This Go program uses the printf statement and string formats to find and return the user-given character’s ASCII value.
package main import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Any Character to find ASCII = ") ch, _ := reader.ReadByte() fmt.Printf("The ASCII value of %c = %d\n", ch, ch) }
SureshMac:GoExamples suresh$ go run charASCII1.go
Enter Any Character to find ASCII = j
The ASCII value of j = 106
SureshMac:GoExamples suresh$ go run charASCII1.go
Enter Any Character to find ASCII = 0
The ASCII value of 0 = 48
SureshMac:GoExamples suresh$ go run charASCII1.go
Enter Any Character to find ASCII = o
The ASCII value of o = 111
This Go program allows users to enter Rune and finds the ASCII Value of a character.
package main import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Any Character to find ASCII = ") ch, _, _ := reader.ReadRune() fmt.Printf("The ASCII value of %c = %d\n", ch, ch) }
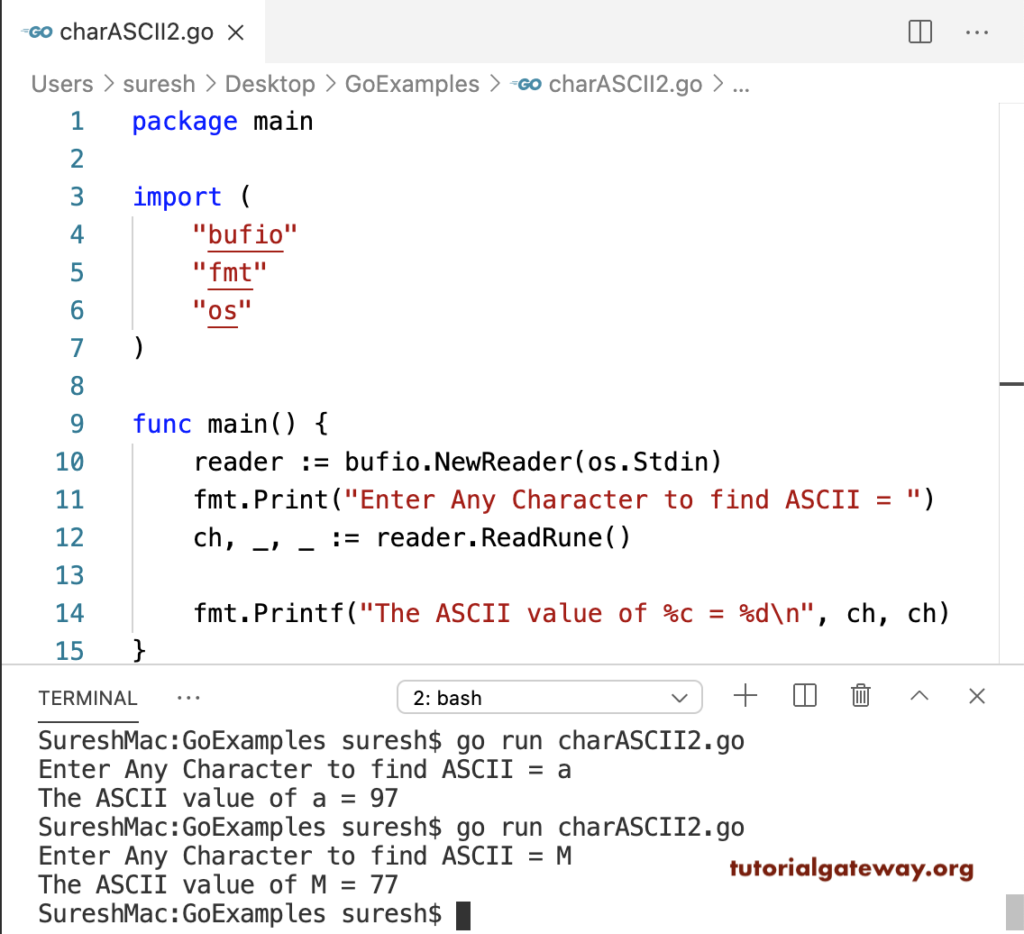