The C strchr function is a String Function, which returns a pointer to the first occurrence of a character in the given string. The syntax of the strchr is
void *strchr(const char *str, const char *chr_to_look);
- str: A valid string
- chr_to_look: Character value that you want to search inside str
strchr function in C Language Example
The strchr function searches for the first occurrence of a character within the user-specified string. This program will help you to understand the strchr with multiple examples.
TIP: You have to include the C Programming #include<string.h> header before using this String Function.
#include <stdio.h> #include<string.h> int main() { char str[] = "C tutorial at tutorial gateway"; char *ch; ch = strchr(str, 'i'); if(ch) { printf("We Found your First Occurence of a Given Character"); printf("\nThe Final String From 'b' is : %s \n", ch); } else { printf("Given Character is Not found. Sorry!! \n"); } return 0; }
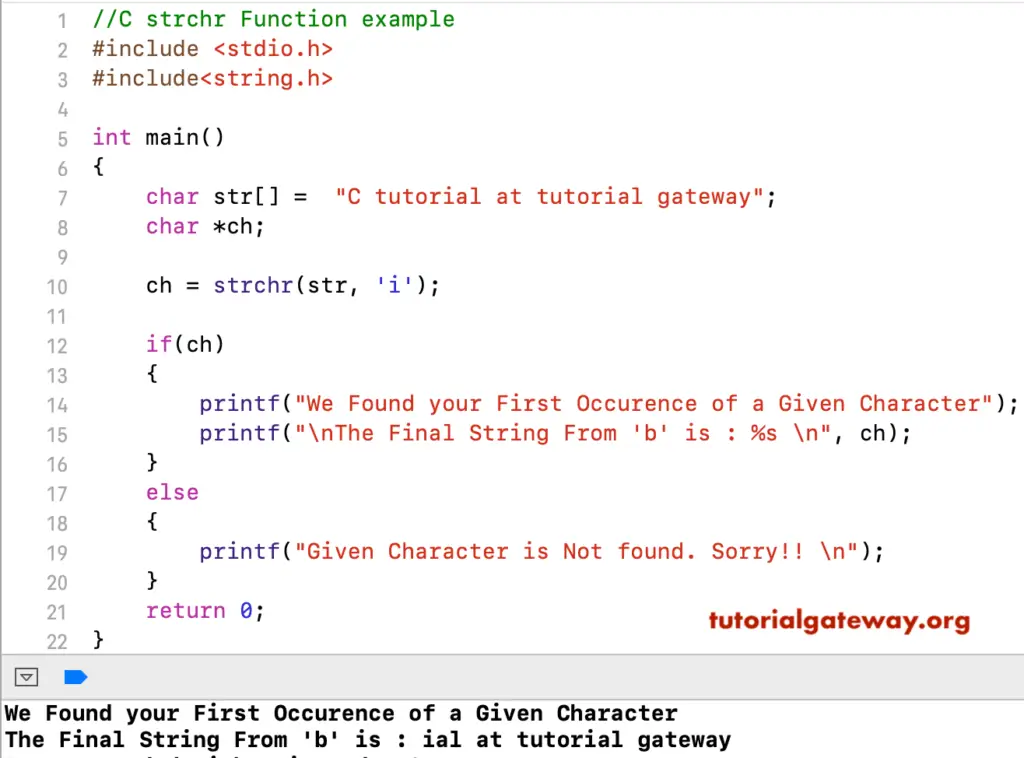