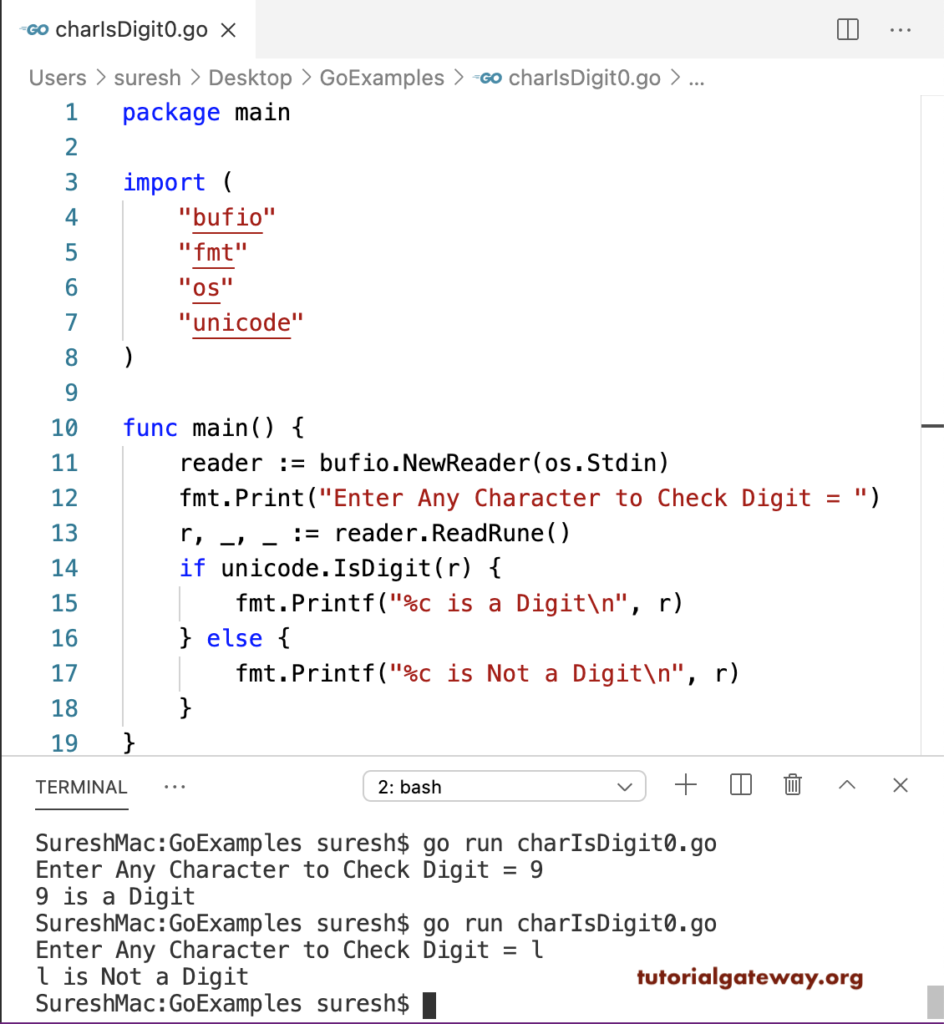
The Unicode package also has an IsNumber function (if unicode.IsNumber(r)) to check the character is a Digit or not. Here, we use this function.
package main import ( "bufio" "fmt" "os" "unicode" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Any Character to Check Digit = ") r, _, _ := reader.ReadRune() if unicode.IsNumber(r) { fmt.Printf("%c is a Digit\n", r) } else { fmt.Printf("%c is Not a Digit\n", r) } }
SureshMac:GoExamples suresh$ go run charIsDigit1.go
Enter Any Character to Check Digit = 8
8 is a Digit
SureshMac:GoExamples suresh$ go run charIsDigit1.go
Enter Any Character to Check Digit = @
@ is Not a Digit
Go Program to Find Character is a Digit or Not
In this Go example, we convert the given byte character to Rune (if unicode.IsDigit(rune(ch))) and then use the IsDigit function.
package main import ( "bufio" "fmt" "os" "unicode" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Any Character to Check Digit = ") ch, _ := reader.ReadByte() if unicode.IsDigit(rune(ch)) { fmt.Printf("%c is a Digit\n", ch) } else { fmt.Printf("%c is Not a Digit\n", ch) } }
SureshMac:GoExamples suresh$ go run charIsDigit2.go
Enter Any Character to Check Digit = 0
0 is a Digit
SureshMac:GoExamples suresh$ go run charIsDigit2.go
Enter Any Character to Check Digit = p
p is Not a Digit
Any number between 0 and 9 called a digit. We used the If condition (if ch >= ‘0’ && ch <= ‘9’) to check whether the character is a digit or not.
package main import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Any Character to Check Digit = ") ch, _ := reader.ReadByte() if ch >= '0' && ch <= '9' { fmt.Printf("%c is a Digit\n", ch) } else { fmt.Printf("%c is Not a Digit\n", ch) } }
SureshMac:GoExamples suresh$ go run charIsDigit3.go
Enter Any Character to Check Digit = 2
2 is a Digit
SureshMac:GoExamples suresh$ go run charIsDigit3.go
Enter Any Character to Check Digit = 8
8 is a Digit
This Golang Program uses the ASCII codes (if ch >= 48 && ch <= 57) to check whether the character is a digit or not.
package main import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Any Character to Check Digit = ") ch, _ := reader.ReadByte() if ch >= 48 && ch <= 57 { fmt.Printf("%c is a Digit\n", ch) } else { fmt.Printf("%c is Not a Digit\n", ch) } }
SureshMac:GoExamples suresh$ go run charIsDigit4.go
Enter Any Character to Check Digit = 5
5 is a Digit
SureshMac:GoExamples suresh$ go run charIsDigit4.go
Enter Any Character to Check Digit = n
n is Not a Digit