How to write a C Program to check Matrix is an Identity Matrix or not with example. An Identity Matrix is a square matrix whose main diagonal elements are ones, and all the other elements are zeros.
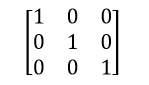
C Program to check Matrix is an Identity Matrix Example
This program allows the user to enter the number of rows and columns of a Matrix. Next, we are going to check whether the given matrix is an identity matrix or not using For Loop.
/* C Program to check Matrix is an Identity Matrix or Not */ #include<stdio.h> int main() { int i, j, rows, columns, a[10][10], Flag = 1; printf("\n Please Enter Number of rows and columns : "); scanf("%d %d", &i, &j); printf("\n Please Enter the Matrix Elements \n"); for(rows = 0; rows < i; rows++) { for(columns = 0; columns < j; columns++) { scanf("%d", &a[rows][columns]); } } for(rows = 0; rows < i; rows++) { for(columns = 0; columns < j; columns++) { if(a[rows][columns] != 1 && a[columns][rows] != 0) { Flag = 0; break; } } } if(Flag == 1) { printf("\n The Matrix that you entered is an Identity Matrix "); } else { printf("\n The Matrix that you entered is Not an Identity Matrix "); } return 0; }
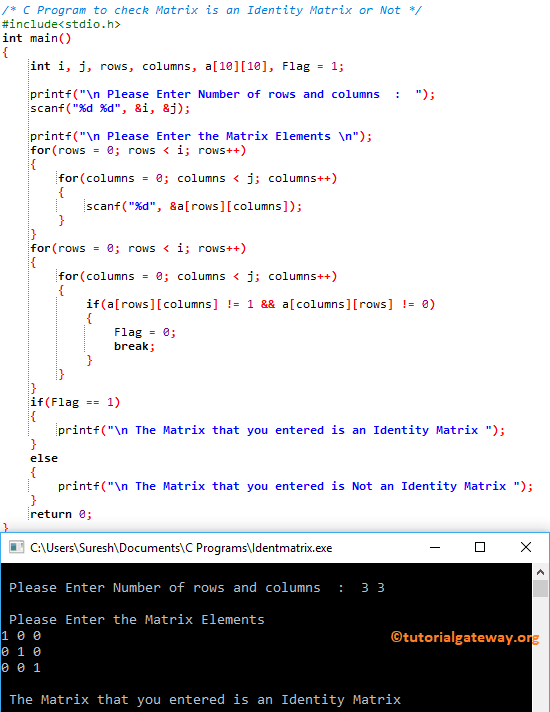
In this Program to check Matrix is an Identity Matrix, We declared single Two dimensional arrays Multiplication of size of 10 * 10.
Below C Programming statements asks the User to enter the Matrix size (Number of rows and columns. For instance 2 Rows, 2 Columns = a[2][2] )
printf("\n Please Enter Number of rows and columns : "); scanf("%d %d", &i, &j);
Next, we used for loop to iterate each item present in a[2][2] matrix. Conditions inside the for loops ((rows < i) and (columns < j)) will ensure the compiler, not to exceed the matrix limit. Otherwise, the Matrix will overflow. The scanf statement inside the for loop will store the user entered values in every individual array element such as a[0][0], a[0][1], …..
for(rows = 0; rows < i; rows++). { for(columns = 0; columns < j; columns++) { scanf("%d", &a[rows][columns]); } }
In the next line, We have one more for loop to check the matrix is an identity matrix or not
for(rows = 0; rows < i; rows++) { for(columns = 0; columns < j; columns++) { if(a[rows][columns] != 1 && a[columns][rows] != 0) { Flag = 0; break; } } }
Let me try the program with other values
Please Enter Number of rows and columns : 3 3
Please Enter the Matrix Elements
1 0 0
0 1 0
0 1 1
The Matrix that you entered is Not an Identity Matrix
C Program to check Matrix is an Identity Matrix Example 2
This program is similar to the above example. But, this time, we are using the Else If Statement instead of If Statement.
/* C Program to check if a Given Matrix is an Identity Matrix or Not */ #include<stdio.h> int main() { int i, j, rows, columns, a[10][10], Flag = 1; printf("\n Please Enter Number of rows and columns : "); scanf("%d %d", &i, &j); printf("\n Please Enter the Matrix Elements \n"); for(rows = 0; rows < i; rows++) { for(columns = 0;columns < j;columns++) { scanf("%d", &a[rows][columns]); } } for(rows = 0; rows < i; rows++) { for(columns = 0; columns < j; columns++) { if(rows == columns && a[rows][columns] != 1) { Flag = 0; } else if(rows != columns && a[rows][columns] != 0) { Flag = 0; } } } if(Flag == 1) { printf("\n The Matrix that you entered is an Identity Matrix "); } else { printf("\n The Matrix that you entered is Not an Identity Matrix "); } return 0; }
C identity matrix output
Please Enter Number of rows and columns : 3 3
Please Enter the Matrix Elements
1 0 0
0 1 0
0 0 1
The Matrix that you entered is an Identity Matrix