Write a Go Program to check whether the character is lowercase or not. The unicode IsLower finds the Rune is a Lowercase character. We used this IsLower function in the If else statement (if unicode.IsLower(lwch)) to find the given character is lowercase or not.
package main import ( "bufio" "fmt" "os" "unicode" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Character to Check Lowercase = ") lwch, _, _ := reader.ReadRune() if unicode.IsLower(lwch) { fmt.Println("You have Entered the Lowercase Character") } else { fmt.Println("The entered Character is Not the Lowercase") } }
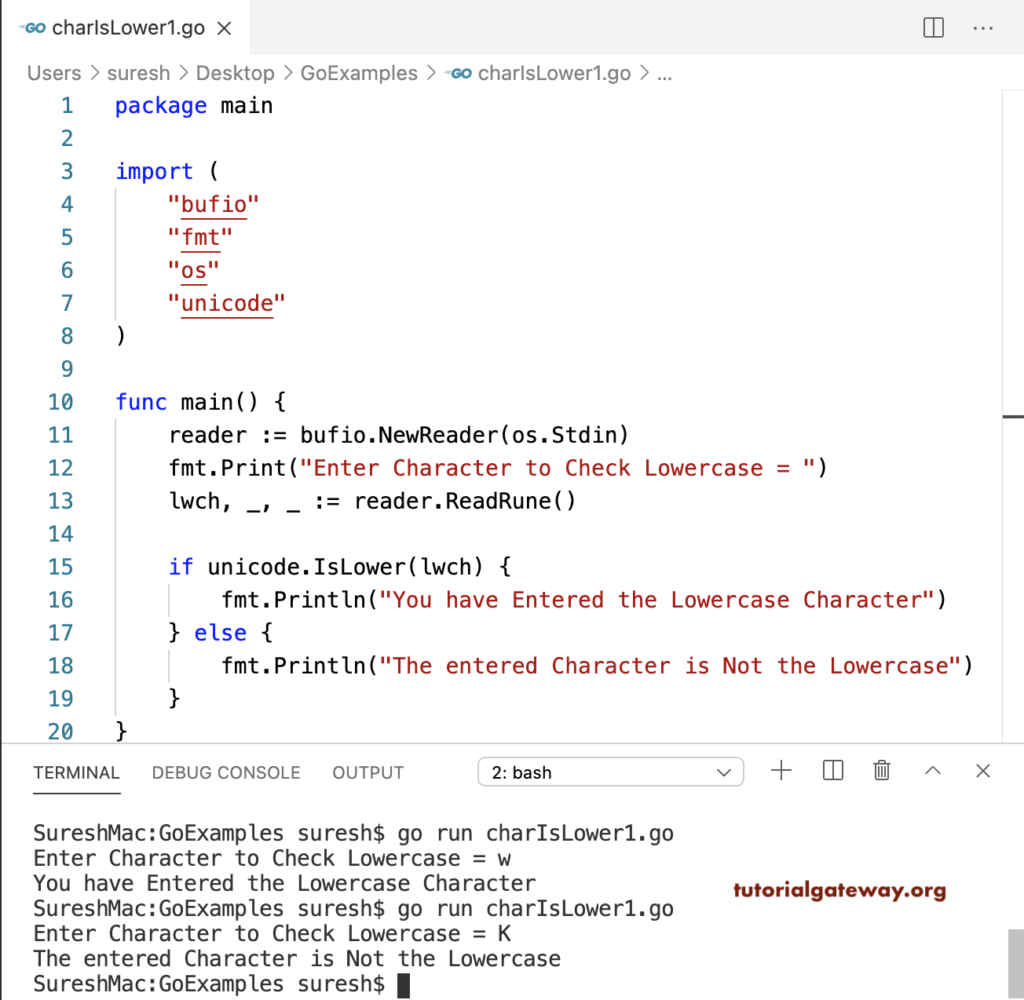
Go Program to Check Character is a Lowercase or Not
In this Go lowercase example, we change the given byte character to Rune (if unicode.IsLower(rune(lwch))) and then use the IsLower function.
package main import ( "bufio" "fmt" "os" "unicode" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Character to Check Lowercase = ") lwch, _ := reader.ReadByte() if unicode.IsLower(rune(lwch)) { fmt.Println("You have Entered the Lowercase Character") } else { fmt.Println("The entered Character is Not the Lowercase") } }
SureshMac:GoExamples suresh$ go run charIsLower2.go
Enter Character to Check Lowercase = o
You have Entered the Lowercase Character
SureshMac:GoExamples suresh$ go run charIsLower2.go
Enter Character to Check Lowercase = M
The entered Character is Not the Lowercase
In this Golang example, We used the If condition (if lwch >= ‘a’ && lwch <= ‘z’) to check whether the given character is between a and z, and if true, it is lowercase.
package main import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Character to Check Lowercase = ") lwch, _ := reader.ReadByte() if lwch >= 'a' && lwch <= 'z' { fmt.Println("You have Entered the Lowercase Character") } else { fmt.Println("The entered Character is Not the Lowercase") } }
SureshMac:GoExamples suresh$ go run charIsLower3.go
Enter Character to Check Lowercase = l
You have Entered the Lowercase Character
SureshMac:GoExamples suresh$ go run charIsLower3.go
Enter Character to Check Lowercase = Y
The entered Character is Not the Lowercase
This Golang Program uses the ASCII values (if lwch >= 97 && lwch <= 122) to find the character is lowercase or not.
package main import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Enter Character to Check Lowercase = ") lwch, _ := reader.ReadByte() if lwch >= 97 && lwch <= 122 { fmt.Println("You have Entered the Lowercase Character") } else { fmt.Println("The entered Character is Not the Lowercase") } }
SureshMac:GoExamples suresh$ go run charIsLower4.go
Enter Character to Check Lowercase = q
You have Entered the Lowercase Character
SureshMac:GoExamples suresh$ go run charIsLower4.go
Enter Character to Check Lowercase = Z
The entered Character is Not the Lowercase