In this Go Program, to convert the lowercase character to uppercase, we used IsLetter (if unicode.IsLetter(lwch)) to check for alphabets. Next, we used the unicode ToUpper function (up := unicode.ToUpper(lwch)) that converts the lowercase to an uppercase character.
package main import ( "bufio" "fmt" "os" "unicode" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Character to Convert into Uppercase = ") lwch, _, _ := reader.ReadRune() if unicode.IsLetter(lwch) { up := unicode.ToUpper(lwch) fmt.Printf("The Uppercase Character of %c = %c\n", lwch, up) } else { fmt.Printf("Please Enter a Valid Alphabet\n") } }
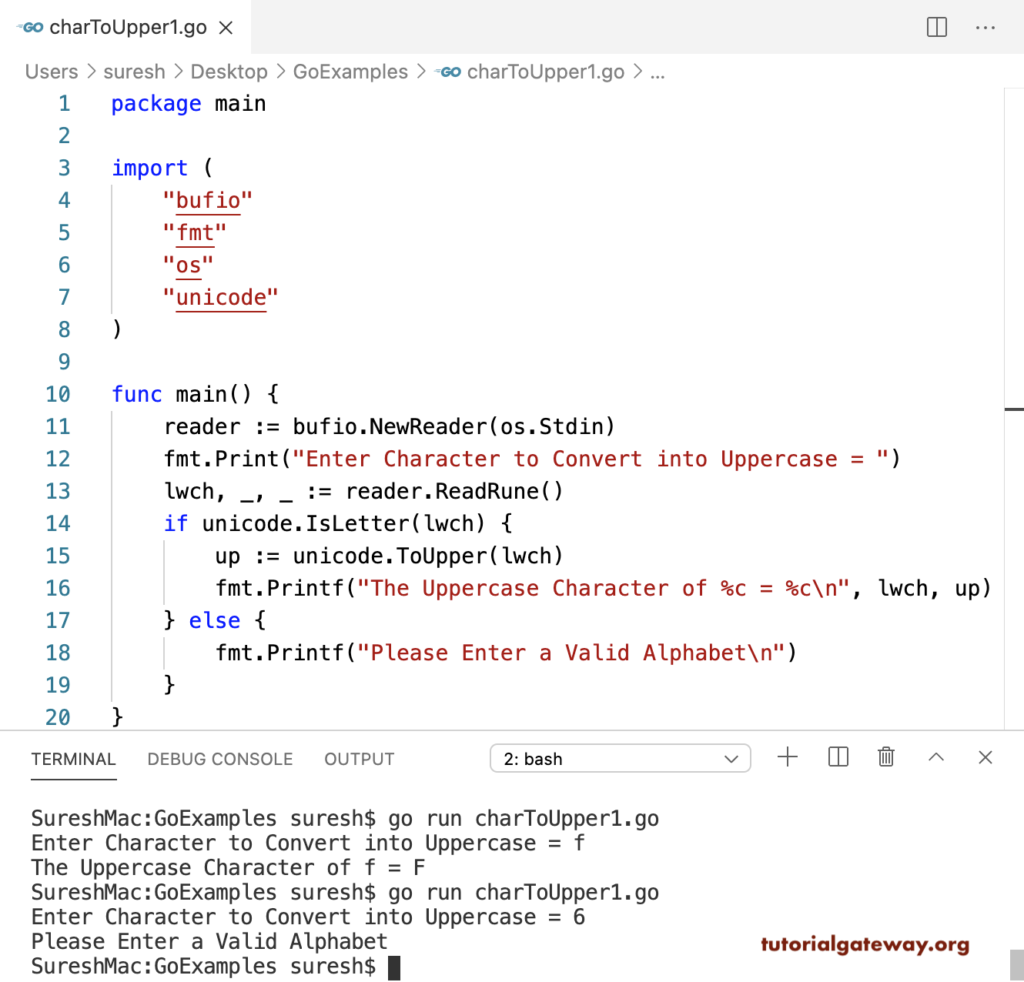
Go Program to Convert Lowercase Character to Uppercase
In this Go lowercase to uppercase convert example, we cast the user given byte character to Rune(unicode.ToUpper(rune(lwch))) and then use the ToUpper function.
package main import ( "bufio" "fmt" "os" "unicode" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Character to Convert into Uppercase = ") lwch, _ := reader.ReadByte() if unicode.IsLetter(rune(lwch)) { up := unicode.ToUpper(rune(lwch)) fmt.Printf("The Uppercase Character of %c = %c\n", lwch, up) } else { fmt.Printf("Please Enter a Valid Alphabet\n") } }
SureshMac:GoExamples suresh$ go run charToUpper2.go
Enter Character to Convert into Uppercase = q
The Uppercase Character of q = Q
SureshMac:GoExamples suresh$ go run charToUpper2.go
Enter Character to Convert into Uppercase = 9
Please Enter a Valid Alphabet
This Golang change Character to an Uppercase Program uses the ASCII codes (if lwch >= 97 && lwch <= 122) to find the lowercase character. If true, we subtracted 32 from its ASCII value (up := lwch – 32) to convert the lowercase character to uppercase.
package main import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Character to Convert into Uppercase = ") lwch, _ := reader.ReadByte() if lwch >= 97 && lwch <= 122 { up := lwch - 32 fmt.Printf("The Uppercase Character of %c = %c\n", lwch, up) } else { fmt.Printf("Either You entered the Uppercase Char or Inalid Alphabet\n") } }
SureshMac:GoExamples suresh$ go run charToUpper3.go
Enter Character to Convert into Uppercase = k
The Uppercase Character of k = K
SureshMac:GoExamples suresh$ go run charToUpper3.go
Enter Character to Convert into Uppercase = M
Either You entered the Uppercase Char or Inalid Alphabet
In this Golang example, We used the a and z to find the lowercase character. If True, we are subtracting 32 from its ASCII value.
package main import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Character to Convert into Uppercase = ") lwch, _ := reader.ReadByte() if lwch >= 'a' && lwch <= 'z' { up := lwch - 32 fmt.Printf("The Uppercase Character of %c = %c\n", lwch, up) } else { fmt.Printf("Either You entered the Uppercase Char or Inalid Alphabet\n") } }
SureshMac:GoExamples suresh$ go run charToUpper4.go
Enter Character to Convert into Uppercase = s
The Uppercase Character of s = S
SureshMac:GoExamples suresh$ go run charToUpper4.go
Enter Character to Convert into Uppercase = S
Either You entered the Uppercase Char or Inalid Alphabet