Write a Go program to Count Duplicates in an Array using For loop. Here, we used nested for loops, and the first for loop (for i = 0; i < dupsize; i++) iterates array items from 0 to n, and the inner for loop (for j := i + 1; j < dupsize; j++) starts at 1 (i+ 1). For each i value, nested for loop will iterate from i+1 to dupsize. Inside it, we used the If statement (if dupArr[i] == dupArr[j]) to check any of the array items is equal to the other. Once it found the duplicate array item, dupcount will increment, and the break will exit the GC from for loop.
package main import "fmt" func main() { var dupsize, i int fmt.Print("Enter the Even Array Size = ") fmt.Scan(&dupsize) dupArr := make([]int, dupsize) fmt.Print("Enter the Even Array Items = ") for i = 0; i < dupsize; i++ { fmt.Scan(&dupArr[i]) } dupcount := 0 for i = 0; i < dupsize; i++ { for j := i + 1; j < dupsize; j++ { if dupArr[i] == dupArr[j] { dupcount++ break } } } fmt.Println("\nThe Total Number of Duplicates in dupArr = ", dupcount) }
Enter the Even Array Size = 5
Enter the Even Array Items = 10 20 30 20 10
The Total Number of Duplicates in dupArr = 2
Golang Program to Count Duplicate Items in an Array using functions.
In this Go program, we created a separate (func countDuplicates(dupArr []int, dupsize int)) function that returns the count of duplicate array items.
package main import "fmt" var dupcount, i int func countDuplicates(dupArr []int, dupsize int) int { dupcount = 0 for i = 0; i < dupsize; i++ { for j := i + 1; j < dupsize; j++ { if dupArr[i] == dupArr[j] { dupcount++ break } } } return dupcount } func main() { var dupsize int fmt.Print("Enter the Even Array Size = ") fmt.Scan(&dupsize) dupArr := make([]int, dupsize) fmt.Print("Enter the Even Array Items = ") for i = 0; i < dupsize; i++ { fmt.Scan(&dupArr[i]) } dupcount = countDuplicates(dupArr, dupsize) fmt.Println("\nThe Total Number of Duplicates in dupArr = ", dupcount) }
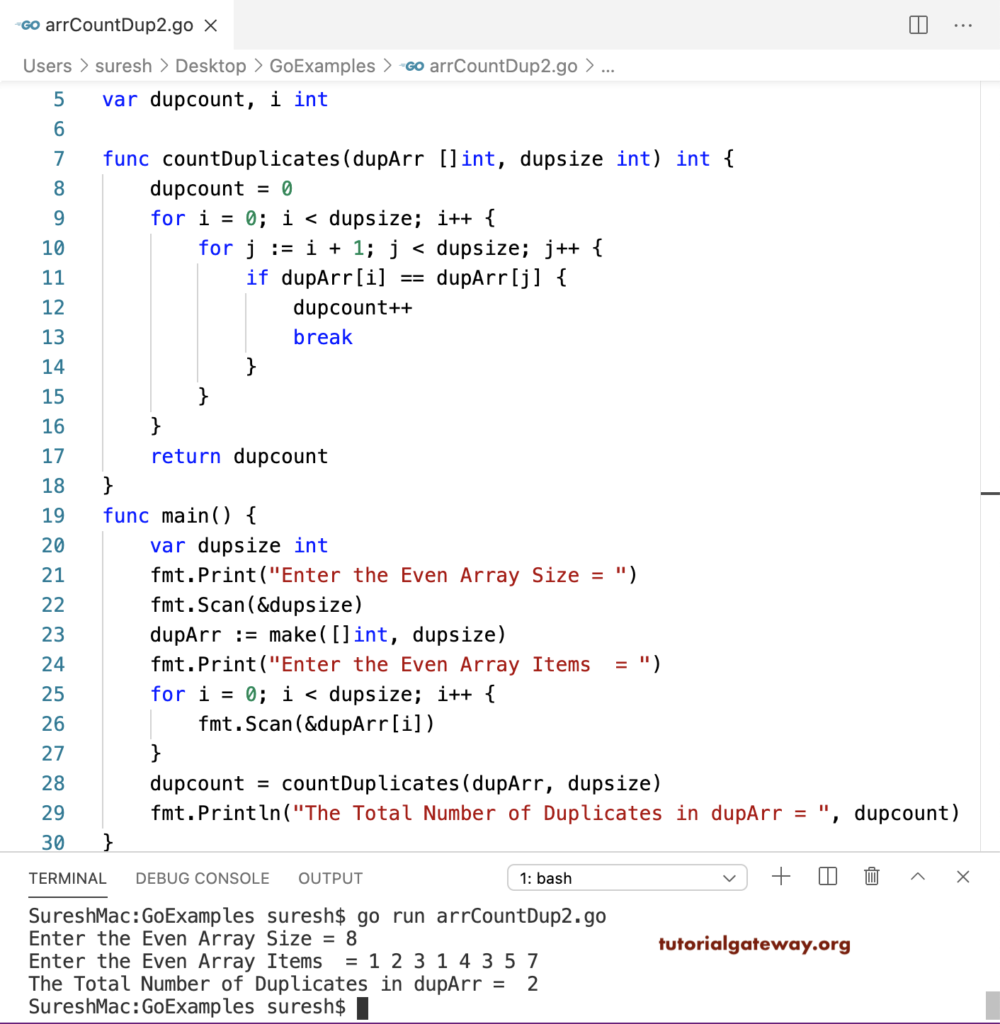