The numbers that are completely divisible by the given value (it means the remainder should be 0) called as factors of a given number in C. Let us see how to write a C Program to find Factors of a Number using FOR LOOP, WHILE LOOP, Pointers, and FUNCTIONS.
C Program to Find Factors of a Number Using For Loop
This program allows the user to enter any integer value. By using this value, this C program find Factors of a number using For Loop.
/* C Program to Find factors of a number using for loop */ #include <stdio.h> int main() { int i, Number; printf("\n Please Enter any number to Find Factors \n"); scanf("%d", &Number); printf("\n Factors of the Given Number are:\n"); for (i = 1; i <= Number; i++) { if(Number%i == 0) { printf(" %d ", i); } } return 0; }
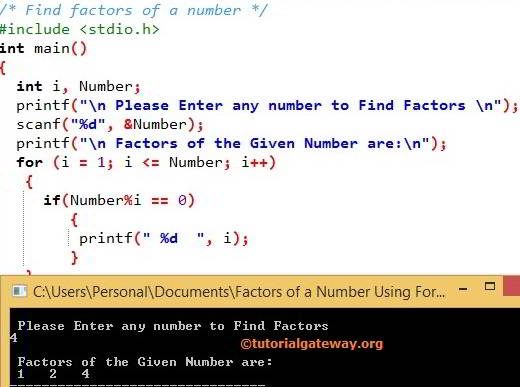
Within this program to find factors of a number in C, We declared two integer variables i and number. Next, we initialized the integer i value to 1, and also (i <= Number) condition will help the loop to terminate when the condition fails.
for (i = 1; i <= Number; i++) { if(Number%i == 0) { printf(" %d ", i); } }
Within the for loop, there is an If statement to check whether the Number divisible by i is exactly equal to 0 or not. If the condition is True, then it will print that i value else it will skip that particular value and check the condition for the next value. The user entered integer in the above C Programming example is 4
First Iteration
i = 1 and Number = 4 – It means (i <= Number) is True.
Now, Check the if condition – if (Number%i == 0)Number%i == 0 => (4%1 ==0) – This condition is TRUE. So, 1 is printed
i++ means i will become 2
Second Iteration of the c program to find the factorial of a number
i = 2, Number = 4 – It means (2 <= 4) is True
if(Number%i == 0) = if(4%2 ==0) – This condition is TRUE So, 2 is printed
Third Iteration
i = 3, Number = 4 – It means (3 <= 4) is True
if(Number%i == 0) = if(3 % 2 ==0) – This condition is FLASE So, 3 Will be Skipped
Fourth Iteration
i = 4, Number = 4 – It means (4 <= 4) is True
if (Number%i == 0) = if(4 % 4 ==0) – This condition is TRUE So, 4 is printed
i++ means i will become 5 – It means (5 <= 4) is False. So, For loop will be Terminated.
Factors of 4 = 1, 2, 4
C Program to Find Factors of a Number Using While Loop
This program finds Factors of a number using While Loop.
We just replaced the For loop in the above example with the While loop. If you don’t understand the While Loop, please refer While Loop article here: WHILE LOOP
/* C Program to Find Factors of a Number using a while loop */ #include <stdio.h> int main() { int Number, i = 1; printf("\n Please Enter number to Find Factors\n"); scanf("%d", &Number); printf("\n The Factors of a Number are:\n"); while (i <= Number) { if(Number%i == 0) { printf("%d ", i); } i++; } return 0; }
output
Please Enter number to Find Factors
125
The Factors of a Number are:
1 5 25 125
C Program to Find Factors of a Number Using Functions
This program to Find Factors of a Numbering C passes the user entered value to the Function. Within this User defined function program, we use For loop.
/* C Program to Find Factors of a Number using Functions */ #include <stdio.h> void Find_Factors(int); int main() { int Number; printf("\nPlease Enter number to Find Factors\n"); scanf("%d", &Number); printf("\nFactors of a Number are:\n"); Find_Factors(Number); return 0; } void Find_Factors(int Number) { int i; for (i = 1; i <= Number; i++) { if(Number%i == 0) { printf("%d ", i); } } }
Please Enter number to Find Factors
222
Factors of a Number are:
1 2 3 6 37 74 111 222
C Program to Find Factorial of a Number Using Pointers
This C program find Factors of a number using pointers. Please Refer to Pointers in C article before this example. It will help you to understand the Pointers and Pointer variable Concept.
/* C Program to Find Factors of a Number using Pointers */ #include <stdio.h> void Find_Factors(int *); int main() { int Number, *P; printf("\n Please Enter number to Find Factors\n"); scanf("%d", &Number); P = &Number; printf("\n Factors of a Number are:\n"); Find_Factors(P); return 0; } void Find_Factors(int *Number) { int i; for (i = 1; i <= *Number; i++) { if(*Number % i == 0) { printf("%d ", i); } } }
Please Enter number to Find Factors
1589
Factors of a Number are:
1 7 227 1589
In this C Program to Find Factors of a Number, We assigned the address of the Number variable to the address of the pointer variable.
P = &Number;
Here, P is the address of the pointer variable that we already declared (*P), and we all know &Number is the address of the Number.
Within the For Loop, We checked i value against the pointer variable *Number because *Number means the Value inside the pointer variable instead of the address.