Write a C Program to find All Occurrence of a Character in a String with example.
C Program to find All Occurrence of a Character in a String Example 1
This program allows the user to enter a string (or character array), and a character value. Next, it will search and find all the occurrences of a character inside this string using If Else Statement.
/* C Program to find All Occurrence of a Character in a String */ #include <stdio.h> #include <string.h> int main() { char str[100], ch; int i; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Character that you want to Search for : "); scanf("%c", &ch); for(i = 0; i <= strlen(str); i++) { if(str[i] == ch) { printf("\n '%c' is Found at Position %d ", ch, i + 1); } } return 0; }
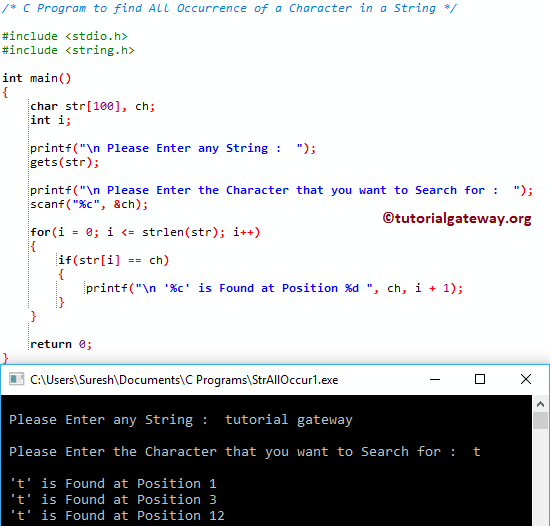
First we used For Loop to iterate each and every character in a String.
for(i = 0; i <= strlen(str); i++) { if(str[i] == ch) { printf("\n '%c' is Found at Position %d ", ch, i + 1); } }
str[] = tutorial gateway
ch = t
For Loop First Iteration: for(i = 0; i <= strlen(str); i++)
The condition is True because 0 <= 16.
Within the C Programming While Loop, we used If statement to check whether the str[0] is equal to a user-specified character or not
if(str[i] == ch) => if(t == t)
The above condition is True. So, the compiler will execute the printf statement inside the If block.
Here, i is the index position (start with zero), and i + 1 is the actual position.
Second Iteration: for(i = 1; 1 <= 16; 1++) – The condition is True because 1 <= 16.
if(str[i] == ch) => if(t == l) – condition is false. So, i will increment.
Third Iteration: for(i = 2; 2 <= 16; 2++)
if(str[i] == ch) => if(t == t) – condition is True. So, compiler will execute the printf statement inside the If block.
Do the same for the remaining iterations.
C Program to find All Occurrence of a Character in a String Example 2
This program to find all occurrence of a character is the same as above. Here, we just replaced the For Loop with While Loop.
/* C Program to find All Occurrence of a Character in a String */ #include <stdio.h> #include <string.h> int main() { char str[100], ch; int i; i = 0; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Character that you want to Search for : "); scanf("%c", &ch); while(str[i] != '\0') { if(str[i] == ch) { printf("\n '%c' is Found at Position %d ", ch, i + 1); } i++; } return 0; }
Please Enter any String : Hello World
Please Enter the Character that you want to Search for : l
'l' is Found at Position 3
'l' is Found at Position 4
'l' is Found at Position 10
Program to find All Occurrence of a Character in a String Example 3
This program is the same as the first example, but this time we used the Functions concept to separate the logic.
/* C Program to find All Occurrence of a Character in a String */ #include <stdio.h> #include <string.h> void Find_AllOccurrence(char str[], char ch); int main() { char str[100], ch; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Character that you want to Search for : "); scanf("%c", &ch); Find_AllOccurrence(str, ch); return 0; } void Find_AllOccurrence(char str[], char ch) { int i; for(i = 0; str[i] != '\0'; i++) { if(str[i] == ch) { printf("\n '%c' is Found at Position %d ", ch, i + 1); } } }
Please Enter any String : welcome to tutorial gateway
Please Enter the Character that you want to Search for : t
't' is Found at Position 9
't' is Found at Position 12
't' is Found at Position 14
't' is Found at Position 23