The C round function is one of the Math Functions used to return the nearest value (rounded value) of a given number or a specified expression. The syntax of the math round function is
double round(double round);
C round Function Example
The math round Function allows you to find the closest integer value of a given number. In this program, We are going to find the closest integer value of different numbers and display the output.
#include <stdio.h> #include <math.h> int main() { printf("\n The Round Value of 0.75 = %.2f ", round(0.75)); printf("\n The Round Value of 15.25 = %.2f ", round(15.25)); printf("\n The Round Value of 152.50 = %.2f ", round(152.50)); printf("\n The Round Value of -14.36 = %.2f ", round(-14.36)); printf("\n The Round Value of -26.82 = %.2f ", round(-26.32)); printf("\n The Round Value of -90.50 = %.2f \n", round(-90.50)); return 0; }
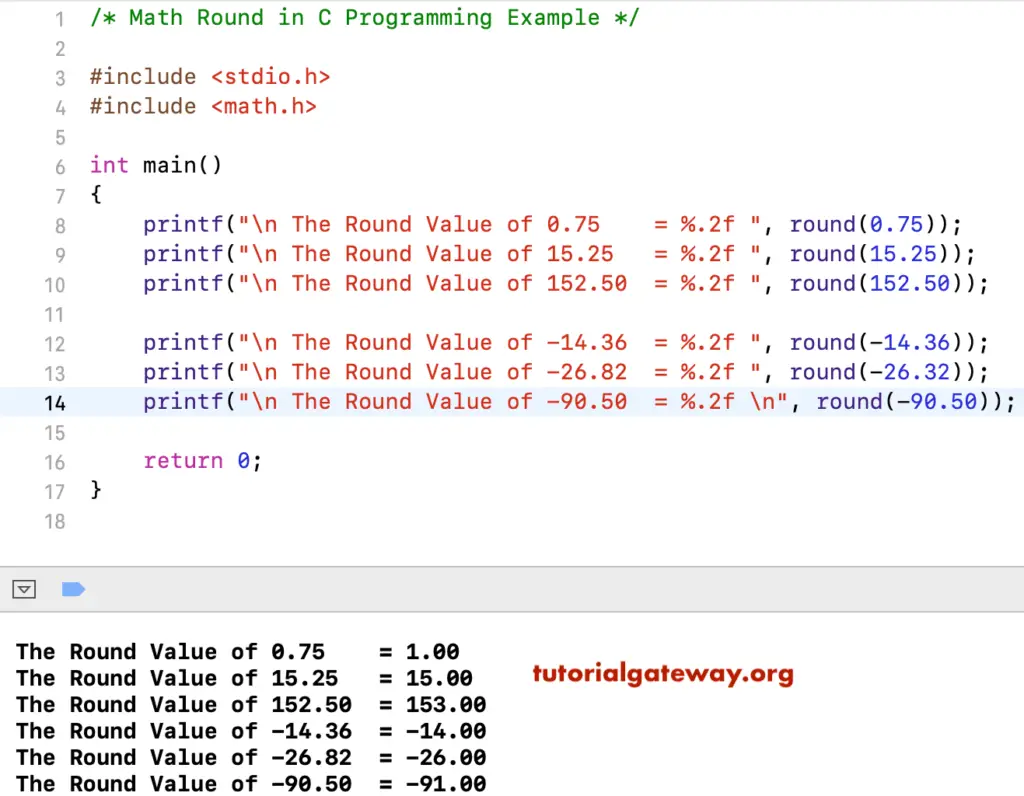
C math round Example 2
In this C Programming example, we are allowing the user to enter their own value. Next, this program uses the round function to find the rounded (nearest) value.
#include <stdio.h> #include <math.h> int main() { float number, roundValue; printf(" Please Enter any Numeric Value to Round : "); scanf("%f", &number); roundValue = round(number); printf("\n The Round Value of %.2f = %.4f \n", number, roundValue); return 0; }
Please Enter any Numeric Value to Round : 12.23
The Round Value of 12.23 = 12.0000