How to Write C Program For Strong Number Using While Loop, For Loop, and Recursive Function?. This article will also show you How to write a C program to print Strong Numbers between 1 and n.
C Program For Strong Number Using While Loop
This program allows the user to enter any positive integer. Then, this C program will check whether the number is a Strong Number or Not using the While Loop.
#include <stdio.h> int main() { int Number, Temp, Reminder, Sum = 0, i; long Factorial; printf("\nPlease Enter a Number to Check for Strong Number \n"); scanf("%d", &Number); //Helps to prevent altering the original value Temp = Number; while( Temp > 0) { Factorial = 1, i = 1; Reminder = Temp % 10; while (i <= Reminder) { Factorial = Factorial * i; i++; } printf("\n Factorial of %d = %d\n", Reminder, Factorial); Sum = Sum + Factorial; Temp = Temp /10; } printf("\n Sum of the Factorials of a Given Number %d is = %d\n", Number, Sum); if ( Number == Sum ) printf("\n %d is a Strong Number.\n", Number); else printf("\n %d is not a Strong Number.\n", Number); return 0; }
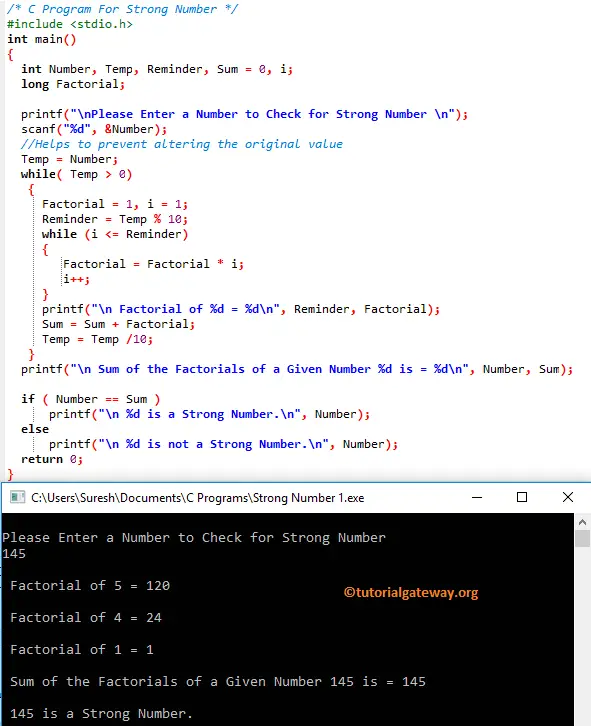
This C program for a strong number allows the user to enter any positive integer. It will assign it to a Number variable.
Next, we assign the original value to the Temp variable. It will help us to preserve our original value while we do all the manipulation on the Temp variable.
Below C Programming While loop will make sure that the given number is greater than 0, the statements inside the While Loop will split the numbers and find the factorial of individual digits inside the given number. If you don’t understand the logic, Please refer to the C Program to Count Digits article.
Here, the first while Loop (Outer one) in C strong number program will split the number into individual digits. Next, the inner while loop will find the factorial of that digit. I suggest you refer to the C Program to find the Factorials article to understand the logic behind the factorial.
User Entered value of this example: Number = 145 and Sum = 0
Temp = Number
Temp = 145
First Iteration
Reminder = Temp % 10
Reminder = 145 % 10 = 5
Now, it will enter into the Inner While loop. Here, it will calculate the factorial of 5, which is 120.
Sum = Sum +120
Sum = 0 + 120 => 120
Temp = Temp /10
Temp = 145 /10 => 14
Second Iteration
From the first Iteration of the C program to check strong number, the values of both Temp and Sum have changed as Temp = 14 and Sum = 120
Reminder = Temp % 10
Reminder = 14 % 10 = 4
Now, it will enter into the Inner While loop. Here, it will calculate the factorial of 4, which is 24.
Sum = 120 + 24
Sum = 144
Temp = 14 /10 => 1
Third Iteration
From the Third Iteration of the c program for strong number, the values of both changed as Temp = 1 and Sum = 144
Reminder = 1 % 10 = 0
Here, the factorial of 1 is 1
Sum = 144 + 1 = 145
Temp = 1 / 10 => 0
Here, Temp = 0, so the while loop condition will fail
if ( Number== Sum ) – Condition will check whether the user entered number is exactly equal to the Sum number or not. If this condition is True, then it is a Strong Number. Else, it is not.
Let me check with another value in this strong number program
Please Enter a Number to Check
153
Factorial of 3 = 6
Factorial of 5 = 120
Factorial of 1 = 1
Sum of the Factorials of a Given Number 153 is = 127
153 is not.
C Program For Strong Number Using For Loop
This program allows the user to enter any positive integer. Then, this C program will check whether a number is Strong or Not using For Loop.
#include <stdio.h> int main() { int Num, Temp, Reminder, Sum = 0, i; long Factorial; printf("\nPlease Enter a Number \n"); scanf("%d", &Num); //Helps to prevent altering the original value Temp = Num; for(Temp = Num; Temp > 0; Temp = Temp / 10 ) { Factorial = 1; Reminder = Temp % 10; for (i = 1; i <= Reminder; i++) { Factorial = Factorial * i; } printf(" Fact of %d = %d\n", Reminder, Factorial); Sum = Sum + Factorial; } printf("\n Sum of the Factorials of %d is = %d\n", Num, Sum); if ( Number == Sum ) printf("\n %d is a Strong.\n", Num); else printf("\n %d is not.\n", Num); return 0; }
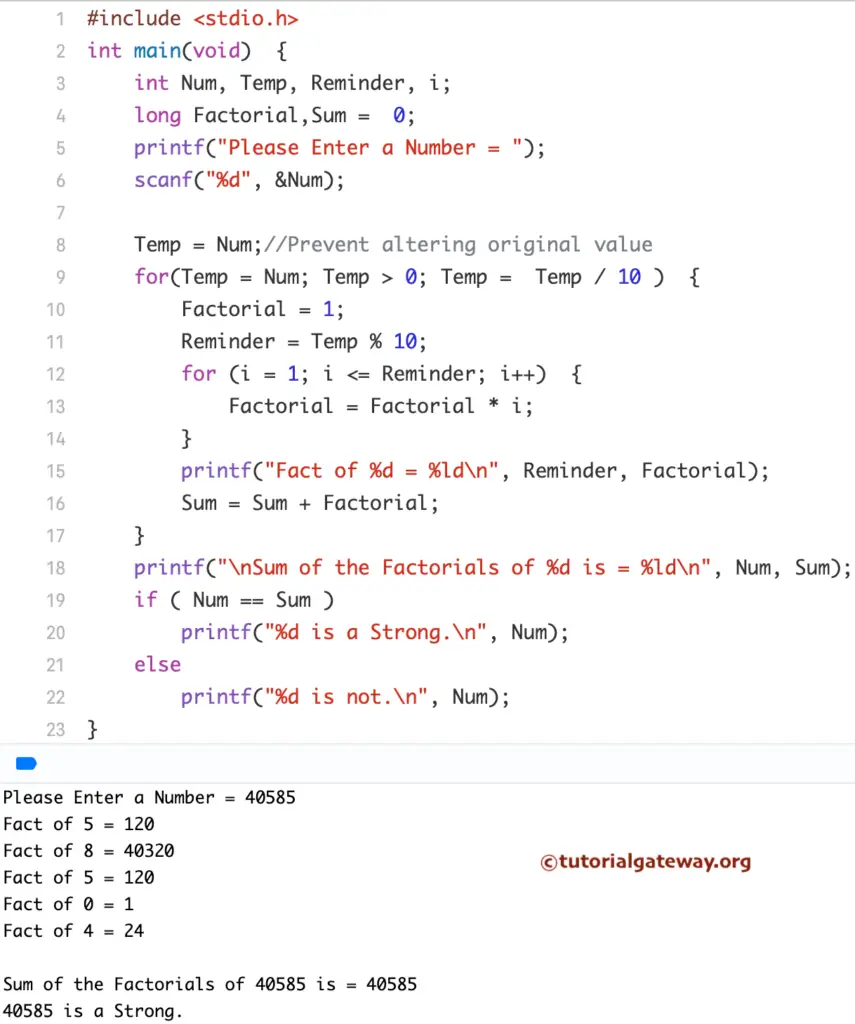
Let me check with another number.
Please Enter a Number to Check
26
Factorial of 6 = 720
Factorial of 2 = 2
Sum of the Factorials of 26 is = 722
26 is not.
We just replaced the While loop in the above strong number example with the For loop. If you don’t understand, then please refer to the article here: For Loop.
C Program to Check Strong Number Using Recursion
This program allows you to enter any positive integer. Then, this C program will check whether a number is a Strong Number or Not using the Recursion or recursive functions concept. Here, you can also try the normal function to calculate the Factorial.
#include <stdio.h> long Calculate_Factorial(int); int main() { int Number, Temp, Reminder, Sum = 0; long Factorial; printf("\nPlease Enter a Number to Check \n"); scanf("%d", &Number); //Helps to prevent altering the original value Temp = Number; for(Temp = Number; Temp > 0; Temp = Temp / 10 ) { Factorial = 1; Reminder = Temp % 10; // Calling Calculate_factorial Function Factorial = Calculate_Factorial(Reminder); printf("Factorial of %d = %d\n", Reminder, Factorial); Sum = Sum + Factorial; } printf("\nSum of the Factorials of %d is = %d\n", Number, Sum); if ( Number == Sum ) printf("\n%d is a Strong.\n", Number); else printf("\n%d is not.\n", Number); return 0; } long Calculate_Factorial(int Number) { if (Number == 0 || Number == 1) return 1; else return Number * Calculate_Factorial (Number -1); }
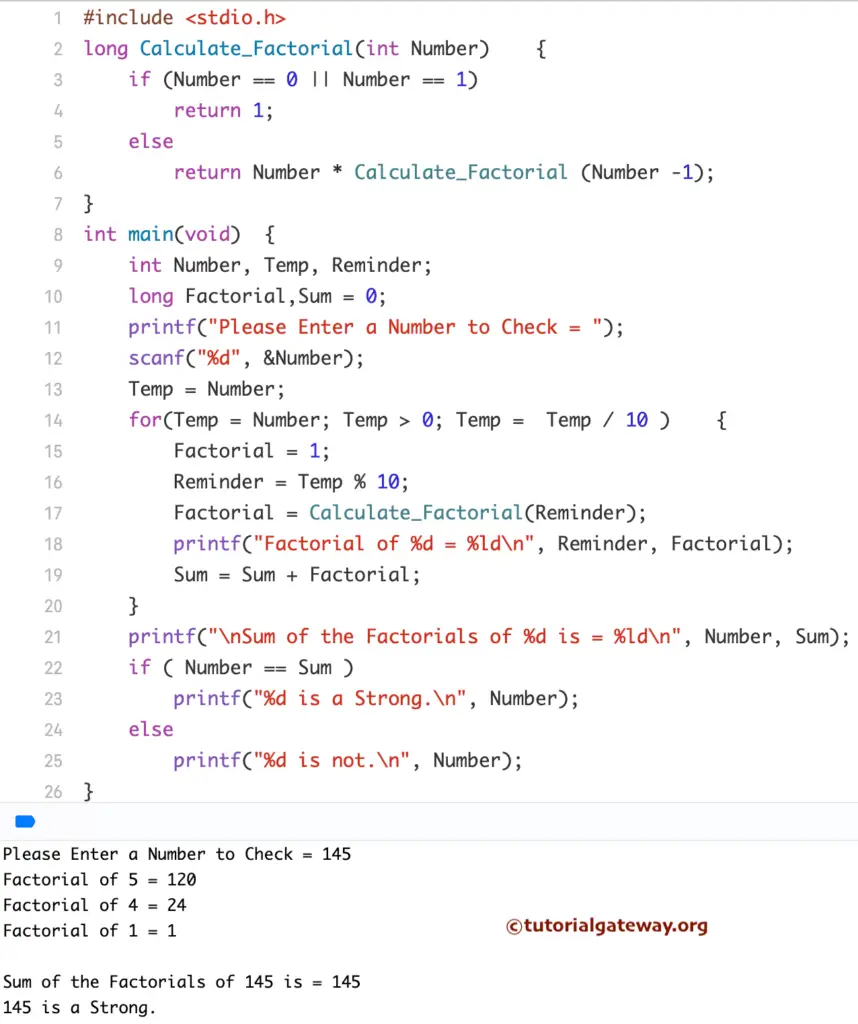
C Program to Find Strong Numbers between the 1 to n
This allows the user to enter the minimum and maximum values. This program will find C Strong Numbers between the Minimum and Maximum values.
#include <stdio.h> int SrNum(int); int main() { int Number, Temp, Sum = 0, Minimum, Maximum; printf("\nPlease Enter the Minimum & Maximum Values\n"); scanf("%d %d",&Minimum, &Maximum); for(Number = Minimum; Number <= Maximum; Number++) { Temp = Number; Sum = SrNum(Temp); if ( Number == Sum ) printf("\n%d is a Strong Number.\n", Number); } return 0; } long CalFact(int Num) { if (Num == 0 || Num == 1) return 1; else return Num * CalFact(Num -1); } int SrNum(int Num) { int Temp, Reminder, Sum = 0; long Factorial; Temp = Num; for(Temp = Num; Temp > 0; Temp = Temp / 10 ) { Factorial = 1; Reminder = Temp % 10; Factorial = CalFact(Reminder); Sum = Sum + Factorial; } return Sum; }
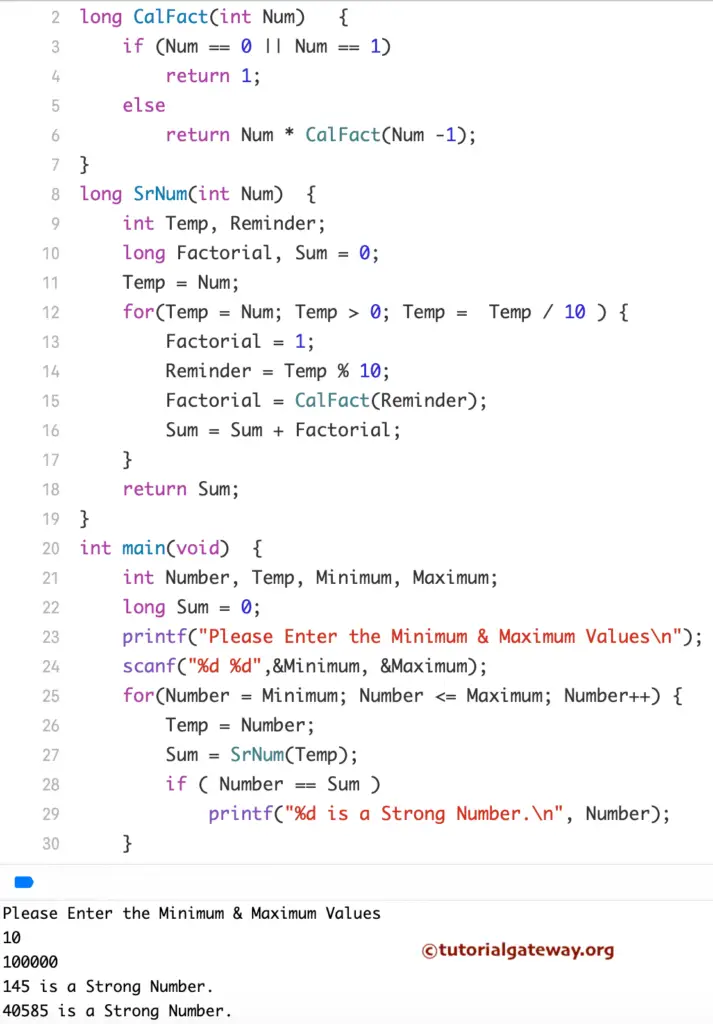
This program allows the user to enter minimum and maximum values. Next, the below shown For Loop helps the compiler to iterate between Minimum and Maximum Variables. Iteration starts at the Minimum. Then, it will not exceed the Maximum variable.