Write a Sample Java Program to Print 1 to 100 without using For Loop, While and Do While Loop with example.
Sample Java Program to Print 1 to 100 without Loop
In the example, we are going to write a Java Program to return numbers from 1 to 100 without using Loops.
// Sample Java Program to Print 1 to 100 without Loop public class print1to100 { public static void main(String[] args) { int number = 1; printNumbers(number); } public static void printNumbers(int num) { if(num <= 100) { System.out.print(num +" "); printNumbers(num + 1); } } }
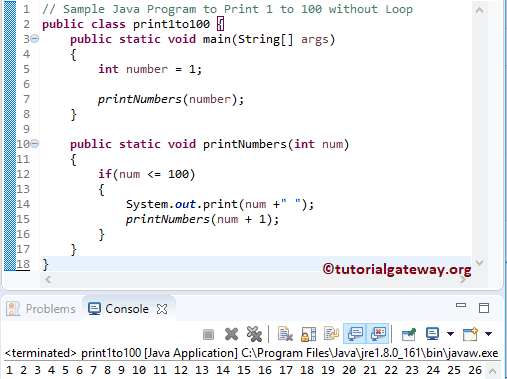
Within the method, we used the If Statement to check whether the number is less than or equal to 100 or not. If the condition returns TRUE, then the code inside the If statement executed.
if(num <= 100) { System.out.print(num +" "); printNumbers(num + 1); }
Within the If block, we used the print number(number + 1) statement, which helps to call the same method with the updated value. If you miss this Java statement, then after completing the first line, it terminates, and the output is 1.
Sample Java Program to return 100 to 1 without Loop
In the example, we are going to write a JavaProgram to display numbers from 100 to 1 without using Loops.
// Sample Java Program to Print 100 to 1 without Loop public class print1to100Ex2 { public static void main(String[] args) { printNumbers(100); } public static void printNumbers(int num) { if(num > 0) { System.out.print(num +" "); printNumbers(num - 1); } } }
100 99 98 97 96 95 94 93 92 91 90 89 88 87 86 85 84 83 82 81 80 79 78 77 76 75 74 73 72 71 70 69 68 67 66 65 64 63 62 61 60 59 58 57 56 55 54 53 52 51 50 49 48 47 46 45 44 43 42 41 40 39 38 37 36 35 34 33 32 31 30 29 28 27 26 25 24 23 22 21 20 19 18 17 16 15 14 13 12 11 10 9 8 7 6 5 4 3 2 1