Write a C Program to Remove Last Occurrence of a Character in a String with example.
C Program to Remove Last Occurrence of a Character in a String Example 1
This program allows the user to enter a string (or character array), and a character value. Next, this C program will find and remove last occurrence of a character inside a string.
/* C Program to Remove Last Occurrence of a Character in a String */ #include <stdio.h> #include <string.h> int main() { char str[100], ch; int i, index, len; index = -1; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Character that you want to Search for : "); scanf("%c", &ch); len = strlen(str); for(i = 0; i < len; i++) { if(str[i] == ch) { index = i; } } if(index != -1) { i = index; while(i < len) { str[i] = str[i + 1]; i++; } } printf("\n The Final String after Removing Last Occurrence of '%c' = %s ", ch, str); return 0; }
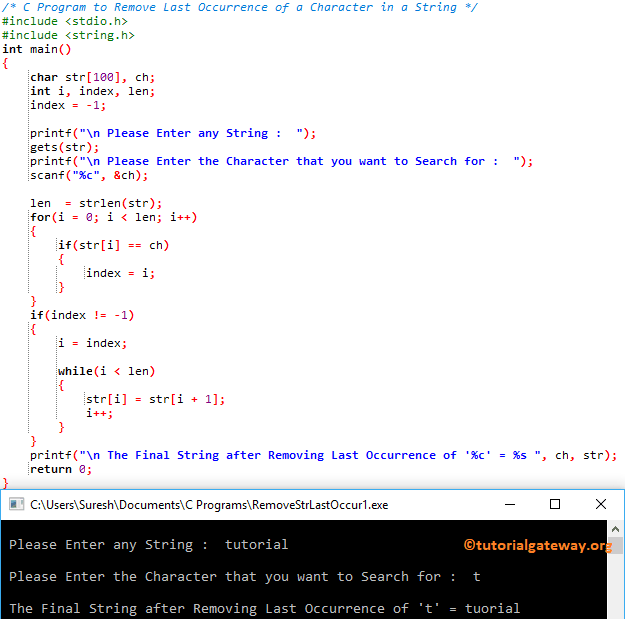
First, we used For Loop to iterate each character in a String. Within the loop, we are checking whether the individual character is equal to a user-specified character or not. If True, then we are assigning i value to index variable.
for(i = 0; i < len; i++) { if(str[i] == ch) { index = i; } }
Next, we used the If Statement to check the index value is not equal to -1. If it is True then the C Programming compiler will shift the characters
str[] = tutorial
ch = t
Index = -1
For Loop First Iteration: for(i = 0; i < len; i++)
The condition is True because 0 < 8.
Within the While Loop, we used If statement to check whether the str[0] is equal to a given character or not
if(str[i] == ch) => if(t == t) – condition is True. So,
index = i = 0.
Second Iteration: for(i = 1; 1 < 8; 1++)
if(str[1] == ch) => if(u == l) – condition is false. So, i will be incremented.
Third Iteration: for(i = 2; 2 <= 5; 2++)
if(str[i] == ch) => if(t == t) – condition is True.
Index = i
index = 2
Do the same for remaining iterations.
Next, we used the If Statement to check the index value is not equal to -1. Here, the condition is True so, i = index
i = 2
While Loop First Iteration: while(i < len)
=> while(2 < 8) – Condition is True
str[i] = str[i + 1]
str[2] = str[3]
str[2] = o
i will be incremented
Second Iteration: while(3 < 8)
str[3] = str[4]
str[3] = r
i will increment
Third Iteration: while(4 < 8)
str[4] = str[5]
str[4] = i
i will increment
Do the same for remaining iterations until i becomes 8.
At last, we used the printf statement to print the final string
printf("\n The Last Occurrence of the Search Element '%c' is at Position %d ", ch, i + 1);
C Program to Remove Last Occurrence of a Character in a String Example 2
This program to remove the last occurrence of a character is the same as the above. Here, we just replaced the For Loop with While Loop.
/* C Program to Remove Last Occurrence of a Character in a String */ #include <stdio.h> #include <string.h> int main() { char str[100], ch; int i, index, len; index = -1; i = 0; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Character that you want to Search for : "); scanf("%c", &ch); len = strlen(str); while(i < len) { if(str[i] == ch) { index = i; } i++; } if(index != -1) { i = index; while(i < len) { str[i] = str[i + 1]; i++; } } printf("\n The Final String after Removing Last Occurrence of '%c' = %s ", ch, str); return 0; }
Please Enter any String : tutorial gateway
Please Enter the Character that you want to Search for : t
The Final String after Removing Last Occurrence of 't' = tutorial gaeway
C Program to Remove Last Occurrence of a Character in a String Example 3
This program to remove the last character occurrence is the same as the first example. Still, this time, we used the Functions concept to separate the logic.
/* C Program to Remove Last Occurrence of a Character in a String */ #include <stdio.h> #include <string.h> void Remove_LastOccurrence(char *str, char ch); int main() { char str[100], ch; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Character that you want to Search for : "); scanf("%c", &ch); Remove_LastOccurrence(str, ch); printf("\n The Final String after Removing Last Occurrence of '%c' = %s ", ch, str); return 0; } void Remove_LastOccurrence(char *str, char ch) { int i, index, len; len = strlen(str); for(i = 0; i < len; i++) { if(str[i] == ch) { index = i; } } if(index != -1) { i = index; while(i < len) { str[i] = str[i + 1]; i++; } } }
Please Enter any String : c programming
Please Enter the Character that you want to Search for : g
The Final String after Removing Last Occurrence of 'g' = c programmin